Keeping Your Azure SQL Databases Healthy: The Power of Automation
In the realm of database management, maintaining optimal performance and storage efficiency for your Azure SQL Elastic pool and databases is critical. SQL databases are the backbone of countless business applications, supporting everything from transaction processing to analytics. However, as they grow over time, they can face challenges like bloated storage, performance issues, and cost inefficiencies. That’s where our automated maintenance script comes in.
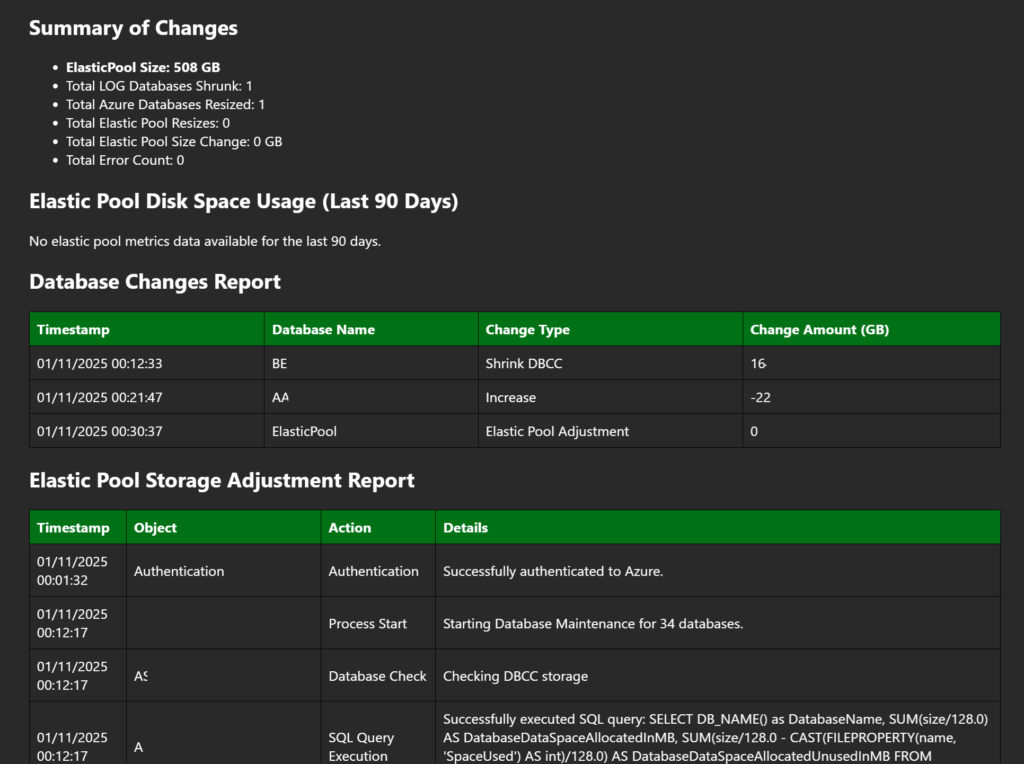
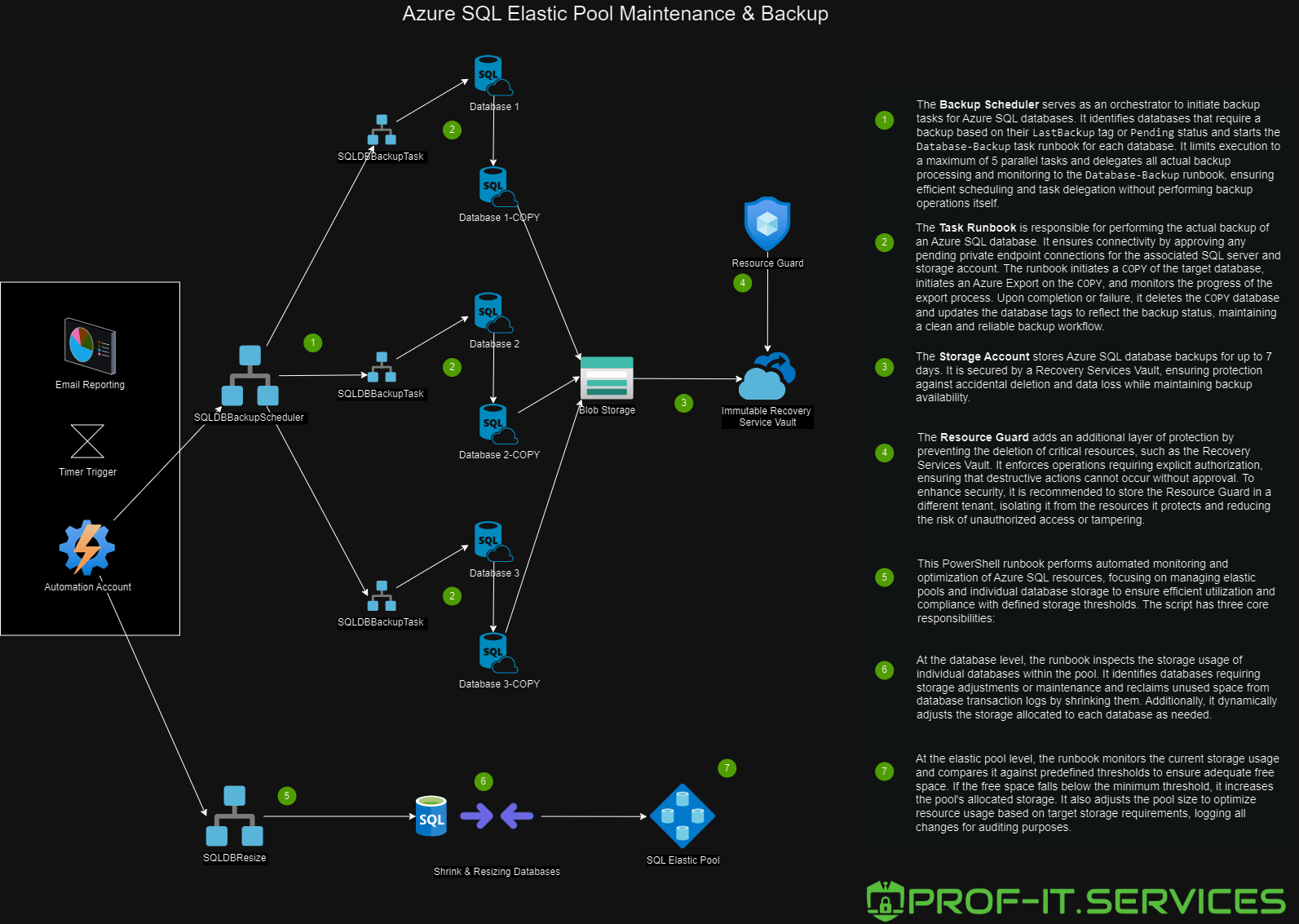
This blog will explore what this script does, why it is crucial for good database health, and why every database administrator should consider such automated solutions. Our future blog will be about Azure Azure SQL Backup leveraging the same Azure Automation Account.
EDIT: Azure SQL Backup leveraging automation account is not feasible anymore due to slow backup performance.
What Does This Script Do?
This maintenance script is designed to be executed on Azure Automation Accounts, which can use either Azure workers or hybrid workers. If the environment lacks Virtual Network (VNet) integration, the script automatically adds a temporary firewall rule to allow access. This flexibility makes it easy to run in various configurations, ensuring secure and seamless database maintenance.
The maintenance script is designed to automate the labor-intensive process of optimizing Azure SQL database storage and ensuring elastic pools have enough space to accommodate dynamic workloads. It performs several key functions:
- Elastic Pool Management: The script assesses the current utilization of your Azure SQL Elastic Pool and makes necessary adjustments. It ensures the pool has adequate free space for future growth while avoiding unnecessary costs. If the available space is low, it will resize the pool to add additional storage, keeping your databases efficient.
- Database Shrinking and Resizing: The script checks each database’s used space and compares it to its allocated storage. If the unused log space exceeds 20% of the allocated storage, the script uses direct SQL queries to perform a shrink operation to recover wasted space. If a database requires more space based on its usage, the script also resizes it accordingly, aiming to keep 50% more space than currently used.
- Firewall Adjustments: The script adds a temporary firewall rule for the executing machine’s IP address to ensure that it can interact securely with the SQL server. Once the operations are complete, the rule is removed, maintaining a secure environment.
- Reporting: After performing these maintenance tasks, the script compiles a comprehensive report detailing the changes made, including database shrinks, resizes, and elastic pool adjustments. This report is then sent via email to the designated recipients, providing a complete audit trail of all actions taken.
Why Is This Important for Database Health?
Managing storage and ensuring that databases are operating efficiently is essential for several reasons:
- Performance: Over-allocated databases with significant unused space can slow down performance. By automatically resizing databases and ensuring they have just the right amount of space, the script helps maintain optimal performance levels.
- Cost Efficiency: Cloud storage can be costly if not managed properly. Resizing elastic pools and databases based on actual needs ensures you are not overpaying for unused capacity. Conversely, under-provisioning can lead to service disruptions. This script helps balance both aspects, providing cost-effective storage without risking performance issues.
- Scalability: As your application usage grows, your storage requirements will change. Automating this resizing process allows your databases to scale dynamically without requiring manual intervention. It’s about keeping pace with growth seamlessly.
- Security: By automatically adding and removing firewall rules, the script ensures that database interactions remain secure, reducing the risk of unauthorized access.
A Note on Backups
While this maintenance script focuses on optimizing database performance and storage, it’s also important to ensure your databases are backed up properly. A separate upcoming blog will focus on automating Azure SQL backups to Azure Storage Accounts, providing a robust and reliable backup solution for disaster recovery and compliance purposes. Stay tuned for more details!
Conclusion
Database maintenance is a time-consuming yet necessary task. Automating processes like storage adjustments, database resizing, and firewall configuration ensures that your Azure SQL databases stay lean, cost-efficient, and high-performing without requiring manual oversight. This script is a powerful tool that helps database administrators achieve these goals, allowing them to focus more on strategic tasks rather than routine maintenance.
<#
.SYNOPSIS
Requires PowerShell 7.2.
A script to automate Azure SQL maintenance tasks using Azure Automation.
.DESCRIPTION
This script automates the maintenance of Azure SQL databases. It is designed to:
- Adjust Azure SQL Elastic Pool storage based on usage.
- Shrink log files if they exceed 20% of allocated storage using direct SQL queries.
- Resize databases dynamically based on usage requirements.
- Manage firewall rules for Azure worker access when Virtual Network (VNet) integration is unavailable.
- Send a detailed report via email of all maintenance actions taken.
The script can be executed in Azure Automation Accounts, supporting both Azure workers and hybrid workers.
.NOTES
Author: Prof-IT Services
Contact: [email protected]
Date: 2024.12.02
Version: 1.7
#>
# Import required modules
Import-Module Az.Sql
Import-Module Az.Resources
Import-Module Az.Monitor
# Define variables
$resourceGroupName = "" # Replace with your resource group name
$serverName = "" # Replace with your Azure SQL Server name
$minimumDBsize = 10
$minFreeSpaceGB = 10 # Define the minimum free space in GB
$maxFreeSpaceGB = 20 # Define the maximum free space in GB
$targetFreeSpaceGB = 15 # Define the target free space in GB after adjustments
$elasticpoolbuffer = 40
# Check and adjust elastic pool storage before resizing any database
$elasticPoolName = "" # Replace with your elastic pool name
# Define parameters
$poolName = ""
$userName = ""
$password = ""
# Define connection details
$serverFqdn = "$serverName.database.windows.net"
$connectionString = "Server=$serverFqdn; Database=master; User ID=$userName; Password=$password; Encrypt=True; TrustServerCertificate=False;"
# Define ArrayList to store logs
$logEntries = [System.Collections.ArrayList]@()
$databaseChanges = [System.Collections.ArrayList]@()
# Counters for actions
$shrinkCount = 0
$resizeCount = 0
$elasticPoolResizeCount = 0
$elasticPoolTotalChangeGB = 0
$elasticPoolTotalSize = 0
$errorcount = 0
# Function to log actions and statistics
function Log-Action {
param (
[string]$Object,
[string]$Action,
[string]$Details
)
$logEntry = [PSCustomObject]@{
Timestamp = Get-Date
Object = $Object
Action = $Action
Details = $Details
}
[void]$logEntries.Add($logEntry)
Write-Output $logEntry
}
# Function to log database changes
function Log-DatabaseChange {
param (
[string]$DatabaseName,
[string]$ChangeType,
[int]$ChangeAmountGB
)
$changeEntry = [PSCustomObject]@{
Timestamp = Get-Date
DatabaseName = $DatabaseName
ChangeType = $ChangeType
ChangeAmountGB = $ChangeAmountGB
}
[void]$databaseChanges.Add($changeEntry)
Write-Output $changeEntry
}
# Function to send report via SMTP
function Send-Report {
param (
[string]$SmtpServer,
[string]$From,
[string]$To,
[string]$Subject,
[string]$SmtpUsername,
[string]$SmtpPassword
)
foreach ($log in $logEntries) {
if ($log.Details -match 'failed|error') {
$errorcount++
}
}
if ($errorcount -ge 1) {
$rowStyle = 'style="background-color: red;""'
}
# Create summary of changes
$summary = @"
<h2>Summary of Changes</h2>
<ul>
<li><b>ElasticPool Size: $elasticPoolTotalSize GB<b></li>
<li>Total LOG Databases Shrunk: $shrinkCount</li>
<li>Total Azure Databases Resized: $resizeCount</li>
<li>Total Elastic Pool Resizes: $elasticPoolResizeCount</li>
<li>Total Elastic Pool Size Change: $elasticPoolTotalChangeGB GB</li>
<li $rowStyle>Total Error Count: $errorcount</li>
</ul>
"@
# Convert database changes to HTML table
$databaseChangesContent = @"
<h2>Database Changes Report</h2>
<table>
<tr>
<th>Timestamp</th>
<th>Database Name</th>
<th>Change Type</th>
<th>Change Amount (GB)</th>
</tr>
"@
foreach ($change in $databaseChanges) {
$databaseChangesContent += "<tr><td>$($change.Timestamp)</td><td>$($change.DatabaseName)</td><td>$($change.ChangeType)</td><td>$($change.ChangeAmountGB)</td></tr>"
}
$databaseChangesContent += @"
</table>
"@
# Convert log entries to HTML table
$logEntriesContent = @"
<h2>Elastic Pool Storage Adjustment Report</h2>
<table>
<tr>
<th>Timestamp</th>
<th>Object</th>
<th>Action</th>
<th>Details</th>
</tr>
"@
foreach ($log in $logEntries) {
$rowStyle = if ($log.Details -match 'failed|error') { 'style="background-color: red;"' } else { '' }
$logEntriesContent += "<tr $rowStyle><td>$($log.Timestamp)</td><td>$($log.Object)</td><td>$($log.Action)</td><td>$($log.Details)</td></tr>"
}
$logEntriesContent += @"
</table>
<br><br>
<div style='text-align: center;'>
<img src='data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAB9AAAADMCAYAAAArizgXAAAAAXNSR0IArs4c6QAAIABJREFUeF7snQl8VcXZ/3/PnHPvzULYcUHFpVoBl6qBBFAkC4totdY2JODyV19frW21re/bvdXY9X1t+3bxbd/WttaKkoS0tlYFFUiCC5AAtbVuqKXuooAIgSz3njPPnzk3wQAJucu5S5JnPp9a4M488zzfOWfOzDwzzxCGYLq48YyRORwoBKkvK1d/asns9ZsHE4YLHyjMyxtmP0nMd6NT/Wli87rXqquhB5ONYosQEAJCQAgIASEgBISAEBACQkAICAEhIASEgBAQAkJACAgBISAEhIAQEAJCwG8C5LfAbJZXsRSWGl1cQcQ3gnAWQCEANbVlzYuyWe94dVvQOG22Yl4GZguKXmWN+7R2vl0/Z+POeGVJfiEgBISAEBACQkAICAEhIASEgBAQAkJACAgBISAEhIAQEAJCQAgIASEgBITAUCEwJBzoFQ9PH23bKGJL3wzG9J6NS8D7pHXhYDqFvnBV8U+Y8Ln97CS0Mui7ynXrP1x+wSvVVC0n0ofKWy52CgEhIASEgBAQAkJACAgBISAEhIAQEAJCQAgIASEgBISAEBACQkAICAEhEBOBQe1Av3ZDYWDXzsClAD4N4o8ACPZCxQVwbW1Z850xEesnU3Vjif3P8HuhxfOe3hOrvEUPnjZqyUf/sSPW/IfKV7G0wrLGvr4Z4Am95NNgfgNQfyEVubWmdOM2P+oUGUJACAgBISAEhIAQEAJCQAgIASEgBISAEBACQkAICAEhIASEgBAQAkJACAiBwUBgUDrQFzYWjmUOzADzLdFQ7YdOBDxSU9Z8Xn/5+vu9mqvV8w0P/5SIp0LxF5UVfGbJzCd6dYwb5/7OPYETlcP/poH5eruaUr9gbXt/dfT3e9Wq4stBuLu/fAy0K6j/IkvXRM6dsLme6s1GAklCQAgIASEgBISAEBACQkAICAEhIASEgBAQAkJACAgBISAEhIAQEAJCQAgIgSFLYFA50G94aX7o3Ve3X0kWrmOm0wiwY2tZ6mStT6+b3fJibPl7z7WgYcrZClYTovV2ANgEoJmI17CjX3VhOcrCGGI6k4hLGfgwgCMAMDH+p6a8+T+Tqf/CBwrz8vPt5wAcG6McBvAmGMvh2tW1c598K8Zykk0ICAEhIASEgBAQAkJACAgBISAEhIAQEAJCQAgIASEgBISAEBACQkAICAEhMOgIDAYHOl3+yIxxkaB7LjPfQqBTE2ylmprS5kuJYJzKcado6PTX7gVQGXdhU8DUq9zC2lkbnkqoPIAFK6fOVkotAxBIQEYYoB8S0eK3EXq5qbTJSUCGFBECQkAICAEhIASEgBAQAkJACAgBISAEhIAQEAJCQAgIASEgBISAEBACQkAIDFgCA9qBfu2Gwrxdu9Q1zOoaIkwGYCXcEkQ73E4qqj9v7cuJyFi0akqRJu/0eW4i5U0ZZvyurrz56kTLV64q/jERPp9oeaMCwFsA9ahidcuS8jWvJiFLigoBISAEhIAQEAJCQAgIASEgBISAEBACQkAICAEhIASEgBAQAkJACAgBISAEBhSBgedAZ1BF09TDlYtyUupmRMOg+5FcReq6JaVrfxuvsEuXFQ93Q9gAwknxlj0g/55IxJnyx3kbX4hXzvxlJ4ZG5I55Hozj4y3bR36XwD/VpH83ooA33TFlY8QnuSJGCAgBISAEhIAQEAJCQAgIASEgBISAEBACQkAICAEhIASEgBAQAkJACAgBIZCVBAaUA/2iJ84uyA+71zPzlSCcDED5SZWAR2rKms+LV2ZVw9QbAfXTeMv1np9ecHPorPoZa9vjkVfZUHQFgX4fT5mY8jLeBWEVa65O9o74mOqTTEJACAgBISAEhIAQEAJCQAgIASEgBISAEBACQkAICAEhIASEgBAQAkJACAiBDBHIfge6OXHeMH28DT2XCebE+XEpZBVRAT1xycz1m2Ot49J1xcPdNjwO4PRYy/SXj5n/va685Tf95ev+/cIHCvPyh9kvgHFMrGUSyKcJ+KUG37GrY8wLy89f3pmADCkiBISAEBACQkAICAEhIASEgBAQAkJACAgBISAEhIAQEAJCQAgIASEgBISAEMhaAlntQF/04GmjnNzcGxXRIsALj55yfQm8tKaspTLWFlvQUPxTBdzgr2787JbH8s5oqm5yYtFj4criOaywDIAdS/4k82xjYLV21a31c9b+I0lZUlwICAEhIASEgBAQAkJACAgBISAEhIAQEAJCQAgIASEgBISAEBACQkAICAEhkDUEUu6QjtfS6mqoF0qnHgWHLgDRNwAcFa+MJPPvUgF9Ziyn0D/xSOHEQMA2TmS/HddMzJ+rKW+5PRZbqhqKTfj4G2PJ61seAoPxO7bp57luznN3lTZ1+CZbBAkBISAEhIAQEAJCQAgIASEgBISAEBACQkAICAEhIASEgBAQAkJACAgBISAEMkAgqxzoCxsLxzLbNwG0AOAPZYCHqdJVpK5bUrr2t/3Vf2VjSU47t19BgHFg5/SXP47fHwqErKsXn73m3f7KVCytsKyxr28GeEJ/eVP0+3sAnmCtv1M3e/36FNUhYoWAEBACQkAICAEhIASEgBAQAkJACAgBISAEhIAQEAJCQAgIASEgBISAEBACKSeQcQe6d+J81rQJrPliAr4CwuEpt7r/Ch6tLWue1382AAxa0FB0nSL6AYBhMZXpO5PD4FWK3MtqSjdui0VWVVPx5dC4O5a8Kc/DqFEB9ZPWneFnHrhwY1vK65MKhIAQEAJCQAgIASEgBISAEBACQkAICAEhIASEgBAQAkJACAgBISAEhIAQEAI+EsioA/2yx2Ye6Tqd/8mgSwAc56NdSYqiTmXxaUtmNb8UiyBzCtwe8+YsJncJkMQGAKb/dXPpS/Uz1rbHUu+FDxTm5Q+zXwDjmFjypynPToDXWtDfubdsw5NpqlOqEQJCQAgIASEgBISAEBACQkAICAEhIASEgBAQAkJACAgBISAEhIAQEAJCQAgkTSDtDvRqrlbPNzx6PCnnk9D0RRDGJG1FCgQQo7amrHkRzF3fMaYFDUXzFOhOAONjLNKdrQ1Md740InLTxikbI7GWXdhUPIddLAfBirVMOvMx858J1g8Czp6/L5739J501i11CQEhIASEgBAQAkJACAgBISAEhIAQEAJCQAgIASEgBISAEBACQkAICAEhIATiJZBWB3rV6qnHsGt9lcAfBbLq1PTB3Bg7XaWm1JeufTkeqJWrp00il2sBnB5TOQIr4AtLSpp/Fo+z3siubCj6GYFuiKmezGXaDXAzwN+vLVu/KnNqSM1CQAgIASEgBISAEBACQkAICAEhIASEgBAQAkJACAgBISAEhIAQEAJCQAgIgUMTSLkD3YQ3V6Nf/RAUVQH4AgEjB0ijuAx8uq6s+Y549a1ccdYkpQJ1TDgVwKEYb9PAl5eWNZtT63Gl+ctODI3IGfNCdoW+P7QJrPGIVur7na3qr3/52JOtcRksmYWAEBACQkAICAEhIASEgBAQAkJACAgBISAEhIAQEAJCQAgIASEgBISAEBACKSaQUgf6JSunnhBS6hsMzEsgrHmKTY9BPGNFbXnz3BhyHpRl4criwxn8Wyi6oLfyzNypwBU15esfSER+VVPx5dC4O5GyGS7TzsB67fJt9bNblsV76j7Dukv1QkAICAEhIASEgBAQAkJACAgBISAEhIAQEAJCQAgIASEgBISAEBACQkAIDGICvjvQSxpL7MNVx0nk6suI6AZmFAxgfhEV0BOXzFy/OREbKh6ePtoK6noAZfuXp7dUgC9aMrN5YyJyL3ygMC8/394E4OhEymdRmSZN9N1ALrfcO615VxbpJaoIASEgBISAEBACQkAICAEhIASEgBAQAkJACAgBISAEhIAQEAJCQAgIASEwBAn46kCvaDj7ZAvOLV0O48MHA09irq8pb1mQqC2VDUVXEOj3B5Rvqi1rLk1Y5qriuURYBsBKVEYWlesA+K/k0v84O5r/XL8AbhbpJqoIASEgBISAEBACQkAICAEhIASEgBAQAkJACAgBISAEhIAQEAJCQAgIASEwhAgk7UC/dkNhYM+O4ERtuVcy6FMA8gYZv93M1hl15Wv+Ga9d1dVQm2YWPcFE0/crS7QjBznj7ypt6ohXpslfuWra7UT82UTKZnUZxloGfTtXday9q/Rv72e1rqKcEBACQkAICAEhIASEgBAQAkJACAgBISAEhIAQEAJCQAgIASEgBISAEBACg45A4g50BlWsLDxVWYFqAp8LYOygo2MMYtZMdH1dWfMd8dpXsbroeMsl43g/iDO7+uq6Oet/F7fMpRWWNfb1zQBPiLfsAMkfBvA3gH86fLhbf8eUjZEBoreoKQSEgBAQAkJACAgBISAEhIAQEAJCQAgIASEgBISAEBACQkAICAEhIASEwAAnELcDvWLp9Fw1hieB+N8IuBpAzgBn0L/6jBW15c1z+8+4f44+wrd3Z/rrxMeap1ZXQ8cjtx+Z8YgaCHn/ysC3tB1eUz/zqW0g8EBQWnQUAkJACAgBISAEhIAQEAJCQAgIASEgBISAEBACQkAICAEhIASEgBAQAkJgYBKI2YFuQrXv3GWfT8B/AjgFwKiBaXIiWnOncui0JXObX4q1tAnf/vzMol8R0TV9lHGVhUlLZsUus2LN9FyrnV8G8fhY9RgE+RyAXwCrP5LK+d+a0qZtg8AmMUEICAEhIASEgBAQAkJACAgBISAEhIAQEAJCQAgIASEgBISAEBACQkAICIEsJNCvA33+shNDI3LHTmfmrxNQ3ls48iy0KwUq8dLa0paqWE9BVyydHLTGFrQA+EgfyjCDr6wra7k7VmUrVxXPJWA5CCrWMoMqH/M7xPQt27X+sHjemncHlW1ijBAQAkJACAgBISAEhIAQEAJCQAgIASEgBISAEBACQkAICAEhIASEgBAQAhkncEgH+hUri8aEFd0O4EIAwzKubWYV2GWTKryndO3LsahRubLow1D0LAF2n/mJfllbuu76WOSZPFWN024H82djzT9I85kw7k9B8edrS1oeH6Q2illCQAgIASEgBISAEBACQkAICAEhIASEgBAQAkJACAgBISAEhIAQEAJCQAhkgECfDvTKVdPOIvA9IEzKgF7ZVyVDk+JP15S2/CoW5SpXFt1Gir54qLwMPFdX1mzC4febKp6dHLTeKdgE4Lh+Mw+FDMyagc8d1vner28//+XOoWCy2CgEhIAQEAJCQAgIASEgBISAEBACQkAICAEhIASEgBAQAkJACAgBISAEhEBqCfTqQF+wsrhUKZjQ4kentvqBJZ1BK+vK1s3pT+uKpdNz1Vj9NgEj+snrKAuTY7kHvbKh6AoC/b6/uofY7+3Q+Ent7OavAzAn0yUJASEgBISAEBACQkAICAEhIASEgBAQAkJACAgBISAEhIAQEAJCQAgIASEgBBImcJADfdGjxSdpCw+DcELCUgdrQUJEuYGJS2Y/sflQJi5sLD6HGbGFF48hjPuFDxTm5efZL4EwfrCiTc4uvsndduzP6hfUu8nJkdJCQAgIASEgBISAEBACQkAICAEhIASEgBAQAkJACAgBISAEhIAQEAJCQAgMZQL7O9AZtLCh+D5W+BgYh7wffahCY+CPdWXNnzyU/VUNxd8E8K2YGDF27RnhHPnAlI1tfeVf0FA0T4GW7ZWpYpI59DJtYc2z6ma3vDj0TBeLhYAQEAJCQAgIASEgBISAEBACQkAICAEhIASEgBAQAkJACAgBISAEhIAQ8IvAfk7yqoaiCwB60C/hg1EOM9qQY51ed/aaf/ZmX0ljiX0EOv4E5o/GaD+DqKy2dF1TX/mrGqb+L6A+E6O8oZmNsbi2vPmKoWm8WC0EhIAQEAJCQAgIASEgBISAEBACQkAICAEhIASEgBAQAkJACAgBISAEhIAfBPY50D3HL7f9GaAL/BA8iGVoMH2mtnzdL3uz8doNhXm7Wq1XwDQuVgbM+GpdefN/9Za/YmmFZY19dTNAE2KVN0TzdSBof6j2nCffGqL2i9lCQAgIASEgBISAEBACQkAICAEhIASEgBAQAkJACAgBISAEhIAQEAJCQAgkSWCfA33hyuLD2UIjGJOSlDnoizOwsq6seU5vhi5cWXQxK/pTPBAYWFZX1tzrxoXKhqIrCPT7eOQN2bxEV9WWrrtryNovhgsBISAEhIAQEAJCQAgIASEgBISAEBACQkAICAEhIASEgBAQAkJACAgBIZAUgX0O9MpVMz5E5Jow4kcnJXFIFKZOZfFpS2Y1v3SguQsbilczcG6cGN5zt004rH5BvduzXMWa6blWh2tOnx8Rp7yhmZ3o1trSddVD03ixWggIASEgBISAEBACQkAICAEhIASEgBAQAkJACAgBISAEhIAQEAJCQAgIgWQJ7HOgVzROP9Fm3cjiQI+JKRHX15S0VILA3QWqHpl6DALqtZgEHJiJqKq2dF1dz39euHLqeazUMgD73VWfkPyhUejbtWXNNw8NU8VKISAEhED8BK79VWEg/OECa4/TGrJY57uBnDyFSI7FViiiOWhZKqQAm10mVlYw/hriLeGAFIWJyfuWavP/SoWV29npkurU0B1WhNpygpHWPaHczq2doUhTaZPZbLbv2xtvjZJfCAgBISAEhIAQEAJCQAgIASEgBISAEADM1aH547YG9gC2jdYcB8q2XcUUCoRb32/vbB1WEG4qbXKElRAQAkJACAiBoUhgn2P2kocLjwyG7AYwJg5FEAnYvJs1F9bNbnmxu2xl47RLifmeBGQZH3lLbdm64p5lKxuKf07ApxOTNwRLMV1bW77u11ljOcPzCN16a3X/GyBu6V3rZ+uf7b9s1hgMnFJxygdOrVsPrdgtt1Tv5wDzDO2xISWLzEpMFQZV1FeorRVb6bgm2Dvdtnw7FMiHo4MhVpaLsH2gYE1MFsjWDh/U7kxBm1yt+lLGVRyw6OByfeVXxKRdO3DQ78QuSO8XDSNWAFopo6CDSFeJoOXC6dwnywoGHO06ulue1hQJ5FnRv3cCbZq8krn5rqvfQUQF0B4+trVj6+TD9Lj6cby0YqkmijpaJcVEgCqWVqjISVsLgu/tOZ0UTYKmiUQ4gYGjFHgYg/IB5AAwznLzPOx79phjf55i0qaPTAe1qekHGOa5CANoB9AG5lYovMmMFwn0rM30t7y2yAt3bNzYgWov75BN1Vyt0NSktre/YW3LHVHg2Dl5Ae2EKIKAVjq5bwjZrhVCn4slkfYwRxDq9fegE9E5wT3dvcF+7bPHvOfusAh2xd9sVk5EcW6ndXDJfOyxg0E3zGQeaKU4gBAQiXBAua5Slq3ccMT2nvQOO+haWlmADW0FSTkBZZHNrC2oQNBlJ6hg/k4WEQegjQTugMNbbWW/8JYKvimLSPG33UApUc1QD9xRaBUWfqDxi60FfX57xm0dx/UV9dqXMYwZO6ydnhNs08drS03Q0CPhasuy7bAb0WEotDN0B1mBdpu4w/xZByliETsaeyKBt/IcdzxFwu2tend7ODJsdzDub+YRw8K0JTf4wfigc0Qwt80lDAfard0B89EwKYICu11b+97FoNJkh2i/sU33+6ctS5NGe1irg/QZBnaUclzA9AwfJDdsa7Jy3O5+oj3P4nGhneGtPfLk4zBnz9Z3E/oG5JxsBzrecwgFuVbua5bNh3dYVthRu1BgW4oDSiGgIoGAZjfkjc3YDREhoAKqUzvu+7njg6+1Pj2+7cAIYgPlOR8kehJzj7nWLUD33Gnrc1sJs/a3srWg1fsmdr/aOza3J/eNjAPiqBNy434X+9Jv1Am59o7WdgUn18pttbx3zhkX1EHHbj9u7zi8uqTJ9aU/isO+eLMaZ82oEzYrpyMnp1W35eS7vX3Xe5faiVwnsMOKm6fpQxDaacaWvqSCgEutu0cHvP6xtzQc4Nxov9JXhY6VS52UEzBjF5PHG7/k8r7+t7NNB+2usZzph5yuuSAT2eRaUbk5ZCHiRvtiS1mIUPTP5slwgIjNuwNO5+tb5ox4t4kyvwm1urpaNc1qUigBxtW/G7VhHIIBe3TA3ho+iJUatfvg+aoZygVGqXDnB6x8adQ+hFjBTg4xIrbbvu+56zST7115+42B2ye4Dlrb9819c0bbvO3NNqe3b3HPPmFjV70F/Yw1eqpn1l1uQTVTJtdPGFR9azU9O/lZ2jpuK7W+2Epm7LRjc9DGsI5hbig3zwqFc2xthbiz6xkF4CLgWDa35gWs7RPODrTj1hJdXV2d0Lc8le0+2GWbd/Gfcx4dG+nU00A4i5lPJcJ4MBcAZJvvCDM6ibGVgafZotWBHF5977TmBGZwB9M09T8766HDoGmyTTxWmzGWozpgqz3k6k5HUVi5TsClQI5SnKehc0znpplbLUe/VDAG/7xjysZe55oDvu28d2tvC9xS/cG4ZtzWfd+anuOZWMYyB45B9vU5hQUME4e4nzSu3R2lctsPV12HHkz2nmt5EQ5zpCO6JhAd1+90u/vIQJ7FHVo74dzWQ7/jnRTMbcsnNYoDe5xgMJATtl1thQKE3LCDXEtznmY3z8w8XK1cS9HbkW25m+ormvZkaszDDFqACrV542Z1Qjho21v1cB5OuezqoLcW0ZX2rUP04BwMAo4TCPS29ttfe+yTaxNrOxLxVs3iTETWW47Vuv/kq4eMnLDN29zev19xVnXo7KcAo9oPHtdGxrfZoXZNe/QIC0FlWQQnsKd15+vB0Z1NA2CcGycjqq4267DVQI+5jJFhvq09ZR04lzG/HbIPOHqEhc6dvaydxanhAdkDe0YH7PD+Y7b2nIjKz+20use2AcWkwh0BhEKI7OEAbMeKzqmtUHSNjYNEVsAlN0AaASiymBEhop2uHdl8ilPwVnUWbdza1xDzl80PjcjZ3gDQjOQwDpnSmoEb6sqaf2EsNh3nwsbiXwK4NkECDKITakvXvWLKVzw7OWhtKXgRhGMTlDfkitmwJ95T9uSmNBtO124otFvft0dD6bEuWUcqYAwzj/D+HygAOIeYFJNxqHOIcKBTypsn2qS9ae5+iZXpRDlknq9D2kWkQJyG06KAOSmqmcNGsZ46ERAhpn2TSLbRCW1eE5PReHbJDL6jfyfSYL3vM6+ZvEVhYm4lqN1g3k02djoa77la7whZepeztX3XKc8951RnsbOsYunkYM6Ro45wOp2TtaVOJq2LmDCZgGMAhBgwjU3kOSZ7b9K964C9/kDm+elrqSgqjeJZBfSewl4cpKYK8zAl+h5p09zdpb06PnhOvAl+D8neX3o6xPcNyL0Hnsk8aqB2Zt5CRK8x8CIxvaRtftMivGHB2Tpq92Hv3d6yPDLUnahd7UXzl50YHBYaN9Zi9wQmdSoRnwfw2QDlMxDq88FLtMEzUs50Qegg0BYmeoiYGm1Sz1Gk9Y3Fc59uy9QEKl0ozKIzxr0yznLpJPb6GS6CwmnQOB6EHGbzCh+6n4lV1+gr2U9/0GMivZ9cMj1/n73W/u9+rAp15+tjcwdDRbtB77/Rb213f3tA32r6SzL/YZOPzTc0+rUCSEX7Ru9r7eXr6slMx+SA0cGEdaz5/0Jux4rF857uc+IZr1mSP3MEqquhXpx55jEuh84hxWUMnA7CSGJWBBXW4D3E6ED3fh0iCwwLpMwGn9cY3EIqsG5n+/B/LD9/eWe8lphxXlXDjBNIuR9n5qsI3vg/6NXhPcjmPWQN730zLxaZRSjzTGpvZAbtPcreYqf3AEff2w8+x6as6lMvhjfiDO17fbxKu94a8370+u6Zj/p+Q4/uavdlN91AVH/zxVe699ghJlMvfUUvG+bY7Ozaz1nQv+fAVG2AHNgmXknTB7DXFUR/7373TWyUKELzX/ObgWBEmaymDYyDZBeYHtGK7uWt9GT9grVmw5ckHwmY9/KtjxZaHe9bwzmA4a5Lo2GrsdrlkdDucCIa5UKNIOI8BeSy9sY5ORqco4iCmpFL5j0i4/vy2jFkHkQib85lA8oGHzj/4hCTN16POZHZ8Md0yM2n5g0g4o5YhUZHyBTqOS1gYuNf7XrpukbRXX8174U2YyPCS2CsYMIKHVJ/r5+RHc9lSWOJfRTaj3BZn6xJnaGAadAwO68PJ4LV25ykT1ZJbKaNdgf+RDEyHbFJ/bZpPxtSo/1oDzE9NkT3nDP2HMd0dUTR7rVrPGP+rLs6tK4uvGtkQ2Y3ZRiEp0H0W2Xb9y+Z+cSOfvX2I0M11KVFxcPCQRxtMR8Pm44DYxKIPgTNRzJhLIA8QBN1j+EOrPcQ/PaN+/zQ9VAyvI+Z+bz2zBT95h7wL943+IN/2z8Ded8Ob8O4ZkLYjJOZzd/NBnZvgOF9pwmqE+wyyHJYm8VkNqORTlMMTGYdxgFoNxFv1ZrfBPAOMba4lvV2Ptq37dl6Umv9gvroOCGFaUHjtNkW64s00zgQjSXSY6BpNMhbA7PYfEu9ofj+farprxgwG+/DrPlfULSGmZ+wbPrbmPGjX7v9pPjHUSk0c1CJNhuvN/1l+TgM12cyq48zcBEBY7o20vdnq3l2nyONH4as3AfvKm16v78Cff1+aWPx0RHNn1Wgq5h4BLHZgOnNvMxykvfsmkGl16chupZqxuSePEUuGO1M3KBd3Ib3jm0ZcJsZuw7bFIx/K68z4oxwYI1U2jmMmUYz8UgiHMbE40irkZ5PmpALIHrwwYxpNEIgb9NqgMwhiJ5j9V6gM7RDZh5rEsNhrw/xkgPad/zFzCjCxjW+TwQp86nrZHYPU6SO92YeXcnMoPfl6x4yfzAV6fo6da3/9vft9WbbXfMNNpMd0+zR9QymaD8C0/5ds/doveSAeBsYv4QTuKt27pNvJfo8xlGOLn/k9Lw2BI4JBOjDxDRZk5pC4JPBONK0h9HXm1z0+K73tsYbXYfwZeUqoX7e+46Yw1N9pv7nWObbRawScN+b5jafADI+jH3j2ujYpvuv0bWcKDszTTXkN5pzAAAgAElEQVSfDTZ90CYGlmmih3d3jH4mkXl3HO3tT9au9x2jNg+zgmo4uzSawGPN+05sDYfljiXQSDCGs7eXknLAHGRwDljlKNJBDZUL0gGwOQ5iRp+e/8e8JQFospjYrBnsdyAqOp7oouiPJV3SzPPdte52oNyuWfcH47OuNTmziGGKRRvcG/hG1+E8G7w/m5e9awzlKqIwA2sZ9Cu7g1fee74/G7eSwbCv87t2Q2FgV6u9CoyZyQgcWmV5VW1Zy2xjs+fwfqdgLYCzEmWgiC5bUrruXlN+UcP0KzT07xOVNRTLBSJ8wuJ5Lf9Kl+2FGwoDJ+6wC2HhejDOpOgEMB/MOV4nJqH3E2sKNus/iIAo7C00MbUB/L4metdiXqkj9rL8Dnr1zo892ZpYBSkoZT6IDR8Zb1HOV8GYx4TDyQx05RnwHzazOeXX7i0aANs141Wl+D7HCTx6ypNz3hiqu9crNhSOoF3WNGL8G4DTiegwgEdE1/wHcYpuymgHeDszXlZK/R6IPFRTsnG7L9ORLEN3ZeMZIzs459OAXgjQUYA5E9rlYMsyXQe7OgS0MmiZ60auq5+zcedgt3ew21fVWDSFmH7NjMkgL05BnMks9pE5HF3n5qivxOu0WtQ44wzW7t0MnGJWzuOsXLJnkAAD7xNwt7ut9Yv1C55LaBEpg+pnZdWXPzI3vyN/z9GqMzKXiOaByJyIG71v8dgsHtHBi0RZaUxmlDKL3++BcNeWV975ZtNVr8TsuE+FuhWrph9lkf4SM88jkHGaFgyODZ2poJVymR0M2qjJ/WR96fotqaxt/s9ODI08bXQVa1zHoAlEPBIg4wSSb5y/4I1TIQyiNtbcSoR3GdSiFN9jj+p4evFHUrfRc8HqqecqRz0AL0ZN0sn0U+8w8LDOUd+un772rcE4l0uaUhICLnri7ILczsj/A+gaEE4g77BPQslsGKxxc9Rn4x3vmtoq1kzPtTq0idx6UVesjISU8AoxXmbWi+pmr1+fuJD0lTQb4UNHbD7MjQSmaOiLiOhUAGZ8Y96hXGbOoehasvSTsTdLBMzrLUWV95Y2vxF7sfhymo2AR6J9mmZ8FYTTiL2NJ+ab1v9Guviqktz9ETDRKonfYU3/PemJ+bdn6xqwOWCHUblHKLJnEOECED7MwGiv72XkgijE4KCMiQ/Z4LsJfO/u4e5ND0zZ2Nbfo5HK3/e96F5nwO2rGDg3lRUOKtmEiHL1xCWz12+uWj7tOA7xSxQNlpVQYo1f1M1u/syFGwrz8lutl8FkdjBJipFA2hzoDLpk1TnHh1TkSwws7BrsxKilZEueAHUy+BlFagW77rKdYfr78vnNrZmaYJnTMS+UFc2HSz8G46Tk7RMJCRAw29q2gLGaya1xw4Enls5buyOjIe0SMCLeIlc2luSEsWeiBn2MgIuZcfqgd5j3D8nson2JgaXadf6A8e2b6k8ZBA6NaqhPzCg6K2DjhwCdI07z/h+ENOUwe2a/VFPS/KPB3t+kiWdGqjGnYByNBiJfvuFhpejzS0rW/V+sxlQ0Tj1CaWXqnxRrGcmXXQS8kzxEV9Z1bYTOLu2yXxszln5l1hnDOyh4GlyaC8IcgE810XOyX/us1rANFi2snbXuL5nQ0ls4HDPsgr1Xonynq3+TReZMNMSBdZrN6kw31M2ORlJMRapYXXia0vYPSHMpyDthJin9BMxBhL8ydK3F/NBbVv5rfl8/dO2Gwrxdu+zFAC7x0zxmbCLi/5hY2rK8OnoyX1ISBExfbI0dNmfv9WhfA1SxiQ6QhLjuottcG7Pqz21+Ll5ZCxuLL9Oaf0fRE9RJJ2aqf0flLPL7+U5asS4B5rBd4L3RRyPsnKuJL2TmYjIbA8Xx6hdi75gyg79RW9byvVTMyS9/5PT8cCD3PwD8ZxIbT/yzVyR1E9gF6Etqy9avyhIkdO0DhbmtufaJrKgEwPzozVFsDlvKGDjBRmJGK1v60qUl6x9IUIQvxcSBnixGxn215c2fqFpV/O29oT2/kaS4p2vLmj9SuWrafCJ+SF6w+Gimy4G+sLFwomb7NwSY6w6kE4yvmfzOvYOBjUrzPbvb3foHLkzzjiQGLVpZNFdb9FsA5jSopAwTYEYbARs182/bM/FMpMF+EzFm9/tqlrbUNdCYCXNXmaQDCZjwb28yaLnS+Lnz3oRnB1xotx4WVawsnqwItUQ4TZo6ywgwPbWzk0uWZ0FYqSwjMzDUMVcwrSq+iRV+4OOYrmXiY/Onx7Qb3oRubyr6Epj+a2AAEy37IkDAyoLhzsfuyPDu+AHVQgy6fM2McU6nvoxJX0xMpzFgwpVK8osA4XG3ZEJpPdUfMsS8X9X1kEOVDcX/vjeE/vcQDQ8sKZsIEP4wsWR+ZTX5f+/05Y8UHR8OUD15i8aSMk7Ai95GL4K51g2EflE/8/Ftfh48qGws+ujevvuP3rUz/qa3QHSTW3LMHzLQf/lrSQalLWwsHKthf50Yl/vcF29jl86tm7Pu+XjMi54+d+8D6Lx4yh06L29jjbPrZre86J/M5CV5azY7g2dqcv4NUOZ6qOOSOXCXvEaDXsKjw4c7H71jykYT5tu3ZJ5Z1el8D5quJzJX60jKJgLEqA2p3KvuKm3KaLSli+4/uyB/WOSjAFUxYQrghfQXf5FPDwsDf9SlEyozOR4QB3ryjdlB0FOYrEYwj0tKHMN1oY61yP06QNcnJWsIFk6HA/3q+08u2DNsxN1EdPEQRJzFJpt7B/Vqrfn7reEdjy8//+W47x9NxLiKFYUTlGUvJ2ByIuWlTOoIEJvLm/ghDf0dbD9+40B2nnZTumHZ/NC2vO1naU1mQdJEvzB3YUrqhwAz7ySiH+29b3Gxu/WY1wfas1DRWDLM5vY6Bs6Xxs5CAsw7Wavp8S4gZaElQ1IlE8mjg9vvBzDXNwCEdtcKH1t/7lMmpPsh0/xlJ4ZG5o65nxnz+ssrv2c7Af4nIlxaO2/969muaab1MyFMrcNfGc+OdTGBvyIbAVPYImZ9QbWOrC99bncKa9lPtBeda9a0c8F8H4BR6apX6omHAP99IuVNqS5t6r4HN57CfeatWFE4Qin7T0Qo9UWgCPGbwN+J9FfGto9tvP18f+4Z98ZRuv0hRNvcV2cBAzs18yX15S0NfoMYCvIue3jmkU6w00Qvq0pBSPCGtqB98V/Oie9aRRN1yWJaAXihy31K5qJs9bGa8nUPRq9MzmwyjvMd79sn2YRrmXBtV5jvzCo1NGp/Nodyp/jtSK1aWfxJJvyeCHlDA+PAspIZ/wgxl949u2V72jU3V7k+Mn2UCrjlIPocAdNT0Nem3axsrJCZt2qVd0J9aVPa5jMHchAHevJPht4btvlekLejL+lEzLdz1Dl7TNLChpiAVDvQq7lavdC03Nx3/mPAu3dPUpYRMHdiM3CfS/rmVN/rZkyvaij6AohuAyd+dUOWIRxU6phITsR4ixg/dvLULxK5oytbgCx6tPgkHaCvEfP5DByWLXoNGD0IEWb8i5h+uEXlLG7K8A7VmLmZ06mriy+Dxp1J3xEXc6WSMU4C2tXuefWzN6yIs5xkzwICFY/NHGc54UaYu8d9StEoglxZV95S359I42ywlL0OhIn95ZXfs5wA411l45wls5pfynJNM6qecbZ0cvvVTPg8GMem4NRiRu3LxspJ44ia2c3vpEs3c+e5Uno5sUTNSRfz+OvhZ4a/5J51x3X+ntSrWjX1SpD6jVw1FH+LpKUEmfEJ3ifG70Oq89a7Sv/2vh/1Vq2aOoOJHiKQ3xFEGKCnXHIvSMfajh8sskVGxaqPHGUh524QZvn+PjIiUPry2pL1S+ONZlCxuuh426VGhvf99y2xxucnlTffnumQ/2bju0L7Z4nxma4TqH6Ey/eN0+AWxJvdHOtUP9f8qqur1fPnLn+ACPNhLm6TlI0EXiGNaekc5xoIZrPoi+cWn+cy30xmQxBBrp1K7dOxO4fotLtK172S2mr6li4O9EyR76NeJs/hIx1zAu2Sagf6ogfPGeXmhR8kkAndLil7CZiF6waX6cb68ubn4x3Ux2pWSWOJfYRuXxa9p1FSNhNgpk4QfppLOd+/q7TJl4WCdNl7ceMZI3M493ywroY/9/OmS/VsracDUPVE+N7JJWtfzPQkuz9I5r6tSCDvfoDL+8srv2eOADHdWFO+7vbMaSA1J0rA3H/uMtYCODpRGb2UYzB9q7Z8XXV/Mr2TOFptlBO4/ZEaEL9vc+GeU1+2YdOA0DbNSlYz1PONRacR07dAMKFb/Q73m2aLBkx13EGdo//sk6MsFqsXrCpaqAi/AyTUaSy8MpGHGY+9o3LL/b4zeOGq4hommNOukrKYAAOOApZ0Er5+X2nzG8mq6m0GtOylvkbz6aHUXmfr73SO+oyfjrFkbc7m8tF1qo7bQWxOPyu/dWVgZdse52OJXJ9YuWrGh4jcJp/H3XuXSnCLfm/CdzMWaY5BlauKTiKFbwP0cTlw5fdT1788Bl7WOep0P/uJyhXTJsHiZrn3vH/+mcvB/yJN09PoQKeqxmnHMvizpPkaEI3InO1DquY9RGpKTenaFzJltTjQM0Ve6vWdQKod6JeumH6aa7mrAEouVL/vlovAXggwAy+yxvVLZzebk2W+p4rHCydYEbsFwOG+CxeBqSAQYfDSsMbn/pSJ8D7xW0SXNhYf5Wr+MYg+KuHa4wd4iBLm9MUmBt+sy469L5P36PRnVUXj9BMV6yYCjuovr/yeUQLfry1r/lpGNZDKEyKwaNWMYzW55lvuZ2QPZtAP6srWfbk/pS5ZdeaxQQr+FcDo/vLK71lPYItNauY9pWtfznpN06ygdw9oa+BSF3zz3pOPx6e5+iFeHW8bPtwd7/edoH1B7dpg/CQIRUMcfFabz+B7Jz3WckV1NbRfinptz+3mZJCMWf2Cmlo5Zr3kUe0619bP2fhaUlUxaGFj8SUaqE3FHc9EaNWMy+vKms2VO5IORcBEL2sovowVfk6MAr9hMfh9ctXHa+esM07wuNOix6eeoCPKlPU34irjO+72CdUZcaAb5/nqqVOg1S8JONPvqwzihjxECzDhH7nILfIxhDtVNRbfBo2bQP5vRBmizZQKs58Oai5LRwh3sxl40+qiYnbpJyBMlXc9Fc3Zp8w9RM6UmtKN4kBPK3apbFASSLUDvXLl1EtB9Fsi2U0/UB4gcx8KNFXWzV73gs8n0Wlh49R5zGqZfDQHytPg6ekw46f6iNav1Z/yXDhbNTcLUIe7beft3c34YyKcmK16DnS9GGgD8EMrEPjJkplP7MhGexaunHoeK/UHQEJCZWP7dOtExL+qKW35VDbrKLr1TqBq+bTjENItPm+OZEB9p7Zs7c39cV/0+DknuJHI3wkY1l9e+T3rCbyq2Jq1pHzNq1mvaRoVvHRd8XBnD3+JiL4kJ7LSCP6Dqp5wt00oSZdT4dLVhac5rm1Oa+VmxFqpNCYCDL6trqzlK37eF3xpw5SzXbYaQBJdIqZGyI5MzEx/0BH6VP15a99LRiXveg60LWNQSSpCHSdz6jkZuwZa2Yo104+y2t1GEJ2UGt3pYZd2VdSXPpfQPbRVjdOOM9EiAX830xHwLWfbhG+l61vXzdZzqDVOn8lwfwvQh1LDXKTGSKB5Z8f2WcvPf7kzxvyHzHbthsK8na32g8Qo9UOeyEgRAebHXe1eWD9n484U1eCJrVg6OWiNGb4IxP8DYFQq6xLZvRGg3czqjLryNf/MFB85gZ4p8lKv7wRS7UCvWlX0XwB9UXaf+d50qRRoriJ9LmLRx//o552UZmdvY/F3AXw1lcqLbP8JMKMNzN98x8r7md9hC/3Q1iw+dKD9s2B80ecTkX6oN+hkMLgToAcjbP3HfVno9FjYUPxpZr4dRL6H3xt0jZlZg+pqy5olZGlm2yCh2lNxAt0MPIjxpdry5h/2p9Si1cUnaQdPg5DTX175PcsJMF5yoUrry9e+meWapk29hY2FY7VrmVMan5QNyGnDvl9FTPSbSavPu666utq3k8aHsqSqcdqVYL5TNhhnpr1jrHVvtGP+ytKylttizB9TtqrGad+A1rfKmDUmXNmUyWXi3+mtu79QvyAxp2i3MYtWF5+vXdTt3aCcik2BYSa+cdLqll/7GTkhmxrCD12qmqb9OzT/AoDth7z9PyisFVC1pKzlD4keTqlaPfUYdlSD74cEXP1Nd8dx30+3A33h8rNORyhQw8Bk33mLwDgJ8Gp32+659Qv8OSizcGXx4azwGIAPx6mIZE8vgb/s2eMsTORKiVjVvGHZiaFtOWNuYnhrtOI8jxWcj/kYtFtb+vT6WS3/8lFsXKLEgR4XLsmczQRS6UA3oQd37bTuAdGCbGYguvVOgMB3hijvM36F86muhnph5rT7QWxCa0sacAToPTBdWFu+dk02qX7R/ScX5I4Y8Q1ofJFA+77P2aTj4NWFnyDwp2pK1z+X6IKA32wqllZYasxrPyLC5/yWLfL8JWBOxdSVNc/xV6pISweBihWFE2zLXsfAkX7VZxzoNnDNvWXNxol0yFTRMOVki9XTIJL7oPuDlfW/0zOBkCpffPaad7Ne1TQouOjxc0a5Tvh2Yro0DdVJFX0RYPpmbdm676ZrbFPVUPxTADdKg2Q1AQ3CjbWlzT/3S8tqrlYvNj68RIMr/ZIpctJKYDfA19WWttQk01eYiCNuO90H5vJUaM+MN0nRObWl68xVAZIOIHDhhsK8/F3WCoBmpAIOERodtF6U6Olzo1PVE2ePR9hZBWCivzry191tx/53Oh3olzYWH+1qvg9EJpSzpMwTeHT4cOejfl1Zc/nqouMjLplrvsZm3jTRoE8CjHuGj3Cu9qvdD6yn4tnJQeudgi+DcQsIlrREZgiIAz0z3KXWwUqA6PhUDaYrGkuG2dy+fO8i+TmDFd9gtouZ20ipq2tL15kd0Uknb0PFLnsdgLOSFiYCMkGAmehBfdiuT2ZLKPeKh6ePVgHXXBFxntx3nolHApqZX1aMK2vKW9Yls3jkl/Zdg/X7AFzgl0yRkxoCzNhUV97s80JQanQVqfsTMA50y/K+57460MFcWVfeUt8f78oV0yaR4n/IhLw/UgPhd3Ggd7fS/JdODI14ffSvATKROQIDofUGq45k4ws1M5t/mq5xTVVD0XLAG8tKyl4CGqAv1Jat+5lfKnoRtHTbwyCa5ZdMkZNeAgz+lyZnbn3pX19OuGZzF3RD8ZVEuCMVJ6AZcBTzTTXlLbcnrOMgLriooegKDfpNSr67jA5i/fGa2esfTgZhRePUIxTUo8Q4LRk5B5UlfNHdOuHH6XKge1cW6PZfMOH/AXI/tq9tmagwworhBc4FfjlSKxtnnErsPgFgRKIqSbm0ELhzC+Vel4roouZqzSPR/jnWuBmE4WmxRirpi8BuZktCuMvzIQT8IOCyOjpVYRPNKQodDj8OolP80FVkZITAq8xOeV35xqTvzKhonDxMcYG5309CNWWkKf2olDu1pmuWljffm65Fxb60jt4Pip8Q4UoJeelH2yYhg/GSJrqqrnTdGiJwEpKSLlqxZnqu6tCPE1CYtDARkFICBGx/m3KPSMXELaWKi3B4p0cYawEc7RcOBjPZPKf23PXmhM0hU8XK4smWoqcBlh3t/cHK+t/5nxZRyb2lzW9kvaopVLBrk+mtAH8ZkOtHUog6VtHfrylt/no6xjRmofFwbl9LwJRYlZN8GSBgxreabq4tX/cdv2q//JHT88OB3CcJ+IhfMkVO2gkwayxua3euTyYUrjl4YnH7SgDFqbCAmZ8PM2b+aXbL9lTIH6gyL3u48EgnaJu7xVOyoZeAR3LH2xV3TnyyNRlGFY/NHGe7kUeY+cxk5BxYlhX9x6RZ635STUjTdSXFn2TGnQQU+GmHyEqKwBPu4a3lfh2QqVw5daqy1CpmaeOkWiXlhWnpzo5RVyw/f3mnn1WZqLMvnlO8QCuYiHK5fsoWWQkQYLSxsorrStc8k0BpX4pICHdfMIqQbCCQSgd6xWNnjlNOcCMBx2SDraJDQgTMmva3aspabk12Eck7LRx01xJI7sNJqCmypRA9Y9uRufecu/HtTGnkOc/b8BMCLkvJbvFMGTZQ6zWLisz/Aql/qy1ZtzqTmyuu3VCYt3OX/QwBxw9UnENFb2bstDsx4d7zm3cNFZsHi51dDnRznYeP4ztzRyQVLSlr3tgfJ3Gg90doAP1OtDWCyLl/LN34wgDS2ndVK1cVzyVCrdwR6DvahASaK0b0tgnnpeNUntn4Z3e4axh0RkLKSqG0ESDgF0tKmz+b7Jy4W+FLlxUPd3J4I4FOTJsRUpH/BBjvaaKLlpatezIZ4ZUrpl0CxYuJkJeMnD7Kuhr4Jm+bcFs6+rUU6J8SkZUNRVcQ6NcAUnIlEEP9v7rStYuTnRt/fGXRmJAic4rd141WpPjGk2e1/DwdDnRjQ1BhFYFkw1BKnuYEhRI9Ne7oUdNvP8kfR2rVqqkzQOpRAPkJaiTF0kKAV1sddJHf6zBmjm4rvo9BJ6fFDKmkPwLtCpgZy/pKf4IS/X2fA93ctWmNfX0VwBJ2KVGaUi6jBFLpQF+4svhwVvw3gI7IqJFSeZIE6BnXjZxTP2fjzmQELWwsHMvaXgPCScnIkbIZJ9AB5kW15S1/yoQmJkS32lJgNnR8JRP1S52HIMB4Xim9aEnp+r9lipN3msfOeZlIvjuZaoNY6/Uc6PmYcO80caDHyixb8lU9evZ42I5ZKD7OP51Ys8akutktL/Yns3Jl0Yeh8DSBQv3lld+znsAu1np23ez167Ne0xQp6G0IITSDMCxFVYjYOAloYPs/X3KO3HjdxkicRePObiJ0WVxg+tPT4y4sBdJLgPlPE8taPumXs+nixjNGhnTwGSI6Kr2GSG0+E9DQ+v/c8uM+V0/1bqKyr1hZNCas6H4AZycq41DlmOkti92ZS2av35wK+QNN5vxlJ4ZG5IxZBqAsFboz+JlOCs/8c+nf3k9W/qIHzxml8yLL/Y5QwMw3TCpr+YVffVpfdnZFWvkmMX8DJFF2kn0e/CzP4E16m3Vm/YK17X7IrVpZNBOKzLMqDnQ/gKZIBjOe153hWfXnP7XVryqiB+Z0HQGz/ZIpcpIm0A7ic2tLWzYkLSlBAfsc6CY8wQuzileBUZKgLCkmBDJKIJUOdHNXjw36BzONzaiRUnmSBLiTXbq+bk7zXUDi4Zk//siMw4K2s4aIPpSkQlI8swSYgfvqSpsrkt1NHbcZDFXZVPTviukHLKG/4saX6gIMMDH/zVbWgntK1yZ+F2ASil7+yNz8SOD91wEalYQYKZoWArx1C+WNlxDuaYHtayXGgc4B5wliPyM9sA6yNeHu8rVv9qds1fJpx1GIn2JgZH955fesJ7AbrOfVlq83EQ2GXIregdz+a5AXUUdS9hBgN0fl18/wZ0H5UGaJAz17Gr1fTRjL3e0TLvTrBO+iB08b5eblPkdy2KBf9FmfgbGLmafGsgmwT1sYVNU0/WqwNiei9605+2i7Yzag15Q2/8hHmQNWVOXK4ipS+B2AnBQYsZu0rqgpX/+IH+slFSsKR1jKXg7CdD91ZeC6SY81/6a6OrUh3L073LV6lMjnO9z9hDFkZfFmN8c61a/xzoJVU88lqOUpiqQxZFvJd8MZrxKjuGZ28zt+yDaHi+2xr32JGd8GQa5Y8wOqDzIYaAN4Rl1Zy999EJeQiHQ60JmAnWZ3yN6J9csM9Q9F+IfWWsMiSzk6pBUrxXbIZW0pcAikFEOHiFiZkxlMUEwU0lpbFlOIiBQDIQaTAnkPtkbXA87RuwTJImWMZM2WN3TT0XzU9SJwVznuvnuwqxy65Jg6omSj5UBddxQym3+n6N1uTN7uMzZ/ZwUmAiH6u9E5+u/RekEa5l4Wbf6VzP0sZp1eM8iU0jA3D4GYjVHEmsyfySzkw8vDMP8W/U2bnESaTe6oXFZMOpqfvb9rk5+jss0/EpOGMhWyNrAUkQmBZJT1dphqRS60Ntp0EoNdpTvApElRB+2Nf61c7tAwf1Ad5GqGUh2sSCs2/24gqw6XXVZQnWAcw4qmgnACGKcCOBKAndCTGkOhlDvQmZ5h0JgYVJEs2U2gxaXc8vrSpt2JqvnxJ2ccFuwQB3qi/LKrHG9XDk1fMrf5pbTpxaDKhuI5ivAHcZ6njXoiFZlw7g+Rcq+qKd24LREByZQRB3oy9NJbloBlNWXNF6S3VqnNDwLeQhirxwnwL+wsGwc6Drs7hvs5K5bNHGflhM2J5WP9sEdkZJTAThcor48hdH9GtUxR5ZVN0+aT5j/KPYEpApy42A738NYRft0Jeig1PAc6Cp4Ayz3YiTdX2krWTSydv6iaqn25Lzh6qjS8CaBxabNAKkoZAWL+7sllLTcnc5rX3HVtRTobQXRKShQlesrtpNn15619LyXyB4jQRY+fM0o74VVg8vVO8Q/M5zWu656fbATHbnnmugc3hGUgn6MTEF9Tu7rld0ixA72qsfiTYCxO0WaFAfLUZa2aL+RQ7pl3lTZ1+KGhOND9oJh6Gcx4Tilnll/rdZesmnFsULkrwBJtNvWtF3sNJuJjJBCcdN+5j2fs+tW0ONCZ0aaI/5eIayIhe5NfO4JiR536nBVLYW0dV0Ljtr6rdg8L07CR41TH9jDlDCtQnYEIhdo7PEd8MGJzODdX54Tf446CfH3EuJF6y1Ov8tZxh+lxW5u4vgKe4zv1GqevBrODR43ZfByTKlSwvgdwSk7tigM9fW06sGviCGnMqpndsjZRO8SBnii5rCxn+twba0ubf54u7SrWzBut2nc+TMRT01Wn1JMwgTAzbtfbJ3zZr1M6sWoiDvRYSWU4H8Nl0gvqytbfl2FNpPoECBgHugX1mK+TZGbt5lrDYpnvmDuDrQ53OUByhVYC7ZdlRV5RbJUsKV/zapbplXJ1TPjYgpzR65Tcfe2501kAACAASURBVJ1y1vFWwMyP6e3HlqVjDFOxdHquGuuuIXkO4m2m9OY3hywY31la1nyzXxWLA90vklkiR2Oty878ZJ2mlQ3TLiHwvSlxNnoHjPTX68rW//dgWz+N5ynouvv8NwAC8ZSLJa/ZSU6Kr5vYtP63fp3srmgsGaa47SECnRuLDjHnYX1Vbdn636fyWbjwgcK8Yfn2/Uwojx6ek5RdBHi1u2333PoFz4X90Esc6H5QTL0MIn6gYLdbdceFG9uSra1i6eSgNbrgf6Dwqe5DtcnKlPI+EWC0uEe0zkzHhuC+NE61A93saF3jalxXP7v5OZ+wiZgBTMCcu1/YVHwbGNf7fZeIONAH8IORTtVNvAbiL9eUtvwg0QG2ONDT2WBpqIvxqLt9wvnpWFz07rUO5v6IGNcMkEGZ2dBlIpSY73lX1BS4YHSCOAyQ4/3O3m+uicdi7DLhWQA2EUcCAAUBDnZFbFHR//eitHRFcklDGydXRQeDr9Pbjr03Hc9It6riQE+u0dJWmvmp/Fxr9m9nDO0TMGnj7XNFC1cWH64VVhNwsm+iGc64ztHDbj9/eWe/Mr1x8dSbwOo2jvaJkgYoAQYW68Nbr8nkxD4j6ExUnaaizxDTz1IUqjcjZg2OStlElLuqprylJh32eGH80bYmdSch02HFkKgjAtAna8vW/cUva8WB7hfJ7JBDwLuanfl15Rv/moxG3j2yAfdhIkrRpnF6Ocxq9n1DcOOa1y4mVH5D8UMgzE+mnfoqy8DfdY4qq/dxjmPWQiJ2zkMgfzeOKktftuTc9UsSXd+Lhd+ixhlnuNp9UkJ6x0Ir/XlY822Tnmj5ql+bPcSBnv42jLdGBncScHVtWcuSeMv2lv8TDdOKA0ATwKm4DsMPFYeoDO4Eqc/Xlqz7VSr7+P7gptKBbhbdf+OG1VeGelid/hphqP1+7YbCwM4d9vnKolr2sWMSB/pQe5ISt5cItW8j9/JE76sVB3ri7LOzJL8GUrNqS9e9klL9jKNkdVEVu3RP1zUfKa0uLuHmFC3YISKzc/N1Jn5VMb0OhXeY1XYwdgDuTs3cqhS1kUthzRSxguxAaTfSRuzathcGMocjlkuaELQsm8lmVwd12Aq5ts63FYYDagSzHg1gLDEdCcIxmnAcaT4SRGb3vHG8Z5sj6UWl9fwls9dvjotrEpnFgZ4EvPQUNVfwbNOgK5eWNpu7/AZV9KD0IMx8LZc/OeOwSKe7GsBE37RhhCceMaGg+pT6mE5AXNl4xsgODt0DeAug2db3+YZlEAsyt2e9aCHwsXvKntw0iO3s1TRzmszSbfeDqGyo2Z7N9poPFBH92S2IXFU/ZePOdOgqDvR0UE62DjKbYuusPL7+3mnNu5KV1l1eHOh+kcwSOd51kPTvtWXNdyajUTVXq+cbln+JCN9J0cZxh5hvqilvuT0ZPQdq2QUNRfMUyFydku+7DYwwM11dN3udiSDgWzKnuPPz7AdA8HXMQIoX1cxqqU3lfGxhQ/FXGfiebzBEkF8EzBx8E0XUx2vmrX3BL6HiQPeLZKrkcJhZ3ZGrtnzxrtJXkg7bX9JYYh/B7T8CcGOqNBa58RMw85m9d58/Go7YV/xp3pp345fgX4kUOtDpSaLIxX7dQ+CfySIpWwhUriy6joh+BkLQD53Ege4HxSEig/mpwNiRMxd/5NE9iVicBgf6fqd+GdxriCgyoaOI+g4fNQgCS5moFdHTzLASaasYy+zRLn9i6ZyWR2LMn1C2hY0lY5k77t87AJiRkAB/CnU/W+8D2MbAGwR+k4meIthPuiH9bCxhh/1RZX8pV6wsGtNJVEiEc8B8MoiOAsM42Md2LQyk8hnozyTD7Z7he5xP+REeqr/KzO/pc6CzBshloIOAMOD93bxz5n/K9D/EpLoC1XVHDejp5KOefRQxmTgEh+x92KsjvSmqkBf94INk4iT0CMG3n9Je/7pfbvMvzMx7CLSNGRscGzf/cVbzS+m1RGrzk4B3R6cTbgTg2x2drLlt0pHHjorVgW7sqVg6eZg1uuBHrOg8Ah/mRe/grm8/mVfMeyPNf6N9OLPLZHYqeVc/ffA+aVjd7yozlHkbmVmZwYL3RPf2ZnpSva+tiSji/Y8Ah8Gul59NFBFvcdZscMpEP2wcPmZhZA8YEY8DQzFxAIwAkWFFAabo5oP+hj7dO12oe9NLl/3GC97FOdpbdPUZXuCi7ocu2i90izA6bSWNZtb2f9TOffItP5/NgSKr8rFpZ5HDZhPKsDTrbKLLmubx+uau52KfCr1+Z7zGpIO+P+x96Hq8Rwcbog+5RUp5kXqMOtHvZX8p+kAdlM9EofDGvN63ibue5y55Pb5XvTzj0aeSoAm8h5neBnFTDuV97a7SJjPeS0tKowO9eyxrNimZSB892zQ6XmEo81SYyEhd8wjDoK/+a79xTDcs03129blx8vPGUclsqusZoWmfblFTurumD8YvB/RPvelqdGnbG03pbQLdt4Vyb050E3lfINLkQDfvuQOmToY2m37NmOyDd858A8x3Mzo39r593R+E/djF8qHoMrTnGPFQD8G+70lfmWJ5Grx42aZP6zv1GMumIZIX/19tWcun43z4D8p++d9Pz49sz/krQB9OVlYf5TcEIiNKFs9LbF0nRTqlXGzFmumjrTb9BBQmpaQypjVB1hfdPbtlu5/yvauL2vWDfjvQQaioLWn+Y6oc6NduKMzbtdOqA9FH/eTRh6zo+MaM/72u3huIRseo3am3/iLOMY630W5/qZ50Jtamfz1It2jEwVjTQbOOnv31vm9ztM/2xjvdfTaZ+XrvY56ewx+jXwSAOejRomDd5PcVSmlyoJv2dSk6ljFjmj7HM90HcLrndz0aYv+1GO+HPsaiMXxnYm3gePP1uXbd1ard39tDzePM3JSgdjH4NQB/CUbaf7R43tMJrekfqP9Vj80c1xYJNxFhcry2JZnfvIXR8eYB85kD5zK9j0sPHnP29m6beY431+2ZuutN0ACvl9o30uoxD9o3zzFrGV3vN1h1rxOarsp7Sj+o98BmN3qaefYWZjyuGF+tmd38ToJq+lYsJQ50c+e5jqiP1J+39mXfNBVBg46At/twmP0wGDP9MG4AO9DNR/N9IrxF4F1gMgOB/fs15u5TmQej8maPg+fE0t4dyub0qTmdOj51J7F4G2k6NdFOOLUOdN4JphWs+QEN2mxZFHG17nUxzrYBV6vef2PXchjBjCx1+/FCd8mwlQpq5pNJ42JmmubXhpv9xw0wu9dvqC1v+aWPqu8nqpqhXmgsrt47MP5aRhwQ0RPmbxLRWgLqdcTdpIO0Dcjfja3j2tMZmjwWxub+oci4YF7AsYYHLHucSzwdGhV7dT8FRKZ/6M9HEks18eaJMPiaurKWu+MtmEj+NDjQzSDdjNPuV8wrI6BtcFXYthytI0xaMSnLii7gW1qxYxMprYzbzgpGHXvaYWKySZvg/V3JIiZNJop/70lZxG7E+OvTm3rtL8nNtVxF2uIQsVJK6RC7sF1GUFmwCbA974xWBHZ3KaK3HZffCwbUtvBWtb1+wdr29FohtflNwGxsAjoamPk0v2Qn4kA3dRvn0y53z/igZU1g6FPh0jgoylGK3iettzOw3YHeplzVSTY7LhNbltLsuvsWW/Z7V80sVVvG0UykldLGlaQ5CEvvcyKZHTIaVod5sRVsxwU7itlRgBNWrucUhBUIqrDOh0IJiC4l1pO7F7v8YtabHAYcxXieFe5mB09owq6QpcIRTWzZrooQAsphz4FOLgIuOF8FKUQO5RBRjuNqM54003YzvQ+brsdxjAPeW3zpZKW1rQNhh7RrKa0dBzD9U3d/Zgq7SilizgfRMJCbC1b5pKx2dpwdlqK3wda2SC6/m6mNZ6nkH4vs6mqoTTOL72VCVSz5fcnDeA+EN8B4EYqeJY03mJx3AdpDpDrNe2HqYa0PWug17WtZH7wv3fpEIoClVJ8Lw6yVJtvp87vF7gdlzTvYn53d39MD85nxfiBgvq02dY/9zbtrZka25qBLCDB0iMgKWEpbbgS5ZJFNxAGOqE4O8VuW1u/qSPDdLYHAu347SfuzKx0OdGZ+f29f1GROXZJWL7rkduoefeC+cYurldmi35O1MvOpXm4JdjutaP94QDLPC7HDyo7HGR5AT336Y9bb7z317KmbRRZ16xl9HpwAQ4Vsi3K0tgKaOGAzh7SiHDAHTb8I4t0O09sBhX9FWG0ZNSL87h1TNh601pCInj3LpNqBTqAdLrMJVf1nRfyqG2Gnu116fveMToafpg/eIe8z1oMdO65Ngdjuiw4opSKODvXFR5mNbFqHXesQz4jr7XTrNIPMQ3Hu/g4dKo/5LrGy8ljrk0E8H0TnEDAy2fbrtTzz4+72Y0v9mCNWNhZdR0zmlLjv93R7TjQXn5o4u/mu6kNvhEoJpkwJrVxR/DFSvBTkbXL0PWmXb1r6ZMtPUR2X07RfPW546cTQ1tfGmBPoc/rNHE+GFDvQq1ZPPYZd9f/Z+w74uIpr/e/MXa0kFxlsTEmoLxRjEkps7Uqu2pVcKQ8IsiQbCAktyQvJ+6e+NBB5yUtPXl5eEkLyCAnYWmnpBmOwpJWrtCs7dDC9BmxcwE1ld++cv2dlGzdZW2Z2Ve7NLxi8M9+c+Wb27sx8c85ZpjXl06H96wTjHRC9SpDPS6K3iHkTS2wnwZ02iW71q5R4z7GttuQHrEt6frOIxUHrld7WOD37iEPXRLYkVpuHg82TtiV7NN6+H5c6HxAH/q6q3y+1K1G1bdtKmJvoy551k/o7tQYntSWP2wXqPc3MbkhXoYQssKyPLvISaFs8Rm+zpI0FtOs9XSLq/j0zLaAzeBOBlrLgxYDrDch4dH+O9z+HSfCk+CFOrA/VWnHvc/AaZu/+5XCjlMzvTN+jm16J3s6uLZb5tmBhqTMYtbcji1jIfKW2clzkS3URXCSiKnXA5o0keGu8q+D9c+as3KLznV/TXDKPme8ycd58WMYkR9V+hgmvCxZPEvBqXMj3RIx3SJeV8Kg/eC9zuO+4HVVfrAO/r2rfon4aab/1q5o8h7wzpFrl9r6/6XOk3W4I296379n7HT/43HDvviZxTgi48iAKbLILCK4C9d0mIfI5bg8jQYJYxG3id0nIt2RMbjw+tm3j7+a+0ndavD6NzbyAEQGdwL+o80e+lbl5DsJgZ6Cq2XMegdbuCdmbUXcHqIC+lSX/H/LEX91dHW/dNetpFT45NWGBQZXByj4PazIiN4uVd47Y6Soc8f7ofJk3kW35HRCVGmg+RgR/nS+8Kh1sUwI6MV4EyRvjm9tXBef1eLM4Tw8DiUM52VlJhJ8xcIJmXqQE/bTB3/Y9zbj74CofLz5fWBQiIjOHHIcxXIkPxFC3M8MgCtAwDtctDW/SvRE2xdnBuCqs0sdinafZlqwgiEtBfAEYY7Ih5Oy1hcFPStv+1+CMdYpXo48KiytkxztENEp7Q4ztRPgpKP5nJ1KQdnYdwAHEQE1owjFSupqJoE9AZ+yUx588JphkCPcBRBcWLPEWyUJcv/vmp7oQZszjmIEdQuAnogO/XzhXX5jhgcT1QLD1mlDx8Z1MTxNorEF71UXjjYJlu2TUIyrb5FHud4fqpQWDPGcMXfnceLe1cWTrbq+gT2cMdiiAuvS3CkzftDeftFaHqGfAxiELaVhAfyovj268a0pb+wERV4Ys2z0dV+HRX2551GMz/wpInJdovVzMwCvd27qLH7jsyYyjWFSGio8XLB4l4HxDw/a2zaI0WN76T0P4/QpWXTS3jhkZBHCJGcP4VZt4StDXvkE3/k1LTs/fVDDmIQAztWILcXlgeusDpjzQq0LeEmI8DGCMVrt7wDYD/CCY79jhzn/h4SmrlHNVamfCBowa6pDVy0rKYPEjAIZp5iLGjEcEye+ftaL9BV052zXbOKTg1FlzJ3c8TKBywx3fBeaXQPSwiOFBl8Vv/L08oi4GO993w8TrgNcuoKsDDwbNafC3rdZhoIMx+BmobvY0QsOLagAK6FFmeYvccuovnEOAw8/zK0Olp8elulnLF2j9JuwmngVurvdFfpwOrikBHcxfHLcycruziDr8qNSGylwvovPLzPi17kMCgP8W8EeuSWc+9FVHCb/Hya4/EvF1fZXV8XkiMhPoaWb8Fsyr5dZTXh1s75hEzlV0jGOJS4nEDWAeq/fY6PAjkfCIJPxHnS+s8iMZfS55cPLIYSNjGwDSvWlL5Av8sHvM7Y/OfbRf3OY0SqQD7jBwBAZU6oiYoEbWeKjLjG1yy8nHBucllwN9oA3QDWsn5O3clrdAEv8RQIEB+6MQ9OUNXPDXbHvQGujLoIasai65nMD36F+T7aMtBqKFgPz9ts6tzzzaTzwQBvWgZtA5tU5fz51KQJ+YAUwvCzB+Oco8+76K9te0YzuAGTNwTajsqE7Z8QIRHZ8x2AEA/B4Dc+r9kaf04g4etKpGz5lk0cNgnKGzVwz+ULL1SR2itIpW8vw0zzcE6KeGfi+iuyOtXZ+tKGE6eU4Ha16j1ycSXqukPfc5g7uFoC/WtYT/ZuLSvSkB3ZJ88cKKiBK4jTzzlnk/QwKBPdEy9bXB2Lo7EukN8c07FgfnPZ+ce7e+1h2kIzBQ0+TxM5GaU4U6iSJGCIyadKOh6rTFwephoGp5ydmwWUXq1O+40tOEugS6fncyhlvJ5pa68vD7jmg+8GafdgEd4FddUXvq3bPXvTfw6HAszgUDVU3e/yDCTzJtu4DkCXcauCWp7FK3Zl1MzzJI241DBv1TEFXU+VrXZ9r3wVy/qrG4GEI8rjk8mVIYH6z3hS9LhzsTAjoDHSDbW+9b+2w6Ng2VOsrDwR4eW06sz1tQcbc773ZjnS+sN5TYnkGpfvT8U5Gf3wLgFKPj1JMb600w3Uki9vsh4VnMoCtXTz4zFo19l5guBaHIKMc969+3o+yael/5mjdNtqUE9OFF8Y3MejdtAC/fQMMqHGHK5Og52AOFAUdAT2+kKhsqLWvMm4+DyJ8ewhFqES0tQMFld/paEuHrnKd/MqC8H9eHltwO0LUmLFSX8ncnHq8N+iK/cQ6ZTDCsH9OggK4Sy3yu3te+UL/VDqIOBirXThhF26znBNHHdeDtxSDgT+9R4ZedNeuRWa1p8n6OwbdpDefNLEnY59T51mk5q7q8adIpboqvBOgknXPkIyxanhfruNBEKGcz9qaHWhkaP0LIkY8QYVp6CEeuxcCTMrqzPDj7ua0m8AeqgF7d7P3B7ijaP9TKCcMGyVs30PCfOO84rcxqATMhoCciRNr82UBFpM5Z22oZJi0gVY3FC0iIu7WAHQpig9Fg50W/Gpz2xCZDbTiwWWDAgICOiL355EmDzdstC2MxZJuobim9DFI2ZBrGnSg+1pRgZEJAB/hZkeeetmjqqg+G7OAn0XF1QPdC6NHHCKhIonjyRYiesI89qSSdEKsmBHQA7wu2PIsMi3LJE9RPSzKoqsX7bWL8SHMu8acD/vB5untd2QBLjPH+kghfUamLdOPvw2OVXo9XMouvyS0nPTXUfoMvXjxh2PBC11wI/BzAacZ47gFWabH/u6go9m0TeST32m5IQLd3exZ8p94f+YVhjhx4h4EBwUDl0tLRVh436ox0M9g90PcObFVTyReJ+H91/7YxcGO9P3z7gJhAQ9jIBW3eIrsDjwEo0U0DMaRk3DTqqPifTf7O6rZ7qOOZEtAJeMeCq+Ju/+oXhzrH/bX/xgR05q/UlUfU74wT3vQIg18TKh3H4KVg1npZm6SYU1fe+pgmoYeqmkq+QMS/NZILnSFJ8OfryiJ/12Rvv/y6VTd5LoOghdB+wbpnjyvB/zHeN/eXtVQrTRCgohht32E9BKbZOvFZ2HPry9Y+qhNzf6zqJk89iObpxGfml6KMSfdXRLboxHWw9DBgQkAHaBNJ/pTjfa5njHSg1DJ2Xwj2/BKg/6cD7yCMOEssElbh1+t8LZsN4DuQWWTgQAF9mrcJQFkm7TNwb70/fEUmGE7docVATXPpdIZUoVEyyqU44AR0xlNWN6Y5uR37nu9VIc/3iEkJpvoewsvCledN5wKDEQGd8b6AI6AnM8DzQ8XnSxbKo1tbiB0G/lnvD5+YTPuplLmyeeJZMYh2Ao1MpV4qZXtynXNdUYf9hdsvXteRSt1BVpaqm4pLQeKvQCKModZcgPtzxcBLZMmKwPT2t01xaERAJ3QKFpct8rcq0cN5HAaGPAM9eVtjj+sMOczMH8otpxw3WEO4750081dM9Mi4tRKAW+NEYktY3oVla9o1YjpQBhioXl58EmyxBoDutRNL4K/jV8y5vrbWzAG+ATocyN0MmBLQAX7aduVXBKetdDx3+ulMMyWgg1AZ8IVVmgjnOQIDyivZxSPvZ80OB4L4pjOXR/6gK71cZVPpxy2Sag9yjqEB/QdRfJYppxpDNicNq0Lhr5/mXaQiDiddKZWCxG8L6Zpq0qEjIaBvcz0IwpxUTOurrCSa0eBra+yrXJqfU02TZx0TaU4ribsD5eGr0rTJqWaYATMCOreNWxGZrOudapiCIQFfuaa00Oq279N9qaeHPF5uF1hzgpNaO4cEmYO8kwccLtc0eZuZ4Mukzwz6v3p/W1byvGZip1O3/zAwv6nUwyQfY+CoTKwaaAI6g5+1HA/0pIa8ellJGVu8jABXUhWSK/RPuF2ewJTV7yZX/KNSlSumjhXx6BoCTk+1bm/lGdgGyZ76ishLujAHK44KuRu1aClYZ45F3mb7ThkTpKCtjTcGVYc8/w6Qytlu6okxiz9YbuvWdC6DmDIqV7jqBulLa4rPlV3iAZDBkPmMKIH/ra488hdTfTUioANbYNkzAtPXPmHKbgfXYWAgMWBCQAd4i71558cGey7D+U2TTrGF/RSxxstsDFsKeWLQUEqmgTQ3+7utlY8Xn29ZYrmB1Cm7SFgz6srWqFzazjOAGDAooK+xbXtucMa6bQOIjiFlqikB3ZZyTrCifemQIjPNzlY1e28m4NY0qx+2GjF+Fd9y8rd1RTZLpH8Z/eYPIei7Ou3cDysGphsC5W13GsLPKey8xydOJpf1sObUhnv7ZEPILwamt//FpAf/QBTQL147Ydjw7S6VykBv+gHGNwPl4V/mdFI5jffKgBEBnejuurK2q0mlXnSefsHA/JVTjpbRaBuIztRrEHer4Kn1/vCDenEdtFwxcICAXt2c8EDPKJcdM/2lvrzt+lx1yGl34DFQ1VJcLKR4fKgJ6ABeQtzlC8xMXcAdeKOcmcU1bd7j5C5eR1rzqvFm24InOD3yeqrWJQRcgTWAzh9Z7iZJnrqK8NOp2jPUyquN147trj8x8Dl9faed7uHy1L979YXQuiZUVtCNrsXMrDf9wEedVqHV/jhuxZyvOF5aB86E6uaSSwBWnuij9c2RQ5DabCqcEfS17DTRhhkBnd9zkTXtbl/rKyZsdjAdBgYaA9eEyo7qkl2Pg7hYn+1DQ0D/3IqpYzvjXU8B4gRt3DGiRaPiI5yw3doYNQakDhYlkUqxpPNyq7J3/a5d8QmLh3ZEHWPjZhLYlIDO4BWShl1oar1lkpOhgm1KQCe2yuvK1zQPFR4z6eeC5pLJNnELWOs7ud7efPICXQK66l91qORUacs1QpC+tcP+xDGWje3ecvHv5r7SnQmf/a1uZahshOCOewk004RtzHhGCjnT9AVGQwI6A3JGwN+u9Aztz57ICet2n7scpxE8xhJX11eEAxoxHSiNDBgR0MF/DPgi/2bykopGCoYE1GdCpePypFQXgo/V2WEG2qTBs0KdtjpYyTHgCOjJ8eSUMsjAUBXQGXhdxrg8OCt1AdfgcPRLaBWu6vkp3oeEwIX6DORtLJGWx7cZAR0ScfYGZkbW6uvjIEVSV/mavV8lwm+09ZCxy3a7xgenrn5LF+aCRu94W2AVgKN1Ye6Ho26t3m93Rb8QnPuEE9LyIILnLDk9f1TBmO8B/D2ATOWejwmBWYvKwiED4wszAjreAdHUgK/tDRM2O5gOAwONgcplE0ZZlkt5t2nL48zAh3LzyYM+hLvKH0959hOC6GR9486bAv6I1gMMfbY5SPszUNPs/RIDv9fNigSubfCH1QU4xztHN7mG8UwJ6IkQmDTsIkdANzyAGcCbEtCFcPkXla02ss7OoLv9smpC5IOMgPAxfQbyqg00zNfia4nrwlTnOi9MLfkOEddC/wUsZWYnQNUBX9viwSRSVTV5ZwL8EBHl6xqLA3AYP7K3nFyr87LE4eysfG6827Vx5EMMzNLVD2bYVgwzFs02sye/ctXks+LRuEpZo/Ni/k4JvqLBH3HSqumaCJpxTAjoJPGHuvLwlwfTu0kz7VmHU9FuYfHiTFMKH2Q4g+jzAd/gjIaS9UHqJw06Ano/GYihbMbQFdD5bStO5Ytmhl8eyuOfbN+rmifeQLD+lGz5vsoxYQcEeeunt73QV9mDPzckoIOl5amvcPJ+JjMeNaHS2SztR0DaxNFdsZiYeO+sVhWeK+NHhRJ/oan0+0TyFhAMCLi81j0cs3V6zGfc6X4GsCfcmjo88YHN5EMn4K9nUeENtRoPlvbS6Ajo/WxCOeYMSgaMCOiMbXLLyccO+hzoK6ccbUdjT5DGdBnMeKO+PHzaoJxsg6xTVc2e2wh0o95ucYfdFTvVuRiol9VsoZkS0Jl5hRSOB3q2xjGddhwBPR3W9NYpC5UVHM+dKwGdKc7wlH3cDk/wnOejOq2tfKT4eNcwsYIZZ+jE3YfFeM4uFNOCk1q3GsHPMqg6V1jf7P0bBBaY2dPye7DYG5je/rbprt205PT8TQVjHgKgzZPetIBevWziBbAsdZFnlEZ+PoAlLwpMb1fCvPP0QwZMCOhgui3gb/uSI6D3nwGvbvFcBkn1APJ0WcWgTW5Leu9KI9qtLhscHP0MOAK6fk4dxBQZqAp5S4ihQXzSoQAAIABJREFUvH8yWpAMwBzojoCewlypWuo5j9wUAeBOoVqvRZm5QxB76vztz6WK5wjoqTKmv3xVo+dMEnga0HYLW6uArkQZl+VqY2Cc9t4zoiC+POCPPKIde5ABzlvunUsSgd05/Eaa6Ro/Z7M1K1je+k/d+I6ArptRB89h4FAGFizxFtkF9CjAk3Txw0NEQFce6MJtP0kgnTkh3wz4w6fqGgsHxxwDNSHvMmboTlHzZlFRfPztE9d1mLPcQTbFgCkBHeBVVhdduHBueLsp2x3czBhwBPTM+NNVu6qppIGIrwB0XRzmZ3cV2d7Fmt/J6l3xInf8jEH/T5+tB7DYRcCVdf7wvbq4zSXOVcs+fXbMylttKKodJPDd8SvCP6utVf9q9jEioANxCVkR9LcvN2F9dXOJF+BGzR6qm9lFs+qntf3DhM0OZuYMGBHQgTvqfOHrnBzomY+PLoSappLrmPjPuvAUDgNPys1iUnBea6dOXAcrtwxoF9AhcUegInxtbrvltD6QGKhs9JRaREtBKMrE7gEooL9lxanC8UBPbtQvX+I90Z2PtSBNuYcYO1mSp36G44Ge3Aj0r1JK9IgX0D8JPEKTZVoF9JqWSaUsbbXRPeB3VoOtNjHqjunect1gy+2mgZtDIC5ePGHY8GF5d4P4MhP4BP4QNl1cNyOsQvVrfQwJ6P9kjk+vL1/3qlZjHTCHgQHKwCWrJo8cFo0vATBFVxeGioBes3jCMTzcegKgE3VxB8aWQHn4GG14DpAxBqqaPM8Q0Sd1NkCEZ0aOjE+4feK6mE5cBys7DJgS0CWhlePxOcEZ67ZlpydOK6ky4AjoqTJmpnxNyPsTlvwtbRHaGC9Y3SgxcXlFpTqThBBrzju7j1nmh8eJYZeZiBJmZvQOj1q5prSQuuz7BGi2iXYZeNHVhYqFc8PvmMA/GNOEgA4gZhP8QZ/+/biyv6bRU8pCPA59507qgOh9SMyoqwg/nQ3enTZSZ8CEgM6ghQFf21WOgJ76eJiqUd3k/QYIv9CJz4zH68vD2tJU6LTNwUqfAe0COkv+e31F5LPpm+TUHGoMDFUBHcAbIk+WL5ra/tpQG/N0+ntp6PyjCjlfefSelU79g+swsEPCLg76176YKp4JD3QG2AKKF/nD61K1ZyiWn7NkTn5RwQfPEvh0Tf3fZXe7JgTnrE55PhzSPoOqW7xfB+tdiKl2GPyilecuXTR11Qea+j3oYRY0l0y2wS1m8uyxBNG3A77wL3UTaUZA5/dEnKY7F7d0j5aDN1AZcAT09EeucsXUsSIWe5KINeZbRWfAHx6WvlVOzWwxMK/J+7Yg6Ls8oQwnbg+URbxOaMtsjaLedkwJ6ADCBVQ4+05fy4d6LXbQdDHgCOi6mMwMp6al5CaW/BsAVmZI+2qvL6DCUlPfvapm763E/H1tgv9+nU5EG2S6tK4ivEwTFzmBqV42sQzCegQEM2sjwo/sTeZzn+8lbyAK6NWNnqnocfjSOQYbLQn/worw8zmZWE6jfTJgQkAHuKHOF6l2BPQ+6c9agZpm780M3Kq3QW4I+CNVejEdtFwzsE9Ar+VasT60pBmg6ZkY5QjombA3NOvqEtBtFieaCKOrRqWm0XscE57S5v3cI4S9IS34g05ejKQmfmVDaaE4Rq4h4PykKvRRqN8J6AybXKI4ML31CR39G+wYlQ3j3eKYEcsJVKKlr4xdUVjn3Fe+5s1M8W7404S8bZ+w/kKCrs4U66D6DMavAuXhb2rGHdRwZaEy1wnovIsZahGrOyIAwNxqbzllanBe0NZJpDEB3aLpi6aHX9Zpq4PlMDBQGXAE9PRHTq2NJfFTRHRc+iiH1IzZm08u1P0+1WifA7WHgerm4ncBcYJOQhhoq/eHS3ViOljZY8CggB4ReXmzncuj2RvLVFsyJaATW+V15WuaU7VnqJavDhVfAxYqFK1LCweE9cKVN8nUd2/+8mlnSDu6DOBTtNh7MAhhlR2PXzSAo1dQTbP3jwzcYGIPq87DBGRpOikN0x2vgSigz2uaNE3AflSzgL6BKO6r861bny6XTj2zDJgQ0Bl4MOALX+YI6GbHLhX06qaSWhDfkkqdI5YlMCTuDpSHdZ8FazPRAUqPgX0HyYkDZu5sYmBaelA9tRwBPRP2hmZdXQK6YOvURRrEr8ONwmWPnXtsQV7BkwzSdlDkCOipzXclmFpjR6wCU3FqNXspzbzdJunpNx7ojoCe0rDesHZC3vZteY+CuDylir0Wpp022WcEfe0bMsVb0OYtsjvwGAA94v5HBnUC5Av428KZ2jjU6lc3ey4EqB7AcN19Z2CbJDEx6Gt9RSe2EQGdeSMJq6zO1+ps1nUOloM1YBmoDI0fYfGIhzO9QLw/AUMlhPuVSyecEHNbTxForMYJELM37xgRnPd8VCOmA2WAgaqQ5y1iOkkvNEUCvrYSxwNdL6vZQjMloEvmdVxozQxOat2arb447aTGQOWyCaMs4VLOBlqFUGbMqi8PP56aNUO39Pwm71WScIcuAd105DN1Bn2c7PwFEf7d0Kh1wbKrAtPXPmQI3yhsVWjSJ4ltFUVtjIGGbCK+JV52yk+DpPcS+JFsvSZUVtDJnYsJqNDVJwaiDPI3+NtU+jztT83jpdOlSy4haPVAdwR07SOlF7C6ubgcEIsBFOpD5qV1vshcR0DXx2imSNUhzy1gqs0U56CzgEB9ebhGJ6aDlXsGHAFd8xgokQ/HDDsNkk4TaqFD4gIifApMY0HsJtAuFbobkiNM/JpwubZYLqz/xLI1m2trITWbMyDgdAnoeTH+l7tmRV430WlHQDfBamqYaoN1PHeqPMPe1Gr2WnqrHRXe4OzURS8jIdwdAT2lYU14oI8ZESKiSSlV7KWwuoHducP18Yf+dfWOTPGuesxzWsxFbdCc042B1XJzeHpwHrR6Omfa34FQv/qx4pPgFsvBOE27vQwbAtUBX/gendimBHSWwlc/o+0FnbY6WA4DA5WBqx47d3jMXfgwGGW6+rA7/2jH2SeccnTtOcFBLQJXPz75Y7BiT4NI58FubFdR/KjFE9d16BoPB8cMA9XNnqcAOlcnOjOeeeWV+IR1Nzo50HXymi2sRASmM1ytBEzQ2ibzEyTsmXW+dZu14jpg2hjYc3n4SUDvOluAZy/yR9SlZOdJgoGakOdGlvR7kL4Q7qIjb9Kii8ylDqteNvECCLESRNovOScuY0kObBDDrm7xtcSToLDfFElcSJKdfwXhShNGEfCaIExf6MtO7vO9fbh48YRhw4e5FoPg19YvQqcVh3/hjHCbNsz9gBY0F5fbEA8DKNCI7wjoGsk0AVXV5J1JhAd1jjsDjQFfWOGyCZsdzNQZMJEDXaV8CPja5qRujVOjPzOwT0BPePNtd6nwSFMyMXioeqCr8NKu0fJLLPhygNQBfbKeyjYYL4Foo3Th+w3TzNyay2RMTddV+Wnj4EcJGJlJWwNQQH9dxlAeNCT6Z8Jlf6zbE8LdXkMgLSHcAWyMctR7X/kTKYfsNiGgQ4lwTgj3pKfenCWn54/KH/MMCGckXekIBQn8YZ0vMlqH11Nl48QZlrCWAhA6bNuDYYPoywFf220aMYcMlDrU3X563v+A+AsmOs3MPz57ZeRmnRfhjAjowPtMdnm9b+2zJnhwMB0GBhoDjoCe/ogtCHlPjDOeJuDo9FEOqRkb5naNuWNK5pfZNNrkQB2GgeomTyOINEUB2tfAm1tjo855fNbj6sK58wwwBpRnYRc61oDpAp2ms8STworPcAR0nazqxXIEdL18potW3VT8fZBQnnR6cqAzXrBlvNRkCPQeL/SO3xDwJRO50AF0SNBMU97J6Y5VX/WqQyVlBH6IObMz0t7aYebfbRTDvpbtiwWmBPQ8Jt9dhqL0KQFdQjzMjoDe17QdVJ+bENAJvOKsFRGfzjOjQUV6DjpT1eS5johU6hN9D/MTdqE1OTiptVMfqIOUawa0e6ADuCvgHzqx/pWQFhNUxYTfgjPPNUTAOpvl13jLqauHSv6/mmXeKbCwhIeYgA7m1/Jknu+uGavfyvWLYCC0X7n0nNFW/og2sB7BFMDbLlfce/e0de+l2n8jAjo4yoCn3h95KlV7hmJ5FSpQWK53CBihp//8XsAf+ZgOrKpG780kcKsOrP0wtrlITLxbc5hwzTb2a7grm0o+HSNeRVrDcPV0mYF7z6bC6lqN3g0mBHQGbXLZVL5wRusz/XqwHOMcBrLEgCOgp0905bLJJ1sUewaCitJHOagmIVZwVOGxd17Q8qE2TAfICANVzZ7bCHSjVnBGRzQWP/2+2amvzbXa4YClxYAxAZ3x1LA894y/Tlu5KS3DnErGGXAEdOMUJ9VAVZP3DiJcoy1fNtGzedGOkrtmPW30UlP18uKT2KbVBN1pQfbQxlhmix2XB33P70yKyH5QqLrJ80cIuhGMfWf2+szireQiX9208NP6MJNDGogC+vxQSYWU8mEQ5SfXy6RKOR7oSdGUu0ImBHSAw+NWzJ1UW1s7JKMP5240e2+5ptn7GQkECJnreftaId6U77ZL/zZ53av9sc+OTekxsO/HuLKh0rKOebMp0xyAxAjUDY1Y/1TV5LlCCPoBMz6pbZHaM447CYjEWVwdLG/9Z3pDO3Bq6RLQLcJJpkIQmQjh7gjoqc3RymUTTrYs1zoAx6RWs5fSTK92s/TeXxHZkiqeCQGdwVHhouJcbGRS7X9/KF/TXHwOM/0DRG4d9qgcb/X+yLiMsRhUE/KqG8pzM8baD4CB58/2hT9VS0Mz1YcOLvds2FtB0BpyVtnGTO1Wp2uWzhCHRgR0ok0uQeULpzsCuo455WAMfAZMCOgAd4477pSjBn0I91DJqQx+mvR6R8WJCk+o87U4oZr7+derurn4K4D4rW4zmfkr9eWR3+nGdfDMM2BKQAfj6by4NeOuWWveN98Lp4V0GHAE9HRY01tnT0TREIDJGpGfso/b4Qme87zRlDSVz413i40j/7w7rPjVGm3fH2qXgPjMIn/rgEgHcOXSCSfE81xrQdByuf9QTuk3tu+kb2Yz9/leGxLr7rxCFQ5dW+okEDpNeqBXNZXMIcgHdJ077eHCEdANfdl1wRoR0JmfGLdy7kRHQNc1SpnjzGv0+oTAQ9DmmJVwrmFi/lKgPOJED818iPoNwj4BvbYWYv00b1OmP2RDQUBXno8ul+s6Zvxcc4jeAyYGg19lyM+OX7G2dTCH+NAloLui8Y/dbchjwRHQc//OqlzmnWIJXq4vtBc/mxfrSutGtSOg534+VLeUXgbbvkfbfGBEAuVhb6Y9U6Jn4Yj4aiJ8KlOs/esz8wP15ZHLdGIORazqZs8fAPqi9r4z3mRY5fXla7TdMjUhoIN4M4Q1MzC99QntHDiADgMDkIHKNaWFVpe9GNAYipoRHXf8ySMHu4BeudxzmmWT8l7SFAkmMYHiJHFiXUV44wCcTkPK5Hmhkgoh+TGQ1nQ16kraax3ubec/NOXFHUOK0EHQWWMCOuRzJEW5817ov5PEEdBzPzaXL51wgivPigiiE7VZQ7JlA4bPyEaY7/krSj12XDZmmtax977TonG+tqv6+2X0OUvm5Bflb72TCPPMnDXTW0LavkUV7a9pmycpAFWGykYI2fEIEU1LoVpfRTsF22WLytdG+iqYzueOgJ4OawO/jgkBnZmfO3vl3HMdAb3/zI+a5snnMOwQwGO1WqXCuEvbZzIFilZ7HbA+GTggHExVk7eZCL4+ax2hwGAX0BMbQ+68E0BVJjylUHcXiL8a8EX+L4U6A6qoLgGdKD7WVG40R0DP/ZSqafb+nIFv6rOEw+N8cyfVUurhc4wI6MxRked4oCczvomIKaPfugUCP0imfDJlWPJj9RWR2cmUPVKZyjWlH7e67HaATsgU66D6/xPwh7+qGXPIwdW0eGpY0t8A5OnsPAM74sT+e32RtbpwHQFdF5MOjsNA7wwoAd3VLVWOyQptPA0RAb2qadIniOLPAFSojTsgDrfrlMCU1e9qxHSgDDAwv9k7QQItmi9QKEs7pcSFDRVh5UnpPAOIAXMCOj9HkhwBvR/PBUdAz/3gVC+bWAbLatSW/1x1SeAO+/2Tb8hGasnKBlhijOc2EF1LMBC2nLGFhJhS52tdn/vR6t0Cg7+tPY0y/7FolP3V2yeui+WCB0MC+q4YcZnOffj+3FQv81wIC/cBeiIf7sF2PNBzMQFTaNOEgA7Cy+OWzxnnCOgpDIThojWhCccwXK1gnK61KUaUwNfUlUfqtOI6YDlj4AABvSbkXZbpAdJgFtCvvs8zJjqK7gDhkmyOGIO6LfDlC33hR4lUNIjB9egS0O2i+FHBieu2mWDHhIDOwCuuLvgWzg2/Y8LmwYRZ2TB+hDhmZDsBmYfY3rN1IHCwzh9J6yKMCQEdQBeTXVzvW/vsYBo7E32pbCgbYY3tvA+MGdrwCXcHfOGrMsXTHVp+rz0s5S31Fe0/zNS+oV6/evnEC2BbSwEcq5cL7pbMlQ3l7Yt14ToCui4mHRyHgd4ZcAT09GdH1epJn6Bo/BmwXgHdZnHqUEhhlT7z/aPmVcs9p8UkVoO1XxhUHazbtSt+3eKL13X0j946ViTDgCkBnQkvxLrj5fcZijSXTN+cMkdmwJSALoELG/zhJQ7/R2ZARRN9car310zQetlaAt8dvyL8s2xFw6xp9J7LAsv079P28Ee0dGu044rHDed0T3u+Mqi62ftzEL6uOUVowiRmdEjYvqAhT+1k+m1CQGfGrrgwJ6BXNXv/FcA9WnMkM95nYZc7Z3/JzJrclDEioANvjFsx5xOOgJ6bMT1cqzesnTBs2zbXw5k6E/fSo7Dtil4cnPbEpv7TY8eSdBk40AM95HmImC5OF0zVG6wCelmozHU8d/4BwPWZ8JN+XeomktV1vsgD6WP0z5rVjZ6pZNEjnGEOxaKiuNvUTUoTArq6fWZL4XMOCfuel1XLvCUQ3EJE+X2XTqIEQ0LQfwZ8bbVJlD6kiBEBnbmDiD11/vbn0rFpKNWpWlZyNiy5nEC6wuywBP13g7/ta5nyWN1cWg5Idftf58MQ9PVAWdtvdIIORazKFReMteJudTBzntb+E2Ik+Ut15ZG/6MJ1BHRdTDo4DgO9M2BEQAfssV2jh/9u7qPdg5n7+cu9Z9g2PUNgPWszRRbDznO7/uWuqavfGszcDYa+VS4tHe1yyyYGztfeH4YN5p/ZW0+5ORuej9rtH6KAjoA+RAcewIIl3iK7gNYBrNWDiyVfXF8RUfmSnecIDFSGio+3WKgLwlr3N8z23Hr/2qXIkhOPyoVubRj5JxCuMTTgXQQ5u87fvtwQfkawC0LeE23GGgAnZQR02MqsHLFuK3rZ/urtN+bG+1yZ9flVk0d2ROPqUswUXX0ckAI68L5lxSsWTl/3jC4eHBy9DJgQ0Jnx7tn+OSelEwVVb+8ctL0MqOimNObt/xXEXzDAig2ild1uUXX/5DXvG8B3ILPIwAECenWzN6AhNHl9wB+uzmIfstEU1TR6F7DAXdlo7AhtvGS73FOC01YOqtsrNc2l0xlSbYwyyaEYC/jDblPj4wjopphNDreq2Xvz7lBetyZXOqlSNgFVdf7wvUmVPqiQI6Cnw5qeOuqG/fPTvN8TwC0aQ9TZEvhBgz/8k4ysVLfGQ97P7d706k65wcz0b/XlbX/MyD6nMpRYJrrt+kwvCx5CpbqUQ/SDgL/tv3TR7Ajouph0cBwGemcg8U7okg8RdIZwZzm2e8ywoSCgyzieBUHf+pthC1ifWFS+5k1n3vZvBm5YOyFv+w7XIjCuMGRpDEQ/HNt59C8G+3fJEH9ZhzUmoIPW57li/runrXsv651yGkyKgUtWTR5ZEJXtAvKspCokWcgR0JMjqifENKmz3EzO0w5ojMHdluTx2c6VXdPk8TOROhvUmR5mb9+UiPyHgC/8FRBkcuxmp1QiRd0xb/0awFcMtbiBLfLXT297wRB+UrCJyzb5WALC5KQqJFHItIBe3VR6GUgGNZ49qV45AnoSY5vLIiYEdADvjvM5Anoux/VwbVc1em4kQbcZsovB/IAds74VnNX6arYupBnqy5CGPdADvdl7BwHq8D+T576AP/yZTAD6W92qluJikkLleBuWom1qgdYB8OvE4gW1CGXCaGKcD4LynEwjBystH+dr89f2swVfirwcUFyTgL494A+PysSOI9V1BHRTzPaNO+flOfmj3t6qbuJ+uu/SSZZg7haugk8tmr7i5SRrHFDMEdDTYU1PnepQyanMvIL03sxWOcC+EPCH78jISgZVhbw/0HzZQ5kkJXB9Q6b2ZdS5QVI5MUaeXxIo42gDBzBCKioefl3vC39DF1OOgK6LSQfHYaB3BpTg08mdDxAwSxtPPDQEdBUNhix+EtAooAM2s3VWffmaV7WNhwNkjIGqJs+Xieh3xhoAdUvwYy62f3zmyovWOiEvzTGtA9mUgA7gJbhdvsCU1e/qsNPB0M+AI6Dr5zRZxMqG8W5rTNEDIJ6TbJ0ky71DFL+gzrduc5LltRRLXM7abt0J0HwtgIeCfMBkT+tvobOrl5deAFuGAJg502T8uWhU/N9MRexMdqyMCOjATjBNry9v+0eydqRSrjo06QrIeP3uS30ilXp9lHUEdI1kmoByBHQTrPZPzMpm7wSLsQqEAmMWSn6ZQf/ttjv/dtesp1WKqkGXntkYd/0E+EAP9FDJ78D85cxso/sC/rZBI6DXhspc62VnHSil2/UbwdwOG7cGZkbW9sInVTV7v0iEGkh4UvHeYJJX1vvaF2Y2Tv2nthYBnfF+oDx8nKleGRHQ1WFA3OULzHQOA3obN3UTVxzz+rVg63ek08OJ+D3byj8v3WgOlWtKR1tdditAZ+qbc7zLcpFn4bTw8/owBxeS2lBv2+H6CXEiL5jOp0NAXL7I3/pYJqDKO379dM/vwaQ7/I8E45pAeTjXUVAyoaff1J3X5KkRRIt0G8SMv9aXhz+vC9eIgA7eAsuaEZje+oQuOx0ch4GBzMCcJafnFxWMfpBAWgV0e4s1IjivtXMgc9OX7QtWeMfbcah3iT4PdMC2SYwL+lpf6at95/PcM1DV6DmTiJ4yeuCkusmICgu3xeN4QFj0el60Y9NdMxOHT3A8OXI/D/Za4Ajo/Wcssm2JI6Bnm/E97fVcDL6KQCqFVBrOOUewmxGxt+yYGpz3fDTbvatq9pxHIJUS7RgjbTPu2ta95fpH577Sb1LtVDd7fgzQdwzlPo8y5IyG8vYVRvhMAdSEgA7GdhfxtLv9kadSMCXpoo6AnjRVg6qgGQGd37M37zw1F+/VQTU4mjtTuWzCKGG5mgiYoBn6EDgGXgHL/4qzWCei0few8/StwcqgdPYzppnPHP9AD/RGz89I0Lcygx1cAnrlstJPWZZsS9r7nBGxGJ9bWJGcCJbI87NxxBVg+hsIriS5b7E3n1wxWHLCaRHQgTcC/vBpSfKXcjETAjoxvxgXXBb0tW9I2aAhUqEqNOmTxLZa6B+ts8sMflLSsKlBX8vOdHAvDZ1/VL50txGRtjB1BOwAxT11vnXr07FpKNSpDnkmMpPyFPy45v5uIMiKTPPPl4XKXMej824wqjTbJ5mwoN4XVqH5nCdDBhY0Th1vi+iz2g8oGPcEysOVGZq3r7oJAZ2BDyDlrPqK9vak7GTQJQ9NHpF/tCiA3WXZ3fKAdWNSGDku5B4hyX1Yu4fBHi4puiO+z5vAKpTxYejYcceUF3fk2Gyn+SwxYEpAdzOO/XtFZEuWupGTZmoaveeygHqX6BXQ4Ton6F/9Yk465TSaEgM3LZmTvzl/62om8wdOewxT63blDbmRCS8IRjsRvRSz+d1hhfHujp2WjLkF58VjidC8hcPdNjp7dPadMVfK4XpH5H30+9AXMbYooGi8p7zb5ZKW7DrAsySaLzi6U3C+yyW77M6ez6wCO9rllsOGu2JHj9zRnWvPwL762Nfn/UVAr2w4sRBjTxhlwSoQti2kZcmuLiDPFZN7x0bmuWRHB7B3PArceTJvmMXxqFt2xHdyXtxifAC4R7h4R9GHB4zl8R+MsPePJV8wxs07P9x02Pl1+hln4I3171v7uDsBoK6dif9WymD39qMomhenvIKoiO0aQYVH29TZHRNxq5BoR7el1jDY5bKirriwXHlCxG1huezEPBNScPdOu7PrBNcHiyeu65noOXocAT03xKvIbGB+EMC52i1g3BkoD2canTQts1R6HavLVl7o89IC6KMSAzuI5exAebuKcpjzp3Jp6WjLLdcBOFW7MT1vr7vHdo++rj+kQ1nQ5i2yO6ByoGsL4e4I6NpnjQOochs3eWcSQb1ftXklE/BePhX+y52+lq5kSJ7z8un5I94bWYRYgdtyxYUdlWS5Bbvj0UPWHHvXmb3hWrbguKs75bXwkewssAqpe8/aN5n+HKmMWo+JEeg8Y+mcnbmIOFXTWPx1JvFzEHRGmuityzGAPwTofWb8c/dF/lZB9tPdtuv1Qhd2dEdtOyYFq3WrAti7dlU38+009jNuIcltJX+OF427ha3qHG4/YwuOyo/2M65Ciwvz82R8U6fceVRRPLa9KPro3EfVxbtB52F/oAd6o+e7EPTjzCb+4BHQe7wJSx4B8+wkOFECx5/rfeG0PA9rGr3HMWElCGf03RZLctEFddPCT/ddtv+X0CGgE9Ezdb42/RuHPfQpAT0/r+BJgE7QxagS0FWoobqK8EZdmIMJJ3GonT/6f4noOu39Ynq4aFTs8nQPqirXThhlbXepizXjdNmmBPRoLO65d5YjoB+O06tWTzo21mXfA8JUXZzvxWHiZyWGlaZ7oWIvjroQJTaOvJ+AuVptZEgGV9eXR1TuLefJkIELH55y9MhhMZW+YUyGUAdV56UBf0SNvZbFogkBHcAHNtuzg+VrI0fqu7qFS8KaKwRdxcyfIIgRBHb12MVwAAAgAElEQVQx8YAT0FmSOqg+vN3ERNwTjk8NGhHHmLGFIJoE27/MRt5H5YkRz6fTE3lDiU5liUJYtAvANgH+kCE+ZHAXxTkugTi5OC7AcZspMc/cMZd9wFi6gSjHLYuYYmxbVtxFwpLCFpawpRSCLFKfsc0EFpa6uklSir1jS0IIlkI1lYBVRVkIuec/QZbNIJFoMyZVdgnAEkJa0k78u20RW1LIGFRmjJ5HCEtYqj2Vj0LNIdUuk5X407ItCZFPQgyHLUdACDdYMkCbLaZ3WERfO2v5uq21tWZyVZoS0IuEfdztWQ57qvd91jdaZaj4fIuFepfo9HqzicQn63ytzmXCvoegX5SoCXmvZMadmnODptQ3BtSLJc6MGBGplCqSGAxSKQESUEyCD3xX9tmCUK+iZC+3q5elUD8pPY2xVP/Yvwmi3bHpiJmk+odKzaP+IPWiVap7VEp0EvFWEuI1lvwig56DW74rumnDzs74jsUXrevs794pORbQaf7K4tNkzLoW4AsBHA/mEUxwJXhOTASyiVjKnh88CcESkiQJSGbY6CkkQaSGJ/GblhjExGcfPaRw9lvrqclHOLDMvtKUyLS8T0BXP24M/khQJ1i7Uz+pySJINcywQGypfyewxUyW+hOCLGb197DUj3Di9zkxlWi7tPEuW3RvQT795e+lre/mYp44AnqfLxTtBcpCZQXHc+f/gHGtgQN/ZhJfqfe1/q92w5MErFrunUk2VC50nWuMva3vzYV+Uy6+L/tTUMmVlgi9+V/E9A0D46ia2mBLlAeTdPBKcnjSLnZNqOyoTu58lICStEEOruh4oGuj0gH6iIFcCugqZWg301WweAGBPsbgQlJ7Z7UkUW8vAfvgEye1vkmsOHp7mNRnWs6pDlgSgUUvJy0pTScGqT7tFMAbDNTlxTofvmvm05uy9Y6e3zTpFJvs1QactVLjgTlOROogRa1NlerNDLUmTewr0tjP7KnFyV8MIDXDetare9vd99uZ2GapebZvPjFLJluQOi3iKECdTNgugDcZ9CJBPsvAO1HQBgs7Pjyn7PmOgZqS+kAPdC15zAaPgF712MRPktt6OrH97uth3DMs3/X5O6asTttraX6o+HzJQoV8/2hT1Vu7hJaAL+zry6yB8HlCQGf7ERANT99eWhPwt+m7xXiQIZUrLhgrYnlriejk9G08sKYjoPfOpArdbo156//tPhW4lYBhujjfi0OE355VFv5aui9uR0DXPSJHxqsMlY1w2Z2/Z8KVZjaWXBfwRzLOs1bZUFroOkYuZWCaVobUwon5M3UVkQe04g5RsKsemzk8mrdtBQGf1ksBLQ/42ny6Fvm5EtDV903YnXeSgDqA1nbjWi/X5tEY1DR8xweX3fGv5rzRr2467+NR5N8Gokl7Iq0cbr2pNktqE2Vzj9CiBKGEEJOYaz2bl4MedTifEHKEKqAuCZAAsVQb3MTf9/xfbaR6WkxsknpAWChJfT/AxGYt8T/1JLSpfZ8rkSFxig/es2FXqEqAOPARe9fS6rR/z6bM2tO+WvMebt2rcLZDbbwk/aSuvPVBXd+t/U1zBPT0v0tGBHRmGSM+915/+3PpW+bUzCYDlz026dj8PLkcYG2XSrNpf79tS71TCVvB2AR1mEh4logbikbaT6V7Adh0X40J6IyXbQhfsLz1n731obq5xMuQdxBIzcNseA+ZpjM1/MRlWzwjori6bk72nSwcAT214cq09E0vz8nf+PYHNxPLrxNRfqZ4h6m/TbJ1SUP5mpyF/N7jhX4felLs9H0emzoJ71kEz0Jf+J3Uq+qrUbWs5GwSrHLvjtaH+hESAffGj9sxP3hO9kPxH64/joC+hxXiTQI8c5Gv/UkT4+5gZs5AVdPEOYC4X+c7NhkPdJUu1NXNtzHzZUDSUYoz73D/QoiDaTkErgv42t7IhmlqDduNzr8yozob7Q2hNtQFgG0kaRMEvyUlvSiEuK+A3lt9p++NpCIx9BeuDliI1ISKr2QWGeZYHTwCenXI+w0wftHXYDHhmXpfWIv387xm71wBVgvFvhfCRKdl62XSFweZfF7T5PEzaDEofaGUwI/V+SPJRApIy9TKJReMFYXuCLG+sEqOgN7LUDCoOuSpYcYdOhcr+7fGwI31/vDtaU0GAI6Ani5zqddTYb7iu+hbRPgGwH2/F1Nvgpn4hnpfROWOy+i56rFzh0fzCpdrz53DLKUlLm0oa1uckYFO5QQDlQ3j3a6xI+5hpou1UsJoDfjDk3WJfDkR0Bk0L+T5dwH6tVZuBiZYjKSYV1fRauTiihKd3K7440R03sCkJ4tWE7pknC9tmBF5THerjoCePqNVTSWfJmIVjUefd5gSgeL2efWz1qo0G84zABi44U8T8radYf2OQDcOAHMHuonKE3otGPfA5pZdo+3ncx26e39CjQnoKl+kJf2B6e1vH24AVSqB9/O33EVE2tLoDNCJwiD5q0BZ+7d0rUWT5UFdvrRkZwSEs5Otk0w5lnxxfUVEeSE7zx4G5iyZk19UsPVaAfyUgZEmiGHwc5K4ItepBhe0TCq2pb0UMCMuA3yHvXnnF3OZj7i6yfufIHzPyCUBRheBL6wrjzSbmCfpYDoC+j7WNruYZt1d3vaPdHh06phnQGkzBL6PktFmkjaHNxTtsj9x+8W9p12pafZ+hgGlyxUmDTsoC7LcHQHxR3X+9luy1b35TaWVErIeKnqQ85hkQDlfvAjBAdhotkbQUwu94R3ZXrum2sEDQ7g3l1wCJHLopP0w8+L68sglaQP0k4qJXLbcsTCJvDs2iNStGBW6TstT1eS9nwiX9gUmmec3lEfq+irX3z/XIaAzUbDe12YkR5Liz4SAvjtP8gt2nnt6cNpK5VngPAD2fO/mAfSrROg9E4+KMAJRXJ/BYtWEgK7C9XIee+qnRl4y0e2BiDl/5ZSjZSz2UwCfN3j7cptliakLp7c+kylHCc9d7gwTMD5TrAPqqzlriYvqy9oe1Yo7VMEYVBXy3kbADVopIGoPlLV5dS38ciGgq0Pojflb7hZEV2jlZqCCEd8f8EUuN2F+dfPESxiiQe+m3ISl/QNTSrl0R/SDSx+d+0q3ToscAT19NqtaiotJitWOgJ4+h4OlZk2j91wQr+GMookNFjay0g8lpG+T4FaGvB0FecuCk1pVasScPrkS0BeEvCdKRuPukJ9n5ZSA/tH4G9u6Ro97dO6jWn8r++paZWj8CMFFrQT+ZF9lU/ncEdAPZEvxbMmibzPx18mkuMK0yD5+++dy7bWc8ELvlAEQTJ0vf2ADM4L+sMo/nvXn4rUThg3f4VKXorRePNnXEeL7t3WOqcn2++BIRDoC+j52HAE969+41Bo0I6Bjo9WFMxfODW/vzZqqZs9fCHRtatYO2tKrNlChr8XX0pNbzvCjnLfsXfwQiKYbbsqB72FARS3cCeAfDP5rN0UfesD35If9lZyDc6BPhaDMwvQwPxwoj+j16soBezesnTBs23aXCvE6oY/mt7iH81l/90a26DJzfshbLYFFSYSO/8+AP3yzrnZzhaNDQAf4jwF/5Eum+mBCQCfgeVB8et0gz5GZ7Jh8ftXkkR3dceW98hOQuVA1zPyiFMMmZpLv2oSAzuAPrTh5Fs0Mq9zMQ/pJ5AJrfPtMCP4lCHNUbkBjhDA/YW85pTg4L5hibsxDLVJ5oy3L+gdA/6LZXtuWmBOsCC/TjDtk4aqaPT8j0De13vYfBAK6iqIQzyu4l3vCJToPsLno5fjHbr9x3UcJvTWxUtXo/SoRfm0mLYUmI/sVDL9lk1Ue9LW+otMsIwI6WNoxnB6cFXldp639DcsR0PvbiOTWnpqQ9ydgfEvlcs6tJUOsdZXSQ2ANiL/XsS3vyYf+Nf10cpkylysBvSY0YZxkV4hMXb7OlJhs1k8kWd91fF3Fsxuz2awjoJtlu5Yh1reUnAzmH4DxWVASKR/TN0ll26mu87c1pA+hr+b8xonVUoi/AeTWh7oPSSUi+mW9P/wtA9hHhOxJWfjGd0BWLaBSL+l9GNghAV+uLgf01htHQN/HjCOg653y2tEqG0tnW0Lerzml3REF9NpaiBenedcxcL72Dg1EQKJnrUKevLCk9wsHurs1v6l4moS431RaDd32DhY8VvndgaeZ+Puy21oTnN36QSKNYD96DhAlKld4x1txZJZzbpAI6AkhRLheAuHYI44X0Z0BX9vndI5p9eOTPwZXXIUo6+sA4q5xK8LX1NYekmtSpznGsfQI6Lg54A//pyljHQHdFLM9uPOXe8+QMdwBkchJrD3n+X7Wq/fyT8atiPwgk++NI6Cbmw8qFOj2M6wvA/h3gE7SKnAearYE+AcBf+S/dPQokQfUFW8H0ck68PbDsIXAjEVl4ZBm3CELV93s+S5AP+wl93J6vAwCAf2GxROGbRvuChIwNz0SBlst2gm3dVZgyup3dfesuslzy+5s4LW6cQcx3na2Mat+RliFDNf2mBLQhXSfsahi1WvaDO2HQOYEdOu8+llrnBDu/XDMj2RS5bIJJ1vCtQyEMweY6YPDXMZ2Im5lEl/IVYo3dQkvlleoolLoTk1yxBDuVY2eM4lECMQfGxyDmVkvCohOuzNLOUP3WuoI6JmN2RHfrQ2Vlhjz1tUk8B0wf2L3/qWvM8JMjVkv8vImLZq6Sh1e5/xJhKzP3/IQEc00ZMybiEcnBWY+oX2tfyR7e95b1GpQpKkb2zX6c7/LcjSKvsaocmnpaJFnLyWi4r7KJv05Y7uLeNrd/shTSddJoWB1aNIVkPF6kNbv3hbEeXZgZmRtCqY4RbPIQFWTdyYRVITmAo3NHlFAn//wlKPtwviTRKz7PFFjF7IHxYQn3dHOKXfNenpXtlqtfG6823p/5P+BcWW22nTa+YgBZu4gon/Asr8SmL72if7EzYECeqj4eIvFexkZOFgE9BUXjLXibsXFEW8DMtPc+nL9YXWrmz2v9unFSFhml508J0iZe05mNOYZVtYioAu6IVDW9ucMTem1uiOg62e2trZWrPc98nGyqZohvrw7B5XxRQKBP4yTyxf0rXkykx45Anom7B1aN3HTcvqE0eA8P4NvYvBk2q0s6W3lsGg7pYxf0lCxToswfXno3BPdXKjEnY9rtZ0QgxRlgfLWNVpxhzBYVcj772D8gqAx2gVTe8Df/0O4s5Cz6sva2w83/D1i4pi/EVA1hKfH/l3vYrKL633a8zFTdbP3VgA/cHhOjgFm7iYpZwdmrG1JrkZypRwBPTmeDlfKiIAOSEFywiJfe0brtPR75dTMgAGqbim5DlL+CdlZw2Vg6qCuupGYfjxyVOz/bp/Ye45NEwwkwkvzSCWgn6sXn1+1bdsfnLHurcPhXh7ynpjH3EQg5/KGIsgd/XhgSnbFQEdA1zzjGbRg1ZSj4t3R6YJIRVn0G/Y67+kAwybCDxb5wj8l6j+eXzUt3hlsQ3kEDtfLdKLPkoHfjBoV/87tE/VHnOrN3qrGkq+RirZnINJejycfXRrwtz2kna8MAS9r9IzJB5ZC0MQMoT6qPhAFdMZWEM0N+NvC2nhwgLQyYERAZ7xvy/iZwRnrth3O2OpQyamQ3AbCcVo7M1DBCC11ZWF/tn+Pqpo954FpCRGci5m5mjuM7RD0azuffhec1Lo1V2bs3+4BAsUNayfkbd/uimZk2CAR0BMesTb6zEVskzwh6GvfkBFnh6lcEyppYObKPnDDG6hwSrbyQeju4148HQI6CbqkrqxtsSkbHQFdH7NKLH2hdOJ4kWddzT1ejipfdDbEUtWJVfbmHeXBec9n9J5zBHQN84FBN6yb4Nq+w1KLk8uZuYKIVKiiPA3oyUI8RxLldRVhLWEOrwmVnNrF3Ar9ISQ7BTB1UY7ysyVL5kAqV9VU/EUi8Vu9843XBHyRKf05Bzozb7WIZvY2lxIhIkOe/wXoiwNpPA3aGoPg8kBZZKXuNmpCnu8x04904w5ivC5pyVkN09szSzV1EEGOgJ7+jKkKeUuIocZD5++2I6CnPyQ5r1kZKhshuPP3BFyVxbV9zvvd7wxgdJGgxd2y+5v3lT/xZrbsMyeg43UQ+XvzrFeRA4Wwlmn1aMwWabrbYdgbxI6jWnzPq5ySWXscAV0D1QyqRZn1wqqOcyjGn4EQFZC4QLP3Y1+Gvg/Lnt3fvL5UdIuoq6CBiExFyNpOElPrKsJP90WQjs8vfaLsqIIPO9eYyn3O4Mck7bwimOX3QDLcOAL6HpYcAT2Z6ZLTMiYEdAZvypc4++8Vh08BrIRbAinnnqNz2vn+0Li6xMX094C/7ZocmEOVzaU1LpK3MWNkDtp3muxhIEYSy+HG1+umhp/Rdc6aLrmHiFZVTd5tu3MyFqULiEEioFctnXIeuWN9ej/Ym8Ww4LzWzrT56qViTbP39wwcMac3A+tGvRwvNZGbU3d/joSXuFEqE6FRCtNslxGj0sAsc7f3TAjoAD/nlpje249nmlz0p2qJe8uVraUF1o74MZyfdzzJ+BlgXAGiC9X9+OwbK28O+Np/lOmL14SADuADZqu4vnzNq0nzwqDKYKXYOWKnS9UZsfNN3jT2WFm2vEXW3nLQrXHdVxSOkI2k9lZQy/QycebIHfTBa24Xjo664RKFgGuEsONHw6LjCOIcdUmIevL7aM/71SeHDAmWtYGKdm2pH6pWT/gEdbnW9Jn6o0/jDimwnSAn1fnbM0uxknq7g7ZGdchzLZj+qFP4YeYV9f5IWabvl72kX/Lg5JHDi+IbmdP+bTx0/Ig3Q1gzA9Nbew2HVNXk/Q8i/GTQDn4KHWOo1Ih0Wb0/rNYoWp/qkPcbYPzcEZmSpJWwi4DZdb7wqiRrJFXMEdCToumwhaqbSidByBawI6Cnz+Lgq6nCs1p5diOIlPDjPDllgNcycF29L/K0rrXJkbqTKwFdOYHs2G49xKDZOaW7XzTOm8Z2jTkp22GbewT0Ea0E+qROGljyxfUVkYd1Yu6HpW93nGSWzrKWMutTnYXWVmzNl24Uxi0xUu2NSdCxIDobTMqBZoKJnNjJccgN27rGXP1oPwv7rWyvDpVUgXmhoXMDCaJbAmVtP87Gu3J+yPtVKfFrUJ8pO5Mbtv1KMfhDITC3riyiLvX3u8cR0PcMiSOg97u5ebBBuRDQa0LeKSx5KYj0R9vo94wfYiDD5h8GZkRykvJOrS23bbN+TUT/5pzX5Hry8KtS0PX8/skrgvNyF4H7kEVjdbP3ZQCnp0sPA4/V+8MDfvMyEAR0AP8oejleMtAF9MQPk8ADSFckYESJUWzyxqgJAZ3Bz1p5ndMWTX0mqfxSVz117nB7Y+HHpItGE8ueH1RBUWaKwYpHKWbZggVLOy5loSXVn3lxSwLd+77OtmXJGItet3h5JMmy7QPyagmXS9jEiXeFsF1CxiXJfCZhW4lycUsKKZjy48gTlsi32RoO2z6OLXESS/scInEawMcwaAwhEXZLp6dS0q8qFb4dkqbrmCdGBHTC5jyR77lr+orX++rUgjZvkd3BU8G4CETjQMhP1GFSEbskQYnDsCUhSpDdBBFj5igIUUjYB+MTQUqmKImPRHcGXCR531iRIGJwPnbfjt9T32KQG8RERG5wYo6o8i4Vfp2R2BCqv3OBE+XUBZkRidBrPd91ZbO+g4u+SDv4c8ZLJOKT63zrNqdatbfyVY1TzoQVX0XMY3Vh9uDwFmaXN6XLFXoNGHRo1aGSa8D8J50XedRt/3p/ZE4iGKCGJ1cCes0y72fYwj0aujAoIIQtPrtoRuvfdXemurnkKwD/Rv206sYepHi7LNCshf42FR5Y26ME9FGFY+4HQ313NT0smeR5BkL/a7JPD4wjoOvhcdChMGj+ytJiGbcfAeiYQde/gdUhtWp/h2xcW1cebjQtDOVKQC8LlbmO58470BP5YGg/jJfs43d8KnhOZtHWUiUx4SHsHraSmLVenElFQFeH3Zs/FCflE53KFn2cmYeRLfLZYkKc3XCRRerCl0QeqVzGxG5W35DEKowK9uxl92y92JIM9yELNLXnBR9y8ZsgXBJ79s3MCj2xN1e3MAmUn4ionXhI1VUX390gFKLHs02dj+R8b8yMrZYtyxfN7J8pVBYs8RbZBYmoN+elOj+TKU/Aa6BCb52vRdvZwOHaVSGad0feW0W6U77tbYzp4QJRUHmnr6UrmX5nu4wjoO9jfCvghHDP9vxLpb1cCOjzmr1zBfBArs7MU+HHeFlmdbHp+oA/rNZ3OXlqGr3HSQGV3nCGc2aTkyHY98sGpk1M8pty8ykLcyWiHyJe1DR7VzIwJW1qCMsCvvDMtOv3k4qOgJ69gchYQAe2s00l9TPaXjBltTEBvaNz2qKLjiygV664YKyIua8F4QYwjlObLTqc8MgsOaFmsmSGJCIJJaTu/yTc6ZRHXW+P0kIPEjUZatvZs+VjFiD1hyomRcIM9a+5FEKTG3TV6T/WrwjfhNqDOEmu/gGlVKhAy3KpXNfj0qjeW5XNedaRBXQVXvm5kPcCi/EHJkygXHhua+xwzqBYXSLgHwX8kVt1HibWhErHgaX6DdV9YPyem0Xx38tb/5kzzgZZwzWhks8y8+1aBXTGA/Xl4ct0UZUrAX1+i9cnJZp19WOg4zDzTfXlkd/ruhixl4+qZu+XCPgfQ540A532Q+wnYAckz6qr0OtRU9kw3m0dM/I+ACoyjqaHJbnogrpp2QkFqsnolGEcAT1lyoZUhT05a+82EJVnSPGopbOE1xmYX18WDutc9x5sW64E9MqGSss65q0/ALhBC18DGERd0Jebd07INF1ZqhTcsHbCsG3b81YS+NOp1j1S+WQEdJUe7qVpnhkS4mZm/hSRE241jTGwJfMvG/yR75h8R6Rh1wFV5jcVT5OgJaa8M4nox2eVtd1cS5mfF/XW13lNnm8LIhXpS7sjAQNxIqsmULbm3v46jo6A3jMzGPjQBbpI98XgTL9jTv2PGDAhoAN4v8iOn3l7LznQ5zd6q20Li+gjp6WhOyTKUZIwv84fvjeXJFzd6BkTsyjIQNl+zmS5NGkIt02bBPj6d6nwkVyksj6cgH4PA59Je0QcAT1t6vavmEwId8cDvYcxAt6Pk5gc9LW+ooX8w4DkSkCfv3LK0TIWvQsgdSklJ57bpjjNJi4zv0VRMT0wp+0NHe1esmryyOHdsTYmUvnbdT19CuiVodLTLSkfAuFsXY0OSRzGuy4hpt+t+Z1hTkDnt4nsT+v0lh+S475fp2tavJ9jidt0CugA7gr4w1fr4jZXAnpVo+cilTtVVz8GPA7jmwF/+Fe6D6Kqmjw3Eei/TYRvHPCcH74DXZLlrIZy/TnQRxWMfgBaQ/+ytCzr/IXTW58ZpGOR6JYjoA/m0c28b+rS5/rQxIsI4h4GOXuYzCnNDIH5LZFnVS6a1hrJDKj32uYEdH7Vtm1/cMa6tw7XesIDXXb+FYQrTfVtwOAS3rCPPfms4DnBaDZtzqWAPq+p5HpB8r+ciBfpjzgTXrRccu6iqe2vpY9ivmZlqGyExZ0qrZLfTGu8QUievKjCGA9U1eRdSwStF032cUFos1E4I+hr2WmGn8xRHQG9h0NHQM98LplGMCSgb7S6cObCueHth9jPoKrQxOsJloqS6DxADERXBXxt/5+9L4GPsjrXf97zfTNZIEE2d8FbteJSlRuSSUAgkwQQ3K0hCS5F26rdbrdb6+21bbS1/d8u1672iq1aFzJhqq2igJJkWJNMIO671gXqgoDIlmVmvvP+OZOgKAmZ5XyZmeT7fj9/AjnnOe/7nJOZ853nvO9bn2IyqDJQcoIhrb+CaGqKbXGGB2+DpK/4KoKDnjHz4BroTUW3EegrCc+KI6AnTN2BHR0BPS4aNws2pi8ub34rrl5xNI4K6NnuNgKOj6PbIZtGU7gfIgJdpRSrCRR+AxC/1TXmsMVh/HTS2uCPazVEnysO1QGRKUcGB1tAr2oqupIgFkGlUneeRBkIE+iGyLbjfqs79Yt9KdzxZr4VOau/m6qJEjGc+1U1ea4h4A96LybJP/rKNnxdF68pE9B7IqNVxLXzqAMO5hvry9p+pltAn9/g+S4J/LLPjDIO830xECaDz6mb2aY1O4JTAz3xxeYI6IlzN5x6VgeKSwFeBMZJw8nvtPRV8pPdlnnO3+c0v2+HffYJ6HgNhizzzdywuS+7VSYRMWbkwyRojh1+ZRjmzmzKOXKw0zenSkCvfnzy0TBcLSCakGHzlE7mdoDoa77S1r/q3uva4CRVrfIsFBb+zDbUD4/qmkQ3+7yt2mvuqkwJL80svgbMKvuUHZfK9ljMF/rL9e6Tdc+hI6D3MOoI6LpXln68VAjo1YGibwH0v/q9yUhEVQXlW76yVvWZmfLnivVTDw93WosgcL6Tzj3F08F41Yqgxj8n2D6YlvQhoHt+tO8w76aEjXAE9ISpO7CjI6DHTiMDL4fNiPfBGe3vxt4rvpYqbUe3oFYCToyvZ/+tmfFsjuiecbf3qQ/7PAxoLskxumQdgAt1jTkccaLR50LM9Hn1RJ8rDu0T0Lno3pltfdZA77lQUfxTgH8wHOdRk8/MTI/m2FQXrGr91BOoy2rWn66U/+kKd51575xn9mriYdjDVDUWfR1Et1JPHUIdj6qO8QtfedsNOsB6PmdKRwrZuYUIubowQbwNwpjtm9nyZJ+YDKpe5fkFGP+pbcxMB2Ku9ZW13az7UHF+k+e/BPCzTKdnEO2PSElzl1S0Nugc0xHQE2fTEdAT525Y9VTfKw0lZ8G0fgtWZeKipZ+cJxUMMKQU9FDXLuMLD1+4frduE1IloF+9blpeRyiyEoBHt08Zh8eQe0dF8pZOae8YTNtTJaBXBTzFJNEInXvlwSQu1WMxJAv8bgtyvpeKdKiJuH9Zqyc/0sGNBJqSSP+B+qggl063a+rDZ+v9jLwk4DnWJdFKhGMGsiGhnxOW790TuXTp+YP7u4RwyHYAACAASURBVB+vrZUrSsYYLmsFiArj7dtve8Yuk3jGfWVtT2vDPACoOjD1UshI/b7LFUIXviOg62LSPpzBFtCjl2yme74Dwi/t8yqjkJWA/htfWet30sXqmkDBOIb5fTB/BaAR6WLXcLSDgfWS5KV+74b3Bsv/g15gqxsLvwxSEY4JPo6AniBxn+w2nAT0BU1FcyToHwCyEyGPgHZyuWYtnr5uRyL9Y+nTK6CvJ+DkWNrH2ObpXLc5/c5+NucLA6WHdXLHowQnTUiMfPbV7ANBVLXYq/fA3Q4BnYEtZMjCfqMruNIwV23+P2b+UhJ8DPeu74LFpb7ylmY7iFgYKD6+i7kFwJGa8V95j3LOXOVd1aUZd9jCzW8qul6AlHhpaCGBWZLAj+q8bbdowQNwxWOzR4RdH24GaLQuzIEE9N40qHeAsFDbmJkOxPKHvrINt2gX0Bs8NwuBH2Y6PYNofxiQc31lGxp1jukI6Imz6QjoiXM3HHtWNpfkiC7rVgLNB6Dve204kpmkz0T4dmRr8Pf++bCShPpE91QJ6JWBwiOFpEbSW1pLJzWDikX5kfF1U9q3DeagqRLQ568smkMGHiKQk50tgQknYA0k5tdVBLck0D1lXeav9HxeGLgH0HjJ+ABvCPJrkW0bbtf5GVnVWPwVIr7NFtLURQjJV9XPalOcpPXTc8bZuZyAYm2GOgK6NiodoI8ZGGwBXQVMVTcWfZsE/dqZh48YqPOVBRekEx9qnhY0eRYy4UcAjtN2nphOTmaILcz8pxyR+53Byrp0kIC+oMkzTwKPJswXo8lXHixPuH+adKxacfaZ5A4/NZA51jaR65/f0jlQu3h/PpwE9KrG4rkg+ffEX3x4tZVtzPVP1T8P++ctFQJ6ZXPJGKNLLgdQFO/6cdoDTGBI/O8pZcHrawlSJyepEtCNwKY/AfiyTl+GDRZzCKAvvSdy6uy6YV/ZeOYxgrKDBO03yx0BXfNCrWrw3ETEN2q8SR4B6Ls6U0ylQkCfu2xu1qjsHfcArAQO5wFAjG/XlQdVKRXWSUh1g+eHELhZJ+YQx9oF0GxfWWtQp5+OgJ44m46Anjh3w7WnEtHRYZ1lEH6rNfpsuBKauN/vUFiU181peSlxiIN7pkpAX9A4daIk2QjwCTr9yVSsbJJH3T2IETmKp1QJ6DUNnlks8HCigRiZOsea7H5TGJi9eGbwNd17XE329QtzwbppebkhaynAM20Zi/E+w5haX978Tx34CwPHZ3fh8CYwlejA+zQGA63d1D33H/1ktrRjzEQxHQG9hzknAj3RFTR4/WwS0N87Klt85tZ+tIuaRs9VTLhz8LxM95F4ha+sbW66WVm5pNJwjXlzohTiv8G4EqQtq2W6uZre9jA+kIKqlmgOmOzP6YME9MqGohLDoNXgROuy8GpfWVtperM8sHWOgD4wR7paJC2gMz0yaW3rhbrqW/flVyoE9IsCZx2WLbMeAWGaLq6HDQ6zZEKDDBk1/nNaPtDtdyoE9GgK9ybPL0BOauW455MRYtAvt4jsWrvEc2XTxY9NPTzLlBtArLsO3ys7jxtzxvKTlnfH7bvToU8GahoLf8MkvqmRnjARrq7zBu/ThZkSAf3VuVl5mz64TxAu1eVHhuMwMV9WV96myqlofaoai79B4N/AnhqOWm1NBzAGXqND1MBN1Ea7BHQrnH2if86aPkuyJGpruvVzBPR0m5HMsUcdoHdZnZexwFcBfFZjOZXMISGlljIzRN2o/PDCRVPaw7pMSZWAXr268DhI0QjGSbp8yVQcBod2hA8b8/icxwe17FPqBPSiEhakSrvoK3eUqZMfn91vAVSl+1JifCYk0VpFajYVXwvwH23ZR6uzJKbv1FdEL9Am/VQ1eKpJRCPm9dc+Z3SB+FJfWVviQXBJexg7gCOg93DlCOixr5lUtbRDQCfg3SzK+Ux/EbNVqz2zyaKHAXayqqiJJ1ru87bOS9UaGGjca24vcO04mUpNaVzPiOo2KquyU6pqIOI0/pyZNuS6XOfeNWPtVo2wfUIdHIEeKDxLslgNID+xwR0BPTHePtnLiUCPnUUG7qkvC34h9h7xt0yFgK7q3xqy80EQZsVv8XDvIVeHzOyaB2esfdcOJlIhoCs/apo8/8WAShHtfCnHOrEqpRnzfTlG7jfv9q76MNZuibSrXFkwyjBc7dojYBiv7pww5nOOgJ7IrBzcp5IrDSOwWUVZa0wHxepyw+d1Hl6kQkBXKdyP4M5FBFylh+2MR+mwQuJM/zktKjpH6zO/yTNPAA84UVMx0ipx33tGzlW6L0HZIqCrkg5MR2daStQYZ+KjZo6AHi9jTvtPMKAEkMcLj4XbKO+pJehk3BrMFcJAhCRqfBXBv+ka10YB/RWLRZm/vOXtvmyN7r9NYyVYY01dXaQMMg4DT4zKjxTrvBgRiwupEtCrVhefIixu5YTPLmPxboi1YbwlGFeFPwiu0ZmifLBZitbSdvNqgE+3aexn8vMjJYumJFdTvPrxyUfDcK0F0WfssJOBx4/OFhf1F9Fqx5jJYDoCeg97joCezCoanL6pENAvX1FwVCTLDIKjqcGH90NQMWR/qfe2pn0GVrUPNU1RwkxXqtcbcs7rB3XtEvj7kW0Tf+2f79damurTThwkwtQESiYxy1UAjkjMY0dAT4y3T/ZyBPTYWWTGr+vLg/8Ze4/4WyoBPSRI1U3+bPy9++1xyBroc1+dmzVq0446EF+sccyhDqVS7D5rWOLy+2e1PGuXs3YI6AC24BA10JUvVY1FXyeiWwEnRUyMcxtmxv2+stariUhr+uW+xo9eeuHOVgCnxWhfbM2YX7dE7pl+76o9sXVwWh2KgYWB0uxO2bmMCF6NTHXKCM1aMrt1vS5MJaCHzA83EdEYXZgD1UBX41QHChcSi78wILSNm4lAjNC+iKZf+MqDttQpVwd/wpT1JKLr0MhEigbJZgbhNQE5f7F3w4ClleK1yS4BPV9YRyzyDm4N2nh9T7a9I6Any6DTfz8DlUtgGOOKz41ebJM4AwL/5lwuGpT18agrPKrqXk3RynYK6HCbXt/Z69/pc/+9pNIwx2/6IzOuHRTW0nQQ7ik185N6b7AWqpTZID6pEtArVxZMMITZBkr07HIQSUqDoZj5ZTLwZV9p29o0MCdpE6oaiyqJSEV2q6g/3U+EWf7HKWs33J5MpsvqQNEXwfRn3cbtx5NhXrhkdts9g/07n6g/FwVKD8vKsBroVU3FlxB4ic73NUdAT3QFDV6/VAjo0bOYRs8vQfjWsD/zZYSI6Zq6ita/Dt6sJzkSgy5r9JxiRTNssYdAJzJwWJKoTveBGXja2tt1rv/8p/u8aDtw99haHByB3jh1okVWgBB9cU3gcQT0BEg7qIsjoMfOIoG+X1fW+ovYe8TfsnLZ5PEi291GwPHx9+6nB/OT+aOss/u71arqaohxb/2VQJdpG3OoAzGvhhALfaWtb9n5EmGPgE7vWExF/UVXRDdTDSVfhJC3AXAP9anU4F+YCX80TNfNi6ev26EBb0CI8zcW5Obudq0i5sIBG8fRgIE3R1mRsxbNat8ZRzenaT8MzF3myR+VxU9qjgTYwWBvfVnb07qIv+KxM0aEjexXIegoXZixCOgXPDQtL3dk5E4mfH7Y3p5l7oTA7/L3WDcvOj+5yJNDzd3FDUVj3UQ3CsICBg7XNs8HAKkow/1/JZBFxAcdqqtElSCSnx6fCUL0cYOaJQsGid5cKER2XABQt86ZdwoSARGhH98/q+U5O2p0OgJ64qvOEdAT587p2TcDlc+f6jbfzRttkTxZkDEHkKWQOBGEcSAa3pe6bFg06jPWEFyq63JSqgR0Rc3nmwpPc7HxKIgn2kBVhkDSJqbIufXejer7clCfVAnoNYGCcSyNVhCdMKgOZ95gDOZWjshrTmnZ+EIygnA6ub5g7dmjrXDkEQJPtcMuBjZLFiWHOp851LjR87yxmwJEmG6HfSD6Z7clPX+vaNtuC74NoNGMIcJYBiJ9c8bYZRLPuE/je/iBrlc1eS4E8Ded5WYcAd2GxaUZMlUC+uVrCo4Kh83/BXAJ0fA992Xw4+6wecW9c5rf1zy1tsOprI5Hm5E8GQmdwIwZBJoD4BQAR9pSysN2j9J+AHXZ4mt15a22XVZTDBwkoF/x2NTDw6bVBEo0gs4R0HUsreEmoBPh7wnX+RC40lcavFcH7/1hRAX0HHcbsUYBHXhi797I9KX9HM7X1kK8MKP4TgG2NT29nbwNHjZ1M3Eziawv+Gau2Wz3uDYJ6G/DbRT1F12hfKpuKPoiBDkC+sATvJ1BP9tC2b/Tne73UEOryOZudC5j1hrZrIZ8myQKhno64IGnVU+LmgbPEUzYBJ0vJIx/CQtli2cHX9VjJaAE9JCR8woJHK0LMxYBXY11WasnP9yBGwThIjBGg+EG9ewZmcFEHBVbCaSkV/WHuCKd+hLmFewglqdgQlRJVn5EAFIp+DsB7GLiNd2g211bj9tsdxooxWEtQ7zeVjQ6vNc4XbKcDPC43g16hIi7LYu6hEAniEKCqJuZuwwYXSErEoGbOw0ppCTZTWRGGNwlrYh0sburq6tn1bhHhj8Sxq2IeZBIrtoYluBQdtdBc2iE3X0KVla3JPcI+dE7RGiPK9rOnSuJEM4WlilCAjlCGIIQySYLLiLDFWF2G8RuSZRNkNmwKBsmu0lSNjNnMSgLYAuEnQx63m1Gnux+7zPv2zkPjoCe+KeLI6Anzp3TMzYG1AHUhNyOUaFdxgQS4ZlMRjEAJZDm9P6nLpSqmrIqi4e6zPPR5xKDP/H5xaxXgKfo997BF5I+9oxUPLDo68tRGcnEgtRFpB77lR8pyUTC4Pvltolf0PE5m0oBXfFeFZhyOtj4FTE+B2AEiM3eDYr6wlKXyVRqR/WfVN//6lJZ7xz2N01CrSnuWVdCLTBVPD46x+raWe9q+9Ra27+/6MHs2R8xJJgputFRpaWYei6t9X4n91xii64LVus2iqyukRkgUuvCANgAon82e9eK+rPCVjXs9zDjqTCMrz5Y3vxWbL9delulSkBX+9XIXrQQ4VS9Hg0pNFUje3moO+trD55jT3m7FLJFVU0eVQLkD7Zc+lWl4CC+WV/e8ocEfKTqBs/nIaDOKe2IkO+QAuctKQ0GErAtZV0uW+bJt7KwDBStF6znyUABHcAOGOI838wWleXUedKQATsEdIDeyc8PHz9QmZXKJae6aeyIYhLiK2CcJICRHP0cYTdAvXuTQTk7ie5perY9vbuf/f/vPf9htcPp2YTLQ5wI9exrCELtjXt3R0b074julZVPDEYYxB8y6DGZLX7on9ryQRoujbhNquVa8dSqVfk56D5Sgs8mydNA9FkG5wogh4GsfaVaXb1BCYrZA/eYH5+RqVO43ned6Ba093wuJoPUNboBGva82/TM4kf73UP0Sav3GeZ2cwSV3V8c3BUTHwk0OkhAj0Ye5UUaEq9DluECOoNqGj0VUuB6Aipi4LSp9wA2hqaxNyHwJICOPWQPxi4C7hICv7rfG/xX7Ojp1bKqoeg8EqTqgCYUVSvZmLmkvHmNnV6lQkBXdXpFwIlAH3heVa1R/LxThH71D+9Ttta43m9L6gR0z9cg8Jthn87nEIuCGS8S87XWUXuC/tNeUCmYB+1RG10xduTfiOh8rYMy3hcwihan6FBMqy9pADa/sXCGILFapynRdWeZFb7ZfacXTWSs6GHkTuN5ItKX+YR4G4Qx2zez5cmBbFJRE+aYTeOki8YgghwSUgiOKrrSNIQUkljmWFJ0E0vD6lOY7XeMLmFg5Cd/akZcFLGsQYkwNA1LRiSxgCsSASKGiHSTlJ2Rwz+zy3+af1A/NwaaB+fn9jLgCOiJ8+sI6Ilz5/RMkAEGfWP5XPf2vN25spNz4LLcQrCLpTAihhRCfYf0vk0KyxAyEj1cjD4RS2r/flHfhf164gIiXX2PaQolpQqDCC5hiCxLIl8AZ0rGhUSYPIiXydSNuB1u0zjr3unrNyU4Kx91S7WArgyJlr8xPjyGhBgt2cpS9xMEOGJwJBwRFJImhRGxLBkxZFaOjP6/L78tIcklmETEFDIsKWxaIkup1UbPnEbXV1bv+totjP3rLrq/MIlNy5ARDrNq1PN/wWrfYRqGlGZEGlKwFQlLwy0YXYBlmlLdCgmZkR78iEsYZkRY3WQK0xRh6nZJNrJMye6INNyGqUYhhmF2RmTog+xw6F/3znlmb7JzmGj/VAnolRsLRomdphLQVWSX8xzEAO1gxvXmCF5i56FyKom/ZMX0o1zuULPWTJGfdGh9/t7I7HgzUqnPQ8EjNxLoZJv4WecKd56Tyt/7RPxyBPSPWPsAoHm+stZgIjw6fexnwB4BHe9M8s49rpZqYzs7YdAF66eNzJddI7vZyIYl3TANQxhWdI9rkkkH7nV1s7J/TxPFDQHSsqRwUbTQnezdA+/fR5mG2p0cfG/UCkmSwk2u3r2UMEwB1SlMpgEy2aWcUEnvAMuKdBhZ2J4/Qr430CUD3b4OKh6DrmkvMN/fbY0Y2W3kSFeWu9uy3G6XUNtDUu8z+/eYMuKK/l3ZJ4k/mm8zyySyumN8t3EBwrDCIXXn8tDP/nebWN6bTGGSyZYhTTIjFnIMU4yEJU8H41wQeQb5cnAYFl/sm9X26EA+JvrzgwR0ddP7KHQ+nkQEXauvLFiSqEGp6nfNxgLXrt2uS8C4G2A7bgja6Zp6C18rDXFd/czWl+xIcWmn8ckK6C5XZOK909uTfuk/lI+pENDnLpubNSr7g8UqdYud/Gc0NvPz+zSQm3nb8Q/oiJyIlYtUCeg1DUXXM9HPe2/qxWrusGjHwB4CPbAvRqTW5219MxVOK8HRGLvp7n31ei/XOT6Dd0pJU/0VwRd04g5XrOqm4m8DrNJi6XyClhWZ49eYZr/nMNJ4hqAxLWUcArpOchwsh4F0ZcAuAX2M2DPqNu8Le9LVbx12VQU8xcRQF1hVBK2uRwqSBbpSSusyysFxGBgEBqi2FvTK9MnHWSp9PYvzQJhOINtrJ5Lk6+oq2hYle4Zgl4DOwMsUMct0XlIchPkcVkOkSkC/ZEXBUS630UqgCcOK8IGdjTBjrSB508neDWtrVWTgEH6qmkquBFuLiFQmI+2PxeCr68vaVK31mJ9o7XOJRTaVH1GRhNfVe4N3xJsFLGYHbGroCOi9xDI+ADkCuk3LTAts1eOe2WTiIc0ZJP41yTt3YswCuhZPHBCHgUFlgGoZ9NragiMiYbMChHMBlKKnXOFBGrRey/jO/HzrOrsuX/RpfE3AU8eM6gQdyTgBXV0aOFJ2qgjoc7SmdE2QwCS6vQdY1/rKNj6cBMagd01SQA9b3gk5fvKrdGy2PakQ0KPpe105DxCg6mU4zycY4L0A3S0i+K3OlMmxkpwSAZ1B1U2em0G4MVY7h0W7nvTRTzDollH54aV2fVnGwqUqu/DijKJfEug7sbSPo02ICTPrvcHWOPo4TftgoHIJDGOs588gLNRJkGSs5O27z/PP15f14JqlBbm7cs2nQDhJm62OgK6NSgdoaDCgSm90cac6HJmtzSNmOb57bO7v5y1XpQGG7FO1qrCQpFjvCOhDdoodx1LIQPR8gjpKWNLXiKAu+eu8qPLJtyrgoV1d26uWz3stqc8s2wR0wovh7kj5g+e0v5vCKXGGPgQDqRLQFzQUfsYisYEIY5wJ+oiBt4lwayRL3DVU0t8ONLcXrJuWlxtKJovqoUdg5lfd2ebZ906LsRZvz7nNYyDMGsj2RH7OwAtZI3jGPZ7MqX2+38+ogJ4NFSF4diK+99mHsYdBM+vLW5/QhnkAkC010BkfSIi5/vKWNjtsdjCTZ6CmcWoZk/VIb8mg5AF7EF6ftGbuSbW1MUag6xrVwXEYSBED0SCzI/51Biz5RTCuAiHXRlOesTrlHP+5G96zY4w+BfSqVcX/Q5KvT3DAjBLQoylKx731C9YveCRIX3LdVDE2IXhaXWlbS3JIg9c7SQH9LV9ZUF96237cToWArmp6WR3RG2/qto7zKAYYHSzoeWL81OdtXZqqG7epENB7opvf+g2Ivu4shigDqm7hm0zwySzjFv/UFlW/OLUPQ9Vh+6Y6tNBsiEUkzqvztqzQjDvs4NRLeziLHxZEM3U6T4y6yPYJV+jMhPHt5pKcd7u4DeDTtdlKvE2AZznRndoYdYAynIHK5pIcs1s+zBxT2abYvHUE9Nh46ruVE4GeDHtO3yHHgKqOXdNY+A0I8cN9dX7H2eIg4xVizKirCG5JBt82AR14QZIs93vtORBLxmenbw8DqRLQa5oKTwOJFmbkDeu5UHVLJbazwGq34O/dO7PtjeHEh7rE/tKMohsI9BNVS1e778wSRF/2lQXvjAGbqgIez77P1CbNwlvP0Cp5MomrfGUtKlNlxj2VgdKRQnY8SkQzdBnPjL0RwaUPeNs26sI8EKe6qfgCBqvAJlMj/jaTac59Non+Gu0ctlA1jUVlTKRXQGe8Mmnt3FMcAX3YLqvh67h6n1lVdKFkupUIE1WddxvI2GFZuMA/K7jOBuy+Da5p8nyVgT8mOGBmCeiPFf2bYZKK8MpP0N+068bAslGvRi5adG37wAUO0sD65AR0bvaVtU2z241UCOgLA6WHdXGn+sK23T+7+dOAr1JnvWkQ3RjuFo/757TsSJV4rny5et20vI7uSBBa663R23AbRb6z+66hXMmVhtH01h9AdJ0GPjMYghlMWwH6vWXK+7fK3M2rvKsiaeKQEtAvIOAfmu2RUvLVSyra/qoZd9jBVT9WeBxMWgWiz+h1nn43ydv6bZ0pEnsEdKsNIG0COhNtNSNUfv+slmf1+u+gOQxkJgOOgJ74vNkSgc5QtYHPcj6jEp8Xp+fQY+Ca2wtcu08wS1mwH6BR2j0k7GB2eevL1j2dDLZdAjognycpypMV+JPxzel7aAZSJaBXNRb8O5GxBqARw3eOqBskV8IUP937YfjZpee3dwxHLipXlIwx3FIJqP9mh/+qZM27Iqd8oHOHKxuKxoaI1uo9J/rYI5a8xgzR+ffPC+6yw0+7MTNRQJ/f5JkngL8DcGvkZxssMds3q+VJjZgOlEYG7BDQGfzcKWvmnekI6BonyoHKGAZUUKAY//YpJCNLQHSKDYZbzPSN+vLWP9mA3beAXhUoOY9YLk1wwIwS0KuaCm8niGsS9DVdu3XBbZ7QnxCXbkbPbyw+XxCrFPpxp6Yjgq/OG6yx26dUCOiVKwtGCWE8rPN2pt086cZnRocQ+Ke05P/tCon7lqfJi0LlxoJRxi5TpdOepM/nQwvo0QiUpqKfg+j7+sbMJCTuBtMmEHwhNv7yYFmz+rNK4J5WT/XKKZNhmBsANjQaxgS6oa6s9RcaMYclVPXK4lIYrC446D2AZvqhr6z1Fp1rMppaWnauB+HfdU0Wg7aaliOg6+LTwcl8BmwR0IHQpCMm5NWe5g9lPkP9e2CXgM4R68z6ORufG8rcOb45DCTCQHWj5woQ3wHorfPLQIQFXbKktDXR85+oO3YJ6Mx41p1tVMScPjkRcp0+STGQMgE9MPV0Aat5GEagq3fgXSzRKoBf5x0WWZXKMmZJLR6NnasDxdeB+XeJnCsOaAahU4Zx6ZJZweWHet+b3+T5PIH9BLIjuk6Vrvu6rzR4m853zgF919ggEwX0qsbiuQT5DxDpFNDfN4xIxf0z251L7RrXl04oewR0tJ+yZm6RI6DrnCkHK9MYmN9UNEeA7rMjsxYzL64vb7vMDk76TuEemHI6sZHoB3nGCOiVS051G+NGfjAUb6xKSd9fUpEZYouqKUPA35BIShymm3zlrbV2/HIciJkSAX1JSY4xTvoAXGC3f2mHTwgD9Mi+1B53gcMtdaXt29PpJSEVArqao97sIL9N6Hcl7SY5RoOYJQlaDcl3RKS1Hjs+87bONNkxWhFzs8q1BROMsLERoPExd4qt4R2+suBQu+wVm+caW1U3FP8HBKsU+1rT+zHxdfXetts1more2sxrAUzRhesI6LqYdHCGCgO2COiM0KQjHQE9oTXCkI6AnhBzTqdhwEBUoMZIP5jO0e4u8//41rb9ALWQiWLbKKA/444Ys+6dE2P94UQdcPolzIBtcy/5/PqKNpWRr8+nenXhcZAUBNNRCRufYR2ZuYOAO8FYYhl7nvR7X9iTYS7YZm5NoGAcS+NxEE22ZRDJr1ru8DT/jCe39oXfU3JvkyozONeW8ZlfN13W2ffNaH/XFvxBAL3i6TNGhLdnPwroK6dmdwp3mwT0LQRZXle24flBoN0ZIgEG7BHQufWUNfOmOQJ6AhPidBkyDFyzscC1c5d5IwE/0u4U8z8nidxJtTZkqe1TQL/goWl5OXmRnaTut8X5MNBeXxbUdtgb5/BxNb8s4DnWYmyOq1OGNGaGr77c/shsHXQkI6AL5vmLy9v8Ouw4FEYqBHRVE6I6UPRfAN1it39pgq/ScL8MULNFfM9pq+c2p+vGIlUCelXAU0yMZQBGp8mc2WVGiBmvEVELLNxn7ThubTqL5geSoGpsW9loBnCaTnKY0FrvDZboxBx2WOoztcmzBIRLNftuMWh+fVnrgzpxowI6OgNgFOvCdQR0XUw6OEOFgfOXFuSOGGGqqMsybT45AnriVDoCeuLcOT2HBQO95xdPARir1WHmFmv7xOnJ7LdtE1EZT8tu9yz/vLV9ilZaeXDAEmJAzb3g/BYCays7pAzhAQT0uctOzBqVPVbVgr4YCZxdJuRsKjoxh0D0IjMahBB/Pnn1nFfS9ZwkFfR8NGbPu953QVBZ2+I+y47BdkuYuHzxjKAKcjnoWdDg8UqBhwGMjAErviZqDUB+yVe28b50CiyJzwnAln030CFYeBeXt7TFa08s7W0S0N8jinjrvO0vxWKD02bwGbBDQAfz2klr20prk7isOPhMOCM6DOhneBgSZgAAIABJREFUoDJQeKQhxWoQPqsTncAfdrvcBQ9OX/e6TlyF1e+moqbJs4WBw+MdMJME9KrGqScQWa/F62MmtGfGP+rLg+pFIu2fxAV0siywx18WbLfbyZQI6ADUrWq2xFICzrTbxxThhxn8BklaB0EPWUztW0XWloFqS6XI1o+GTZWArgS1DnR8nyRdT4TcVPOgc3wGmIC3wQiwxAOuLHdb7osd2xZd2x7WOY7dWCrVfnWg+BECz9M81jaSe0+vq3hui2bcYQNXs7R0HI/sbANrr43XBcmzfRVtKlpc27MwcHx2lzzicRCm6wJ1BHRdTDo4Q4WBKx47Y0TYnfMIGKXafGLunnTkxPyhnsK9evWUybCMoNZUqY6Arm0ZOkBDlIGoQFT8AIg1v+dz586usaOXz1venShztgnoEk8JIzKrztu+LVHbnH72MpAqAV15FT2EhfEwmAvt9TIF6IwPQNRAUtYB1BL5YMK2ZC65pMCDQR9yYaD4+C4pW0B0pC2DEz3yal74kvYpnzyjUMJwTq7ZIAg2XXinNouyy/3eVRmdcSAqoOeaKkpf38VVQqeLyXtvWavak2p/KhtKzjHIekhzCvd3LRIz/N6WIalHaJ+EFADaIaAzuNHnbZtFaViKMgUUO0MObwbU+8xPwXwDSF92UAa6wLiwvjz4uG56+xXQqwOeZnD8X/6OgK57ihLDGw4COgO7YZGnflbri4mxFHuvVAnoysLLm6acHGHj9yA6DczZB1x7USn2rI+9YAGQzrrLsRN0qJasYumhosvDIHQSsJcltoJ4FYEfytsr31h0XntnJt2kTZWArmiura0VL898dDZDfAeMI8BwQaW8Z+oGuEv9XpCIspkH4hFgUrWeVZRKvk03sRNdJ8zAHmJsZcLrgvlO0U2P3j83uDuT1kJfzlc3Ft8I4p8kSkyf/RghJllTX7ZBa5SzVhvTG4wWNJXMlmwtA5HW9O1g7BImpiyeGXxVJwUL7zo+u2vi4Su0prhzaqDrnCIHawgwYIeAzpI7Tjlq4uihLqBXBgrPMlioaB+XtqXgCOjaqHSAhi4D1U3F/wHoL0djkTzK793wXqLM2SWgg+UTbqbZ91S0bU/UNqefvQykUkBXntU0eI6QhryBWJSA2A2ICKR6L+bNgrCdCS7JOM6AGM/gw3rrbqr/630nSJZmjp7tbAf4bYAfdGW7Fp2wsnmbE60YH7HVTYX/AYhfad2f7DeBWTLMufXlzStVkoT9/1zd4LkUAnU2ldpjZnynviz420w/J8lEAf2yNYXlMiIeYSA7vpV4yNZvCw5NW1z+5FsaMR0ojQxUB4pL913MehTQGLhEtKKutHWeI6BrnCgHKmMZWBAorJAsHtL6O6b2UUJ+yefdcLduYvoX0Js89wC4It4BHQE9XsbsaT8cBHQA71oGT/PPbHvDHhY/Rk2lgK6suHrdtLzdoe6jBfOI/VYJNiMQpgWEov8UksIwKWLazUW8+IYQkhnhCJndkOgcITr3uvOMvYumtCtR/aOXjnhxU9neJgH9HYupyF/e8nYsvlU+f6obO0eNzGYyu3ZzJJv2dnfNPrnLD3+0duE17QXmjh1qs5c9yjCsoyB4NiyolGZKUE/pw0ybhODfSWC9jJjvHBnO3/L7JCJeUupMH4PXNJXMZMhVuu0i8D11a9quSqY+pW6bMgUvmuIxa4wSz/Xddt/vPNFWC7s+o7sGYY/NY9UN/Vm6eHYi0HUx6eAMFQYcAT3xmbRFQAcsNuhz9TPtvxybuOdOT4eB1DJgy6GuUpoMOvXeJH73ogK6zFsPwhk6GZLM7ZxjzPZPbflAJ66DpY+BVAvoUU8YVNlSko29IXe2DEc2u8d0rypddUCwQfT9OGdXV/Zh3G0dKQR7WUU+gcbpYyIxpGgmNsYGAv8mIq3nRph5m49fvWqXI5wnxueVwaKx4Q4KMONziSEM2CtoUU7F/mhwlYGuxp4yYT1Lm3gruVxn+c5e/86AlqV5g8olJTnGOF4KcLk2U22OQL+sqbBcQruAvtliURLr2Z82rhygmBmY31g4gyCW68z8KYGH673BixwBPeZpcBoOYQaqGoo+KwStTST7+SFpIXzPVxr8te4LZ/0K6FVNnh/tq4F+U7xz5Qjo8TJmT/vhIaDTSxZZ3mRuysfK/sWPnXF4tiunnYFjY+0zYDviJ12hrun3znlm74BtnQZpxcBFgbMOy5LuViI6WZdhDGwhQxb6Zm7YrAvzQBz1Ynf5cs8xVg5/kZkWApi47zPejtpgsZj/JjN9tb6sdYXuL7VYBre7TfXys45HVlYrgCN0jsXMW4SwTndSWMbPamXTlJMNMlQ98aPi7z1gjzZfWdAzYKs4G6hLMuK9vKVEmB1n136bOwK6LiYdnKHCgCOgJz6TdgnoFBan181pcWpCJj41Ts8hzkBVY8EJglwbGDxap6uGlEX3V2zYkCimKjXVhY5mME1OFKOvfsy8wXC75yyevm6HTlwHSx8DF6yblpfTHQkS4RR9qAPXQE9qLAZd2VRydIgiV4PF1SBMTGG2tj0MvlluM/7gn9/SmZRfTmfUcq14oWnZDwVRrU10WMxcU1/e5lf41YFpUyAjDTYFKoR7z03+MhTOTb6x7MSsrdljVZ14be+3KsulnSnc7RDQVRnLcMia9uA57e/atEYd2CQZsENAB+FvdaXB+Y6AnuTkON2HBANXrJ96eLjbUmUwtdZBZ+BmuW3CzbpL3vQrnsxvLKoRRIvjnRVHQI+XMXvaZ5aAXngJQSwBEF/6cUaL0Y1z7p8X3GUPix+j9gjo2U8xSKf48nSu25x+59nrd9ttv4Ovl4HK5pIxRpfVApC2D3q7BfT9DNTWQrw4c+qp4Mj3iHG59nTWMVJNoHck+KZR+ZG7Fn2qhliMEGnb7LJlnnwrG48BKNZsZAQQX/CVtcT93azZjoyDq24qWQCWd4Hg1m08S9xWXxH8mm7cyiWnuo1xIx8E6Fxd2I6ArotJB2eoMFAZKB1pcuejDMzQ5ZMqpSKPmDDOf5q/J0XQEH0cAX2ITqzjVtozULmiZIzh5hcBPlynsUSYXucNrksU0y4BHYSg2Ouau/g8R0BPdG7s7qcE9OxQZIMAtF0u77GZLvSVtSqxzb6HQZ8PFJ7qYvGfoGgGzvjOpPRZ1gWBRUYHfjgY51v6zE5PpMrHiv7NcKEZsKcWumQ8zNsnXJI96uXskDt7KTGV2nEBgxntUkbK/bPad6Yn0/FZZYeAzqrUnUVlS2a3ro/Pmtha2yGgA/wKSZpRVxHcEpsVTqvBZsAOAZ0ZPl9ZcIEjoA/2bDrjpSMDC9Z+brQM5SwHkd5gJOb/eU/k3rjKu0plPdb29J/CvbFwKkisSWAD+YRvTbAwE1LMVjVOPYHIek0bm2kElFEC+urCS8hKQEAnesQqPe4iP/k/kZrLjmlwBHQ7WM1czCsbisaGRPSFLOME9P2sX7OxwLV7j+s6lvzfuiOlY51ZJuyG5B90jLLuXDqlvSPWfuneTnG7a6epbonHXQZlQN8Yd/vKg1cN2M5p8BED12wsyN21y1wKQH/6dsAiwsI6b/A+3ZT3COh56nLZhdqwCdsEjFmLvc1PacN0gBwGMpgBdeifG4osA3C2LjeYsVNun3C4f74joCfAqeVEoCfAmtNlWDFQubJglDDM5wk4Rqvjkmf4KtpUJEhCj10COgOtOdQ9927vUx8mZJjTyXYGegR0uUFA6hXQmS/xlbf93XYHAFQuqTSMsW99GUQ/AmzJWDWgGwxEAL6DIq6f+mZnfrruAR22swGDqlYV/4CYVRS6/jKHjF1w02zqptEspNpH2pLZj4lvrC9t+9lQiD5X022HgA4gbBHK/ElcADvUUqxpLCpjIlULW1sNdAZekKa71D9j7VY7fw0c7MQZsENAB9PddWWtVzsCeuLz4vQcOgxc1urJt/bywyCaqdMrZvx6i8i5YdAE9JoGzxkQWM3AYXE5wvzkpLJ5U2qpNlqHN52fQRTQVbqxvxPwFjNOYsKFBOTZyc2wENDBf/GVtX3JTh73YzsC+mCwnDljDAUBXbEdFdF3GtMl0e0EnJiSGWB07EuZd5eVH/lv/5ShcbNa1eCravJ8nwg/t4HTt41cnHp/sf2ZN2ywPSWQNSs9n2eBepAtESW7CPKCurINq3U7pwR0MTavnggXacMm3gZhzPbNbHlSG6YD5DCQwQw4Anrik1e1ouhMcpNK9+xKHOWgno6ArpFMB2poMrAwUHpYF3eqMgd6SwURSuq9QVWCKKGnt/RMCxH+PSGAfjpJQgtHInOHSgSmTm7SBWsoCOiKy9JAqXmMtXeyZdDtYDrLLlF0gHmTktHIJl/rn9n2RrrMcSbaUbls8ngz272KgVPtsJ/A7UziXTCfZwc+gM0Iy2m+OfaU+LPJ5kPCZqKAvqBx6gwJazkIubo4Y/Bzsitc5p/3pCOg6yJVM44dAjoz3eUra/2iI6BrniwHLiMZUBeCDcN4BCBtgQyKCJb8yy1G7g8GTUBXtbVImKpe6HFxzYQjoO+nixl4mmF9/f01I4Oraj9OHXDN7QWunSdTBUnjfwFMiovfGBsPCwGdqNbnbb0pRkqSamaXgG50YYaToiupqUlJZ1sEdMa2HEGFd3tb3xxspxY0eQok+C8AnZGig4IIAfeaYeOGe+c0q5cIHmwOdI9XvbK4FAY3aeeTISFwj7V197X++S8M6fTAOubkor+fdVj2qKwHbIo+B5g3MWNWfUXbKzrsPRCjYGOB67M7zXuYUK0N2xHQtVHpAA0NBqICejiyDOxEoMc7o5et8ZxqRaAu4+gsjeEI6PFOhNN+2DFwScBzbBbjKQbG6nRekDE5mQw10QjecZuUAD9Fp12OgK6TTXuwolFEHfwEQCdoHWEQI9APtLuqoeizZNCfITHVpgu4A9NE2EgWvnhy+dznMiE4aWCHBr+F+kwSYzffRMQ/0P5O3nNgIfdFuMOmkniSwd+r97bdOlSiz9UKsEtAh+TyZDKoHGp1zm8qniYgHwNohK5VLCU9axjhsjpv+zZdmA6OXgbsENAB+mudt/UqR0DXO1cOWmYyoHSVbkGNBJyp1QPCT62tE2oHrQZ6TYPnCBZoBHBaXI44AnoPXRJ3WqHQDYe6UVb9+LSj2Yj8UWt0We9kDQsBnekqX3nr3XGtzwQb2yGgM+HZHHTPcNLRJTgpKexmh4AOYJvLyCq6d+aawb9pzqCaVSUnM1t36L79Fcc0WcS8phPml/9R3vzPOPqlZdPK5pIxRqd8Yl90/UT9BnKHQVR+fxJRQvptSk/E6pVFC6AOwIAcmyxcl00559/tXaU9ragjoNs0Yw6sw8ABDEQP/TuxAowSXcQMlxTudgnowsApi2cGX9U1Hw6Ow8BQY6C6qVjVCmwAeKRO35iNE+uT3INXNRatJ6KpWu1yUrjrpNMWrB4BHao80L9pHSBFAroSWysDhUeYTP+PGVfYJJAOSBUzXhMkv2RHpqsBBx8iDS4PlJwYYdkGYHQmucTgp7IkKu6paNueSXYPZKsdAroqfUAWzfLNal010PiJ/LyqobAQQiiRR1sWWQZekySn+70b3kvEJqeP/QxUNxRNh6DlALRdnIDAw3Uzgxc5Arr98+eMkP4MVK8uPA6WaAZwrE5rCfhB3Zrg/+guLd5vnZYrHjtjRMSds5LjPVByBHRV/GZZnTd4Xiw3BXtuam9eA7DeF03GP+rLgxfrXIR2YVUlWgM9TMW+Oa1Bu+w6ELcyUHikwfQsQON0jafS9hgdnTMWn/esSvHvPBnEwMWPTT3cbUaaibTesk+dgN7L/YLVnpOsCN9ORN4UTQczcxsJfH3S6rYnamuR9qVA+uOpkisNsWrz/xDzd+y48Q7mxfmjrIWLprSHUzRXaT/sgrVnj5aR8KM6hbE+nP5jnTf4DTteghwBPe2XmGPgEGCgJ3WYuQJAsS53HAE9KSYtzjJOrp+W+RfpkmLB6ewwcAgGqgPFC8GsLgcaOomyQmKs/5yWD5LBrAp4lhFjbjIYn+7LLJ/IYpo91IQknRylGkt9lwphPENEE7TakjoBPepGTaBgnGTj/xHoSs3lSuKgibdKxjd5+54HnOxjcdB2QNPqpsIfAuLHuj8zE7Mmtl4Mvr7e2/arWM6UY0NMj1aqRJkxPv8hMJ+jyyJmWEYYsxafEwzowjwQpyow5XRIsZaI4itve2hj3hFSTl9cseF1O2x2MJNnoLqxcCpIPK5TQGfgKbltwhTdkbHJe+sgOAwMPgML1pQUyYhUv2OjNI7ObNHX6me1/kkjZhSqXwE9WsN1VfHDFG89l+EuoBPCiNDseG6/zW8oukgI+rvOyc2oCPTGokoi8gEQ8XDQsdvMf/jC9bvj6ZNoWyWgm0zPMUhbqjxHQE90NlLf7+L1Uw93dw09AV0xW/1Y4XFwiV8C+DwAc9DZJjAkvc7gr9aXB9WXacY+VSs9xSTQANJ4a/VjNjrB/B1fedvtQyHlve5JLg2UZh8hO35BRF+L97slZluYJQGz6srbVKp+7Y8joGun1AF0GDiIAUdAT3xR2BWB7gjoic+J03N4MFDV5LmTgKu0esu8LX+UdXSyFzOrGovuJ6IFem3DKxZEmb+85W2tuA6YNgYqNxaMop3G84LoGG2gCijFAroyYfZjZ4wYbeRcLwSuZyBbq3+xg33AhJtHvRK5bdG1zuXp2GnraXllY8kxISFVedKT4u2bivYssUtGRIH/nJbXUjG+nWNes7HAtWun+RBI40UrZimYKhZX2COgVweKjwfLDTqDqUDYIdk6f0nZxvV28u1gJ85AZWNJkUFSZWXWlu2HmbcKYZ3qpO5PfF6cnkOHgZqGomuloD+QRt2BGSFIzK+fFXxIN1P9C+gAqho9txLhW3ENSvSktfW4wky4UVPVOPUEIkvrpkSlYjllTfDkeCInK5tLcswuuYV1poTJoAj0mqbC+QyhBPRDrsdPrEPCuz5v8Oi41mYSjaMCOug5Zs0Cuss9Y/H0dU4EehJzk4qu0RIXxOuhNwJ9u5CuosUV61J+C3XB2s+NtkI5fyAilcXCrtTXh546gQ+kxDW7u8Y8snze8u5UzHOyY6pMLiFXzmoCCpLF6rM/Yy8Dl2T6RQM7uKluLLqYie4ivbcZP2Eqg1+W2cZU/9TkorX6898R0O1YGQ6mw8AnGVjwyNmjZW5YXdbSVrPXiUBPapU5EehJ0ed0HuoMfHFFyZg9ptxIQm+qbIZskNuOPyfZM5yqpqJfE0hlX9L5bGEyKuq9zc/pBHWw9DGwMFB6WAfvfUFAHKUPNT0EdOVP5fOnuo13824kga8yoC2gIk6uLMn8K5bWz/2z2nfG2XdYN2cGVQcKawniRxlARASEb00qDf6pljI3G19/PNsioKsKqkSzlnhbG+yY32hWu3BYff/oPH+OALjWVxa80w6bHczkGahp8JzBhLUg5CeP9hFCl0k09z6vPeUGNNrpQDkM2MqA0kGNbrkMjFKdAzGwG+Dp9WVtT+vEVViHFtADRdcS0//FM+i+et5P5eVFipK9vRzPmIm2tUVAl/LX9RUb/jNem6qbPPcAuCLefv21z6QI9IQEdMZaX3lwhi6+BsJR9ephRl4ENH55Ej/vzsXMezxDq67RQFwOhZ9fsqLgKJfbWE8gbXXeGPyhEaGixbPTo+5nZaB0pCE7rwO4FkT66v7EtwB2SMItp5bOvbWWajMunXst14oXVy2/hRg3xOd27K2Z8UKY5fkPOum/9pNGVY3Fk0H8N9Jdh/HAaWFIJv6Nz9v2n3akb1dD2SKgA9tNk2bfN6P1idhXmdPSYWDoMuAI6InPrROBnjh3Tk+HgUQYKA2UmkfJzkUM/oINNZl/VOcN/jTZPU11U9G3AfrfRPw7RJ+ItFC9ZFbwAc24DpwmBnq+S0MvAzReE2QPTBpEoO/3R5VepHGbLhLAbQAO1+pn7GBdYPwtN8v86p1nD04mxthNS++WNU2Fp0kWzaRXDNPutHq3zxHd0+72PvWhdvA0AMxEAV397otxm14i4EStFDLd7StrvXqopenXylEKwRY87jlJmlAlY0drNYO51to+8afJXljUapMD5jAwuAzQ/IaiK4UQtwOcpXno9/bujZyw9Pz2Ds24hxbQ5y6bm3VYzvt58QxKe3OsxedlRkStHQI6mL/iK2+L69KB4nd+Y/GNgvgn8XB9qLaZJKAnss5Epxm6f15wly6+BsJRAjqbkZdJY/oWAl4ARWY66VsGYj/9fn75moKjwhFzvVaBjrGT3VxUP73tlXTxWNWoEuPyFhJwc+9BQexZIvQ50cWSf29kdf588fRnMy5bw/zGwhkCtBJEbn2UfBqJXwfEAl9Zq9rgD9+HQQsCnn+XTPeBeJKtRDDvBFlzfGXttnFuk4C+g4WcU1+6YYOt/DjgDgMZwoCKKrFC4ZVE+jKFDJcI9KqGos8S0bMg6Px+cyLQM+R3xzFz0Bmgqoaic0nAB+i92MqMCJN14ZKyjcuS9UplAAKRErq1vjMwqGFX17bzls97LSOzUiXLa7r3r1xRMsZwWa+A9GXri/qcRgJ6jzmgmtVFZ7PEbQJ0KsdZglDHPPK+2+1grCBBX/V5W9/UgTkcMGoZ4qWm4p+B+LspKVMXK8nEtb7StpuHqqg6d9mJWaOyxz6sqiPESkks7Zgxx86sfNWNRY0gKovFljjabAbRDOf3OA7GBrHpZQHPsZLRwsCxmodttULiXP859mQx1GyrA+cwoJ2B6pVTJsMQfoBO0A4OPO7zBs+x4ztU64uNDY7bCmmHgE5EN9Z5W2+J1/CqRs9viPDNePv11z6TBHRdPtuJY4eAvq/c9EskUVpXEdxip+0Otn4G7BDQVaqRSDhS9MCc9pf0W5w4orpxa459a6Yk3EagkxNHSqYndwO0RHS4vpkpF7T2e3v+0oLcESOMZQDNTIaBAfsyv8JE15+yJrg0nhIiA+JmSIPaWogXpxfPAfGvCDhF98HtwTRws3C5z7OzBIcS0E/aZd6rKuponIYdFlvn+Ms3tmnEdKAcBjKWgcrmkjFGNzeAebIuJ4aLgL5gteckGcFzWgV0hmVF+CT/nLY3dM2Hg+MwMBQYqGwqmSkgVWkabdmvDuBlB4O9OtIdLlhTUmSF5VrSe7EGzNRtEFct9gYftuNQbCiskVT6oL5Lqct6XYBGabVDotJXEfybVszkwahqdfEkisg/gWx+v+vfVgsS7WGXuO6BmS1PKW0/ebeGPoJap6JLNhOQojONATnewmxMqy9v/ueALTO0wTeWnZi11QYB3ZB8/v0VbY/YRUt1Y5H6fb9OMz4zo94d6fzSvXOe2asZ24FLkoErG4rGhgSpGuhnJgn16e6dYPqOb23rItQOvTINmrly4IYYA1WNBScQG3eToKl2XEKURD9c4m39qR20OQK65hroAK32eVu98bzYqXRsR8rO50H4rK5JdgR0XUz24FQ2lhxjCPkqWF89aGJ+OSK41O/d8J5eax00uxmIrgfI9SBM1DjWHjaoqH5mqyoVkF4Pg6pWTTmNWPwBoGmpuLXN6s4/8LiLjK/f5215Lb0IOrQ1lzUVT4uAV+jMYNHPiF0AfkYSi4bTxRwV9SJc8koi/hFAelNs9Uk0WWD535PK5v3SztICKgOEOT6vnhkX6Vrv0VIRpjFn8YwWR0DXRaqDk9EMqMORsKAGBs7S5YgjoCfBJMMSME5YXN78VhIoTleHgSHDwNXrpuV1hEOfB9OvAM3RvftZYrzawZj+sIZL3dXrJh+NkOsF6BZSozcj+QVpiUvrZ6Xhu9KQWXGJOXJxQ9FYN+F1ItJZKxYEqqora12SmFX29lJ13zu547dgUUWkPQVpbMYTXpTCuK5+RrO6tOKI6AOwpgIDjHGbfwnwt2MjeBBbMaQE/XhJeTQYa8jOZaYK6DVNnq8y8AcbLul3gHC9tXX3Hf75L4QGccU5Qw3AwMI3SrO73uh8SHe2BDWselck4susbRNXOKncnaU4HBhYGCjN7rQ6Kojo1zq1z09yR3skuGpJWTDpjFp9zYkjoGsX0NHFBv17PCJY9eNFU2CS1nSqjoCu9yPoyuaSY0Kd1uta0zAzXkWWWeo7e/07eq110OxmoPqxwuPYpHVENEHbWMwdRFxUV7bheW2YmoEuCXiOdUn8jAg1qRDRwSwB2mgJea3fu+HpTHm5PH9jQe7I3eYjzPBqnpK+4EJgPMcCvxyVF3lg0ZT28CCMmZIh1CGI6/BNM6TEj5i5hIh018/p2y/Gq1aOKPZPtTftlhLQjXF56tDwQo0E7yTBc+tK21o0YjpQDgMZy0A0uoCoEaQxuoB5l7V94nj/fP+QPgizKwLdEdAz9tfJMVwjA1c8dsaIbjOrzCDxHwyUABihEf4TUAwsy6Gcz9/tXaUuYib19GSu2vQ8kw0RngxJzM9YxN/k7cevdw6dk5oqrZ1rAgXjWJqbQPqCDZSB6SygK/sqVxaMMkzXN5j5egLiKn2pZQIIKoL1HcH8vXdFrn+Vd1VEC+4QBqkJlExils3a6xonzRk9Z3W5yvzz1m5NGiqNAb6xbG7W1qwPloIwS6eZArhocVlQpYa35fLB/CbPPAGobBg5Ou3uxdpJ4EVdWeav/j6t+X0b8B3IRBhQQUSBot8S6Os2XJxQFv2LgZ905EfuWzpFf73mRFx2+jgM6Gagsrkkx+y0SiTRVwlQZTBsC3hi4I2wlBUPVmx4XbcfPXvSYfzYkcI9SifTrb7y1u/ESC1VN3ruBGFhjO1jauYI6DHRFHMjVf/EkvxPrQI64VVLCq+/vOXtmA1xGqYFA1EB3UXrCBoFdKDLsETR/bNank0LJ/sxQtWtys8e+wNCtOSE3jR9sTv+upT40qnrgqszIl252nw3FX2RiP4PgBG7m8m0VGnv0S5BtxgS7Xn/jHyw6NohIKYzqLKhIN9lmCdK8DcBcTHsuGloAAAgAElEQVTAI5NhKs6+YWb6bn156+/j7Bd3cyWgi/H5fyPm8+Pu3H+HnWAxz1feog6OnMdhYNgzoA79AVcTM39OGxnMeycdOXFM7WmOgB43p04E+keUKSHSPWHTiJM6czpqM0wUUdnV8vbsdo8fnW3s7uwS7rAZPdAO5eTI7NAH/GHIxWNH5kn1b3sPf1+O7szhV3bn8fit46PtTnvBzz/+MTijIioZ9OlT+5sAet5fedB5y9bxW+mzebtp8/s7hWmNMrKz4UJWrluEO/JhiDEkhardtwAMVRvQFffvUZwdWEb3NbfGk0HvUENUN3lUlN5XbTtrYuyCoHtY4q6wK/xuZEf2niOP6epaVNAeOaQPfczRofyITlx6RPWSysN1E2r3rafnaf/62ZHTSSPeP1zszeqm7N17RZd7DLk7O0XIFaGwaUXNl64RVnjXltDyea+pC122CEtqnOh3KZubGciOc/kdsnm6C+i9xlP1Ks/lkPg5gGN0+h8H1g5mfDdH5NTpuAgTx7iZ17QWonqG56dgfB8EkS4OSOablpS13ZQmnzm20aLEFKNTPgKKCin6HkKlrzT4gF38Va8smQxDLgdwhD6jP4G0LwEBvwjJvyUh17pyja073rc6szs/0x3rZTHmj/Wdm24CPX/qwfuPT9u+f7/16X+3+fuv5zvtABv7+l4b7Q7Rjt2dIs81mtRecv/3mguHRfZ05oSXz10esmu+9/NRFSg6D5Ie0F2WZj8+AxEwN4PxS7cLz1vC/aE7YnYev3pVaMBzzt49TV97TcWnHet0/z49Uey+7FL74R2vd0bt3TMyRCcC2J1zbPTvan/TtT1E2WPdvOfDrdLT9lp4QF4SNa6Pfuo8bnROjtnpDn/Ep3qHUfaM6M6K7qm2dP6LR+5x8+gdOfzKZ/Oi/3bQ+0z67Cc/6eUA++L+3mP2gxz4PnPc4aOMHTvgys7Nygp1IQ8cHgNCOSCqBeFk3fvDvqaZmZZ2dISrl55vz4UUW36pNK5XW6FsE9CBPT5vMD+WD/PqQNEXwaReMrW+bDgCut6lU7268DiKiDdZ6yab/2lZVpl/VvsmvdY6aHYzsKBx6kRJ1loAx+kai5lDgqmwriL4jC5Mu3BU+pUudJ4Hxl8AaE3VF6PNamPyNjPfJLdPvCvWl4oYsW1pFo1QMExVl+tsWwboD5R5L0O8IQRaZYT/Ul8RDMby3TSoNsY42GXLPPlWDq4hyecz0akAxgCDe/DBjGelNM/zz1pv++d25fOnusV7eUuJMDtGimJp5gjosbDktBk2DNQESscBXVoFdJbcccpRE0c7AnoCy8gR0JXERZVNUwpNMq5j5hMZeJPBf15SvmFNAowOaper1kwe3xnKqpEki4UQoyFlDhiG2ncQwBJkgcgC2CJChMBSSqi/SwZJwaxUP4ZhqH9jySzV3xnURSAJll3MH4uAJEhdFkwu4pJAbLG7z8xKQuTQAQfSikwmFoRPpmumaKAsHSTEsGSDWLn+8bPvzJjYIAGLBUAmBLKpJ7r8sN6052pvoyLcBkXYYWAPNJeQqmryXEiA307xn6NTgV0A3iHgPUnYQcBeBjqJKXo5QxIbAtG57X1IEMURQCIBqaLeRbSM1AGTSCGotXzgo1aRjF5e7fdhQliQ6tsTWk0SOftEs4PP4wzhJglTkmUIkBtMggRo/3qSRAaIBZgMgjABVpdzTQkYBljdVjH3X15QtePJwE5mPOEyI3fcN6P9XTs+FCqXTR5vZLnfBem9KJwhAjrUpaEjaO9kkuIudQfIDo4HwiRgN0j+Zc8e+d92HR4PZEOm/Lyy8cxjDJG1GkzqklLqH+adDLNgKNc+30/y+UsLckfkmioCPaME9MvXFBwViZgrAJxh64IhhMHYDuJ3ifE+gz4EsJMJHxAjrL47mDmLGabKvkdq7yE5m9Q59SfOqplgRb8bDvmwIEnq26iPZ/8e7NM/YiZJiO6/Yn/UjobhYildpB71naa+26QlGMoINoiYIMkkAwYxCfVdJiVMQWxE95KiZ1+kvtdA2MUSz3RbWXc8NGfN5tgNia/lBeum5eWGIqp85OHx9Yyjtbqox9G9wXtgqcq7qiwUuwGxk0l2qCrpRIKYOCvKi/o/WIDUHiC6g/7kXpMACTLUfjMOKwZsqvbjAmwlfBUvusEnIT+1v1XzzqzmliCUT8zEH+2pOepHdI0yQgzeSWS0WYg8YFcp3NpaiBemTikhgy4lEhPUHl0yqX2V2sGr7afVa4/cV0JSMqv3FVjEkEzRlwRJUmVOVYQZ0Tbqz9JiSeIg9iywiC1jHbPy/+N3HrUoVAbOT72nfDSRimlJ2VC/V/09vWuovx/39R5zYFsWaofMJkX3nSKHCbnEOAykAu14dLQEch/vRwMutkQbCLrGN7P1z3add2v9hUrUx1T1s0tAZ+C9+rLgUbH4VRPwfIsZt8bSNp42joAeD1sDt61cOW2CYUT01mNkfl3ALHPqPA7Mf7q1qA4UHw9mJaAfq882DgviosXeDU/pw7QPSZ1mLgiUzGDIP6buoIA/ZKIbd3Zu//Pyea/Ft4m3j5p+kSubSmYKyIcoNZH76vAvzIyXiWidOkgTgl8iS75DIbEttGfCXn+lv+flKblom4N7H2qn8alYmEp/pcD453PcyDssFJbjSNDxhiFOsywuEcRnA5SXwmgBC4Rv+rzB2+yM4tm/gFS2h1HZ4x4FuFzXcmVgNwk+11fapj6/nMdhYNgzULlm+ngjEgro/B7bl8q145Qjh76AXhkoOVGwfJZ0XgIexgJ69JAuHJlDkq9iUGlvKmQl9zEkwky0kQTutthaatehUaIfCFesLJhgmeY3LMblBIy3KdvOp1TM3pNBlcA4yYeJ+typ9LN9GWLnJ9xobdszT2ft1QWBwrOkFA0gjE1yahLp/un1YNd8HbTuBlqI0bPjA5cr0UFZC3q24R89Om2XIOxkC78/fOKYn/3+pOVa35uqH598NMysTb1ifiLz1ncfomqft7VeH6C9SOp70ZDRd+Ny3ZcJYrOcu5nFHWJU9k11U1Zti63P8GtVy7XipaZlPwPR99PAe0sy1fL2436eCUEByfIVLW23y1zKPal8tT1MqKkvDdYneY7R/znOkpIcMU7eR8Al2oyOD0hGvz96RMjeAHFbvititWqgr7y+cHR+pyl8yeC9gunObJf7lrtm6C9/UFtbK16csfwxAipiJUZnO6XW7sezaW+g09zBwlKXbHfse3f3C8m/WqwpXXflEhiucUUVEnQDgGIGsqIXZO1/Yv5dOrBhjIbF2Mx+J20fgWlTqEvMePDcZr263QGGDx8y+5gtuwR0EDb6vMHCWBbI/EBxhWBeGUvbeNo4Ano8bA3cdmGg+Pgu5jcGbhl7Cwa/IQ2U+2e2acWN3QKnZaIMLGgo/IxFYi0Rjk4U49P9WN1aM0Whb2bLk7ow7cZRt/NenjHtFKbIrWCVnmVwomU+5VcHS/xRcuQW/6z2nXb7nAz+NRsLXLt2Gb8F6CvJ4Gjqq24vqvroW8D0Pgt+F8zvEIv3WchtYOwixm42RBdJK0QHRHip28DkMl0yLLOE4FxmI5ctORJCuMGWmwRMyP/f3p2A11WVewP/v2ufczJ1oJRSQGhRwTIIAmmTljJkKKOAoqZNKSgXFUUEUXFARaM4oIh+iHoVFbgiTdI4V5naJulEc1KqqJdBLw4FZCoFOmU4Z+/1fl0nqQK2zUmyz5j/eZ778H1277XX+q2dc9be71rvMgPjC+PmLsPfbRpWdZNYTUyMlljIOLd3oAKTRTFJBPvqwExftyrLzfjMykqsvdi6qbGdvg3Oz9Z9dnHHoaV9dmo7JLX3aSgfF0BXtecUwkrGUBrMQigwhMBFa0/cP9kfrARwRGhYisQRB0wbX+wr0Aeeo/w/ARLefpAKt+Ty0Dtr40+G1h/5XpDb5mXFrJk7F5e4pOW1EJTvscpuZRLwh50rlL/aUht3e3Hm/HPB6mMmBcmKNoHW5sFvdc49CqwCbkX/W1rr4/eFWe+Fy6unqkFnqN+rYVZwLJelcClvby+Vsg+Fmeo7lZ0Nwd/CnuSqIhe21nbdWUhd1nDPnH29aOAyn14KwcsyIGSpFW4xnMhSG/hXMtPhns0XtFe9yUBWay72rn9ZtQT4M8Q/qbl2w5iY8NDQUTPO057fAHJqmH8RxrMXLj5l/eJMBdBdXRd2VH9UFV8Ps94sKxSBQFXu9mKRdy4+eY0LrIb6WbCi6os7V9xfE/bvW6iVHIuFubeMgg4TjTWE0e8Ll1efpgYui0yutmIZi70YVpvd3ILrj1gV/0wmU/zvNYDuBn+REuvSpBbkx7fm73vbXzpjAXTg5y118beng9bw21kHeGUm9FRa6QTQG1fOOV6sdcGJgvvYwPa3zlu/PlsVv+C+4w63kZK/hHy9fxhr68OaMRVy3VjcXgTc7HLRYJWBpJVpIh3MVCpCiVS11K59IJ3j8+mYxvvmHqRR/1uiOC+T6Rr32GaFr4JbyiOxpkzMPA3TumHlnGM83y6DZGz/rDCrG6TuS5cF6uVLZlyKJZfA1OXbcqnC9pQ2KMya5L6sZ8TijGxusZDaI67PrgFwQnjNl+0QnNtS2+VebPNDgTEv4AI91mClADPCw1D/iKnTKxhAH5GoH6g5dG/PbyMqNU9PcqvgHll5zxmw+gMZzgsbl8bPmhsqyryv3nrS2m25ap5brWH2q/oyIB/L0iqNXDW1KK8rinUSi745jBePLwdqUphHOqp+LJBFRQlX6I1yQXSY2pb6dfeH1ZTUezUEfwk7wGAtLloyL/6TsOqZrXIalswp8/az1+xMxXv54JZT2br0ruu4bAer1PqXHbn2d3/O5AvlbDcstOulJq9V3yCCD4d93w6njjuftT/XWhu/LpOB3+HUJ9PHXrJm7viehH9X6Nvaif2vlpr1/5NJR/cex/hBl4jseaJjpgFZ/m4FBt6lmve21Ky7Nex74IKV1WdbHz8dzAzFHsgvAZcf/dNH1ca/2iTuneXIPuffe+L+sajfIUhtE8lP4Qk84UVw5p2nxB/OZNX3GkBPfVEE+FUmK5DRso1+vKWme4/p0TMVQBfoN5rruj+aTtvcA+ajHdVullSo+winFUDvmP07qB6TTj3z7xjZ2FLXdVi26tWwvPooz+ChkK/3hPFRv/j0+P+FXC6Ly7DAguVVb4DIKgk5CBoYPbGtpntdhqufkeLPX141OSZyjQBX5GS2vVtNregMIvq+fM7qkPrOb5/zFhX7P27FdUY6g4WGLRCI6vX+5u1fCDPF6VCVTO0RVxHZEOYKroH9Qe1bWurWrxjq+vx3CowFgYaOWQd4MKugODy09qraUlNeEebqvtDqFmJBGVmBDoypAPqCjjnniAa3AbLfCLqmT4CbZ9Se9ckmaRrxS6MRXPdfpzSunHUIAuNWxE8aTTk8NzcCAvnEjFVdX89EcC21xYO1f5C9ZVTITbN5VTcx1uLKlnlxl2o8lM+CZbOPNEb/V1+xB+/oiy7UALprecNDR8W85yacr9beJCJTR68x7BJcvueHAmsvbZvX3ZWN7aeGXcMcn9B476xDNOp1CDQ3e6Gr7tCIP6v11N89kmOKrF2+YVnlRM+L/BbA3FAvKvqelpXdt6Fp5EG0oeozMDEmcKvnQ00/P9R1+e/pCuiSZ6R8UWdt57/3iU731L0c13DXyVO8ksRaSIjPiiHUi0UMCghWB75/7miyRA48j9lfZmgLKnZVpgVEv7eld/JVd58d7vZEr672XgPoje1VbwbkN5lua6bKF8HVzbXxG/dUfkYC6KlFefhwa238prTapZDGjmo3SyK81JFudKz4ZWt9/Py91aGxvdq98Dg2rXrm30H/aKmLvzZb1Vq0cs4xQWD/GOr1FE+JMfXNteseDbVcFpZxgYb2mTM8eC7la7gPw0ZPKeS9iS+699iKfq/0WmPkyp3pRcNL6Zp+jyoUawLF+49eE380Ey8E06/Kno+sfKAyeviWyHcgeM8rtzoMo3SWEaqAS4MI/LZnYtC4dOaGnlDLHqKwSx+oLN+6NeImbh0a2nUFvWrx1rDTtYZWPxZEgSwLvO2eygOjscgqAcKblKlqxQRTiz0dJwPoo7tZ3XZA1jO/geLIUZTUJ6qXzFjd3ZqLMc8F7VXvtJD/GUX9eWruBP4QBP6po3nhuNeqK2RhR/VvFDg7d03klfckIFZvaJ7X/fGwhNy7EuvbBxlAf5Wo+ztor6q1Yr4j0DfkaJuLJ0XtB8ZPtPfcMnOD2waEn0GBhiUNnjf58eshuDoHKIFafOHI+vgXR7NyMgf1HtUlL+6o2adPe+92ewyPqqBXnyxy2REru27J9Fho4fLqC9XgBzvTBZeGWn8WNmoBBTZs7dt3bthBNLdt5aMnVX96527YTbnMVjFqoGItQHWjqFQ3z4s/O8ImuvHqJ1TxlRGez9NyK/CcZ1F757zMrj53TWQAXYLHQu5rNxC6sHVevCWtclMB9NnLAHX7B4f2YQA9NMpUQQtWzD5BRN1KwBA/+rRAT2uuWx/2yvYQ68iidifgMhIYTzpFdUq4QmZeS926gl4Z6lbNlpdH3w3RL+VohbXb/+TPCOSyltPyN031oo7qg33FLwWoDPceYmlhCqjiT1B9R+u87rC38Biymm5CSjJa9jcM7AUfykeh/VBzfmt9l3txwQ8FxrxAaguSiL9GgBAnZar1RKYX+z7eDKCP7s9n/opZnzGQz0PEjK4kPBqomZf1tPfuGba9+vsQvHeU9efp2Rfo8UzwjjtrHsjoWGDhiqqFKm4/SSnJfhN5xSEE7jiiNn5xWIG7C1dUnhAgsp4B9N2ou1ThHVXHCuTWcLdlGtY9/hxUPnNE3Zk/ylXGkmHVNosHL1o25xjf2DUi4WYEHbIJikdMLDo37C00hrxujg9w28SaaHCPiMwKsyqqesWRdd3fDes7bU91a1gx5zUGwQoRCXHrpzAlxnBZqn8Lyrw3tp24rjdshcY1cw9C0l8LDXFhQ9iVHLvlPQ0/MrPl9LVPjYjA/Ua3V39DBFeN6HyelEuBXhG9ckbN2bdmY2zDAHrYAXRBUtS6oKhbnZrOx718uHNnwGdhOgenewwD6OlKpXfcRR1VM5Mq4e65rnhOFKdlc0/d9FrLo4YSWNg+62iFcXsIjyTl5h6LF2vOap637p6hrp/v/17TUVN6gPZ8RCGfzFEQ3RE9GRhzJZ7b8ttspt0eTt9c2D5zhq/eLyCjWn02nEvy2OEIKJ6yopcsqe2+L+y9tNKpRkNHzThje58NNf2p2/dS9G0tdd0udR4/FBjzAqkXIgl/baiZHqA26ckRPzu1uLfoWbCi8vUi3h+BUPeCHBMp3C99oDK6ZWvk4TAyHyjQIyJvbqnN7qTBK+46q2RT6Qs/A/DmMf9FUnAAeuszUn55Z21nXyar/s7lVZMTniyFYk4mr8Oyhy8ggpYZNfFFYQWbGttnV4vq/WEH0EX04uba7qLIcnHJmuMP2tEf+6EITs9FmlhV7RfFF3pKozf/+qS124Z/1xTnGTUdNZGpQc9XxciHstkvqri+tS7+qVw8Y+ayJ93vQr+Re8NeRKAerjrylPjNYX2n7cnIjd9e2uJ91UjqfhntBMhcdkURXluf2LEjOGLpuZnJGrhgRdUXReST2fyeKMJOykCT9K/RksiJd8y9/7mRFJ7KMHBq9beguHwk5/Oc3AkocJfX03Ph4nP+5LbFzviHAfSwA+huTynoalVsTaf3Ur+4IscqMD2d49M9hgH0dKXSO65xxawTIca9YA3vo3gekeD0llMf+H14hbKkbAgMzFQOOkVk3zCvJ2rPa65fvzTMMnNVVsOSo2Ky74T3i1uJLhiXi3rs3I99s8J+oaV2/c35+HCqLq3f8qqzEZE7oNw/NBf3yF6u+ZwGctmRa7t+melUcHuqw/nLqyaXGHk+ZJckIO9oqev6dcjlsjgKFKSAywYSKO4HcEh4DVBrIFWL6+IhZy4Kr4ZhlMQV6CNXXLiiqs6KLJchssEN4wrXtdTFPzuM40d9qJvkFdHe3ypwyqgLYwFZFNAnjC/1i0/PwgSfVBr32Q0q9idQiWaxkbzUUAJGFx9xavdFYQWbFnZUnwSLlWEH0KHyXy31XbcP1ZxC+ffG+44/SLzYjSo6Hxh19pGRNNutzLx1Xyn75HdrO7ePpIBiPGfhr6qn6nisQZjb+ewVSjapJue01m/4azF67q1NF609cf9kf3AfgDeF2Xa3fau/Kf7/2uYjCLPc3ZXVuHLWIQhkJSAhZq/KdK3HRPn/CErNUZlYge703n7PrKMjUe8uEZ02JjQLpJEiWFeCsrNvr+18aURV5gr0EbHl+iQF/u6JfdvimvV/yNa7fgbQww+g5/o+Sl2fAfRwu8E9FKpidZilCnSz+jiz5fTuB8Isl2VlXmDh8upjraBTJNygp4F9++K69T/PfAuyc4Wz7jqsZHxs8qeNwcdytk+UwL0ouNErww13zo6nNbEpOzqDV0mlQK16KyDfg4SXqjurbSiyiyn0JXjyiSNPif8wrBeLIyFqWDZ3muf5G0dy7p7OUcAXNfNb6tf9IsxyWRYFClVgMIC+DsDB4bVBbaA4ra2+uz28MvOvpAXLq94gIn+CIBZa7RRBwuDQn9fGnwytzDwsqHF51fUw8omwqqaK37XWx7O6JcxbO47bp9SW3gfRUNOwhmXCcnYr8IxCz2yt6/5DtnyatMk82nnPrbB6EfcOzZb60NdRkR8eubLrfWFNEm3smF0jVlcwgD60/aVLK8u3lnvfA2RBqL+fQ1964AhVayG/UZgPZH3rj3TrmOXjUnuh77fxBohcBd37NqchVM1d4UstK+OfQ5NbfDW2PheuqjzQ9yPLABwdZstV7TV286E3tM1vy3gA3dV7QXv1pQLclLN3XGHiFUtZgkdKUXbC7ZnKruMCrZ3VV4rF13Ly3V0s/RRyO1R1aU9P0DjizAMKmb+i+vPG4NqQq8biMiagm1RwSWtt928ydondFMwAOgPox2bzhgvxWv9oqYtnbcbfBZ3VtdYi1BehLkgjMGe21HXFQ3RhUVkQaFw283g1pkNEJoZ6ObGNLbXrW0MtM8eFpfZxjpR/DNBrcjjQ7FNFS8yf+ME7zrhvR45J/uPy7qHd7Pt4gxj8GABX6OSyg8StCMD7J05ILr1l5oZkLquyaFX1UYGPh0Kugw/Bwpba+E9DLpfFUaAgBRqWVU7zPK8LkAPDaoC6aaww72it6yqaCXG7s3GTCdXAbW8UXgAdCFT9GcW8Kstln2lcMWuxGNMY2j2n2NpaHw93TDpE5S5YfdIkm0wuz+GevmHxjY1yFAGMvOcZlP6ks7bTz2ajF6ycfaQE9i5ADs3mdXmtPQuI4P8118Y/4sKpYThd0DF7nlq9lwH09DQvWH3MpCBZfr1A35OjlejuO+CBQMxFbbXrHkuv1sV9lHvu8n10ZWH7uYeiSa/ujjNGlm640Huh4f45rzF9doUAoe4hLqqf8TdPvz5bAfS3/uK4fUomxH4sIucWep8UTf0Vv5vSv++JN599d3+m2pSaAFUWuRUG810u4Uxdh+WmL6BA69a+fd9198j7XRqXVX8UHm5I/6o8MmcCqlZhPmg3b/1RtrdqZQCdAXQG0NP4y1+4vPo0NXCphsL7KLaKp2c213S7lU/8FJDAglWzT5CkdkAwIcxqi8VFzfPiPwmzzHwoq2FZ5cSIF/mGAu8EEMlFnQSwVvTXyUA/+vN56/+Wizrs7ZpNunPvnY6qswD5drh78eZbS/O2Pm41gFvt/bGgZtov2iQ7s9f3puH2kwS0K2QxX4y+s7mmuznkclkcBQpSYCCAHnETGQ8IqwEKqFG8u7k+fltYZeZjOResmlNlfetSnoY38UvViuixzXXrw548lDeEbkXuI+13tYhIQ1iVUmh/a113aVjlpVNOKoCeSKyAyPHpHM9jciegwDYDve5p6f5mZy2yGjwfbLXMb5/5NoH5oUD2yZ0Er7xLQKFfa63rDi0LxgUds+apNQygD+MWu/jvNaW9f++9EdB3C6RkGKeGdqhCH4TIZa018Xi2UqCGVvkMFNTYUXU9rHwso9kyVK5tqe/6UliTVzLAkNEiG1bPneYlk+2AvD7UC6l+Ptg8/bpsBdBd3S+4b9ZxNmJ+BsFrs5C5IFSuIi1sbTB1W13b0Q8nMtm+BR0z3yjquWx+h2XyOiw7PQEV/KwMZReOJvNAY0f15VC497D85LOAYosYXOfvP+3mtqPbMvp3vjuGMR1Ab+yYfShU/57P98co6vbzlrr42/d2fmN7tUvfxgB6GsiLOmefFVi9K41Dh3PIdhGc1Vwbdy8f+SkggcaOqpmq0h72DGULvHtJXfzWAqJIu6oDL1r9L0P03aG+bE+7BqkDA1WstVFc1nZK/JF8e3BtaoJ5+ORZJxkYl879yOE1jUePSkDQBZWrmmu7ukXCWY0zqvoAcHvkqsiK0ZbzqvN9QN/VUte9OORyWRwFClLgghUnTrcSdAPhbaHhAuhQfKS1Pv7/ChIlzUovbJ9zqsK6FchhToyzBiju/eNdCsiOqjsEsihN6qEPU2xsqY9ndXXvxR01+/Rr73IFspo6fmgMHvEKAUWgBlfvf/Dm/7758McytiprKPVUiuQpG98LxdcBqRjqeP57ZgXCDqA3ts+qFzX3cQX68PrNPR9rIvE1BS6B5GRPdJc0529ivKv9TQcvzWbwcXhS2Tl6cFLlKgDTM3FFhfQakROaa9c9monyC6HMhpVVr/UC6QjdWPHFYPO0pqzewwq5oHNWvVXzPwAOKgT/oq6jYHWw/7Z5mQ6gu4UvD6+adZKx8hOoHFLUpgXQOBXcHUv0Ntxxxh9HnGm0cUXV+yHy3wXQ3DFbRQV6YXFNmVf2/dFMlhgN4JgOoLsH/z7t3QzAjAYxL89V+XZLfdcVe6sbA16atYgAACAASURBVOjp91yGAug7jMW5i+fF3QCSnwIScAF0aCqwFe4KdJX3Ntd3/bCAKIZV1YF0n/4NgL0QOZppn6qw6P+ZhL5n8enrV+fjbPvBPXndDMg3hxyYGFZ/jY2D1Q20fwtPr245df0T+dTmRR1V5wQqS0OuEwPoIYOyuMIWaLx79qEosd2ATAmrJS6ALqqfb6nv/nxYZeZjOYPZmdzk0jAD6Ao1J7XUr7s/H9scVp0aO2Z/BqrXhVWeS1/YWhcPLSV8OvW6ZM3c8T2J4B5AT0zneB6TE4EnRXDN0yhryXba9t219uKOmtI+9H5aLK7UkLN45US3kC+q+HpLffxjYTWhccWsEwVmddgBdBG5uLm2ywWnivZz7tLK8oqKyPcAuO/w8DK6DENMIZsg+LDdtLUt2ylRh1HNjB/qJrM/emr156H4TOgXUwTWyHXPofRL+fB9HHr70iywoWPOYZ61KyGhB5y/EtROuzbbWeRSk8P2e/xdAL4S5mTcNDl52CsFsrICfdclGztmLVA1NwkwlR2RQwHVlV6/nHfn2fGtI63FwuVV71Mj7neYn3wUUGy00Gu0bvqSbH/Hv5xjrwH0wZf4Ln3oa/LRcKg6ieDq5tr4jXs5Tha0V6+XIpw5by3qlgwRmC3kALpCvtFa1/XRoe6BsP59wYrq00Vwb1jlDZbDAHrIoNkqbkF71ZsEqZmzk0K9psp/tdR33R5qmXlW2KKu6gm2V6+wKp8UYFyOquf2+9sowNVPS9mv8vEhdmFH5X6q3kUAPrUzrft+OXIq3su6VeYW/1Tgs9aUtbXVdm7Pt8YuaK9+iwC/DLle3AM9ZFAWV9gCmVqBrqKfX1Jb3AF0t+etVb079AC61VNb5nWvLuw7a++1X7iq+ljr64MCGf3+iaoW4l3UUrcuq5lFGu6fU+b1219BcVox91VBts3dE8D/WugVuvnQtVldkTcEWMNDR8XMUxPeLZ66FMbhPkcVZGflptKiuKm5Lv7hsCYSX7Cy+nD18WjYAXQVubC1tuvO3Chl76rn33vi/iWx5LdgTUNG04fvpUkKfQlWvtnT63996bkberLX+vy6knvPY0RWq2J8qDVT/F+QNLPbzlz3QqjlFlhh7rvCBnCr/EPbOskRaCBfsi8e8rlc/N7VdNREpqLnTKPybc1Q9oIC6+YcVVe7tvS9UHP32dnJtnPp9yujWw/3TleY7wp0Wo4aPeYvq6rrvVjsjMUnr3lxpBiN7dWXAPjRSM/neRkTCCBYFwT6cdRP785l8Ny1cMiH9ob2uTMM/JadL3GPyxhJhgpOI4COBSuqGkTE7QXqZaga2S9W8X+lpuzYodIaFGQA3aWhE3zfPm+ubpu/rjdbuAs6T5wlNpXiM7yPYosanNlaGw97j9vw6siSdiuwYHnVGyCySiTc2YYien5zbXfYAbO868WGJUfFvP3GX7jzN8itznPprnKTBUR1C4y5akvvpOa7z747Z2kt99JBsrB9zikK+zUAxwAoy7vOLLwKuckTW6FYJsZcm88p9Ba1z6oPYFx65DA/fRb61iV13WFPCAuzjiyLAlkTSE0WtlgHwcFhXdStQFfg00vq4m41StF+3IpDGNMJDXXFnBo1sxfXrwt3zJ1vvaCQxvaqOERmjbZqqngB8E9rrd/wu9GWNZzzB1dducDWguGcx2MzKCBQdWNbNT8tUfvJH8/rdpn28u7j0p8+0ll1tli5EQK3D27xvIfJO+09VuhbLbXxq8IKoDfcM2dfEwv+LKFP+pW3tNR1/bpwWEde04Zl8yZ6ka03qaIxh3ui9wvMjX3Sd8Mvax98aeStKdwzU+mZV1R/0wguh4T33aSqX2qt7w5/ZXuBUS9YceLrIcFKCXmRngCf8p+f9rVcBNBTXaCQhSurZmuAmyDyJgCxAuuawq+u6LotvS/UZiuAvgusYVn1SREPNyvwxpAnFRd+n2ShBar4Q4lq/WjGvI3tVRcAUvST9bLQHaFcIpXND9gsgp8A/peaazc8H0rBoyxkyAC6K9/tFS6qP1Bg3iivl9XT0wmgX3TvsRXJaJl7kTw3q5XL4MUs8KkltfHrh3ogKrwAuvSL4mP79U+65eYsB7saVs+d5iX9PwMoDa3rBM8Evjm97bR1fwqtTBaUFYHU6mBE7ofi8BAvGAAyt6WuKx5imXlblHvxag7c+EaTlC8rcHbOKqrqUv38d6kpbxpq0lFO6qiQhnvnTIpE7VsU+GIG0p3lpFk5u6jqSoV82Zqy+/Nx1fnLXRraZ87w4IW9R95meOa0llPX/T5nfcALUyCPBAazfXQA4l56hPIZ2ANdF7TWd7eFUmCeFrJgddUbkJQHJdzJXYFG9ajWk7v/kqfNDq1aCzqq3icq3xlt8FCBrt5Y5PRfn7R2W2iVS7Og+R2zPmPUhJaKPs3L8rA9C9wXEflK/yaJZ3Oi+Yg6xO0du2bWawPf+zCsvl8k1K0gRlSlMXWS6ldb6rs/GVabByZHj1sKyOlhlTlQjsweK8/GrrVui8l+9F1u1V6bqyA6gCSgvxArVzbPiz8bbn8WRmmpjACRYC0Eh4VSY8GLQYCT2ubFHw6lvAIu5G0d1QdHFcsFmBFmM6zFRUvq43cO9Q48zGv+R1lucuSy4w+EF71URD6hYb47zmjFi6VwXRk8v/30bG9DoW7yxKpZB6tvPgIjl4lqSbGIFkQ7RNabSGRUK9AXLp91phrjsqrxk3MBdZlC70JUv1xqK36XT+/o0wqgO793xqsm92/HbSJybs4906xAOgF0V9T89tlzjeqSIghMBALcWyJlb0/nJiuwAPpWsfp5/4XpN+ViVuHFHcft06slHSFnYng0EFvbVrv+mTRvaR6WJwKpVTf7Pv4zGLwlxCo95fWh+s6z40+GWGbeF7XoruoJfkzeI0bfC+CInFRYNWFFfhDpiV67+JyRp/7JaN0VcuHqkw8IkokrVaQB0ENH+9I9o/XNp8IVfSLarRY/9hLSNpr9kbLZrIYlR43z9hv3R0BeG9Z11eDhhK+n/CJPV6WF1U6WQ4F0BdyevL2292cioU7k6g0iieltp/x+U7r1KMTj3rm8anI/5PdicEho9Vd9fsLEYPotM4s/faybvBHYyGIjo0qB/gw8c3auJkUtXF41R42szNW+vaHdd4VdUJ+odqt6P/YStmDGOLvIL32gMvrS1shpBvohQE5E7rZ3Kuy7YHi1V2v1k0vmdbsMV6F95rdXv92oLoZIKCsvVdFjo7FD205ZXdS/pa/ugNQk8yl/bxD1PoeBIGPa72xD60zAbQNxt/Hx4cWnxR/LaVAyxEalW1STNplH2+/+KgRXp3vOno5TwN+5W0tT68qur6Ap5TqmP+etmTu+rN//jQhOCQ1CtQdi6vJlsk2DNnhYsbHKE/kIBPXcriS0nt5rQar45bOmrCFX2zOedddhJRNK93uzQK8AdA4gDKRnoesFuCuS7J1/xxl/3DHSyy1aNucY39guEZSPtAyeN1oB3eEmhaviR/v0BL+6JQ+3khnWYOytHcftU6qlnwX0qhwN5IbVI+kG0F2hi5ZVHhN4kR/sHChWD+si+XSw4us7JvqfW5rmS6fCCaDrJhXvkiNrzrirSZpyMuhsatqZaq6m6gYJcBVEQkk3LaJ3jh8f/NctMzck8+k2Yl3SE1i0svp039dfi4QwMErtXyk/Cp6fdlkuJoik1+LMHZV6UbDvxteLyEUQvGvn/jPhvYxPv9pJVb3HQ+SKxfX3b0z/tOwe2dRRE3nI9Bzi+fJ2CFwa/KOZKmr3fSCKrRZYboze4fd7q9rOWPdiIb0AcikEH22v+iyAa0P63XGzOb/7jFd2Va4eLLP718KrUSANAYUsaK/6oIjcFNazjQDdzbXxOZDiflGaSnPaXvVlI/KJNKSHPsSNhYxc2VIbd6uyx8THbQkkxtw28KJtmEES1c2q8sHW+nhrrn7bGjpqxnm2163SO3ZMdFg+NVLwPCyWBaJ3IuGtK7QxzisoFXLW3dXjJ5XiJBVcZC1OF8G++cRdVHVRBDCyqKW2qzXMdrl9gA/Q3mUAakIoVwG5Oag95CO53vMyhLYMu4jUu6f6kw5DInGVMXJh6Ptxp1GjVApV0QdNQt67+Iz4hjROKapDLlg963WaNO2j39Na2wMpf0u+Zz7LWucNjLs/tTM9/nWyc2ZBGNdV6IMVsegpt+YgE8/e6n/u0sryijKvEp65AKrnYCBtfShtDsOt6MrYmVnliNXdn2rK8USVwexmp0Pl3YCeFNaksqLrr3Aa5FJ9XzdjVfzzo+n3hmWVEz0TaYfghHCqxVLSFFAoNqngHmttc4VX0XV7TeeWXD3XDlXnYX95u5Ua/bb3eit481CF5/rfRfT6ltruH6Vbj4b755SZ3mCRiLgAzgQIylw6jnTPz/ZxBghUsUMFfzewn2uuW//QcOrQ2DF7qarmZsVnuhVVJAC5urW+K+fpNBYsq54tnrrUZPulW/09HafAFpPUtzWf0d0+2rJ4fm4ELr7t0NK+6VN/ioH046P9nvhH4Gld26ndf89Na/Lkqi7t1apZByPw3q9qzxMRF0ifEIJvWg1M7Vur2hGJyPvf0BH/62gGYWldcJQHXbJm7vieZHC2WvsBCNyK9Kk5TPc3ytaM/vTBvXJeAvSfamUVovLtiRXJxwp5ktLCjjlHqNqlQCgpBDeq1dNb5xV/auTR300sYSwJXLjq5AOTyf77RMRNSBrt73mfqn6stb7722PB0D07eb12TRgvHATY8FI56u6eHXdbq4yZz4WrKg8MAs/te1uzM2A0GcBQE3X7FPqYqvnUUXVdv23K8USNC9qrz1agWQfGa/xkRkABuJU1mxV42ih+FbGRxU9Eok8V24Q4N6kWE558rYnad4vReqgcBKSC6WWZoR2LpcomtfakTIwHF3bMOlPV/BCA67cR/56qyuOeBrWL563/21jsoV1trvx+ZfR1b4hUi8VnjeBNgO4HhLOYI11XVX3cir5zk1SsLbbvmyEMZFHHnDf7am8TYETv/xS6ScTMb6nt6kzXeywc17Dq5Cme398OhDLu7hWDy5tr4rflq10qo8GyXx0gsejbYfEOde+4FG68Ny6NMV++Nivf6rVdYM5prlvnsiLlxadhyZwyb/9gpgTyASs4fuBdnU7I9nd4XmBkrhIbg8A/pe20DY+P9hKNndUXweK/AVSMtiyev0cBFzDvUajb3/xJq/i12mgzXlz7z7b5CPLdbUSDWrfHUTBl/P753jh/a2TLr98y/P3gzrrrrJJx5qUKRFEWGDsio2zYlPf7gdrIjsT2aTtGsmp14fLqqf0eotmo60ivoQkv8Ysz7n9upOeHeV7qB3Cy/QKAD++cMemNtGxVJATy5QmPJb98y/u4+nykjvlw3sJV1cdqEnfA4BiMbLKNi/k9L5DL/Oen/XIkf8f54BB2HVJprzr/McWDd4RYzLOwDTtX6B8CaGlYM5X3VGcFkgL9AwSXtdR2PxB220IvTyHn/qaybGJF6SG+SR6FQM6H6FmqmCgief39Ho6FWih6ofKIGGk2QNyW62NP95ZtLoaXPO5FskzaOE88fE8g00f+MlI3wcoHWubF3aQffihAgVcJNC6beTw8cwsUJ4w444OqC3B9vWd79MaRPH8Uaqc0ts+uVsWdIvr6kbVBFSqPmai5cPHJ69bn66zzkbUtvbPcRIRYQt7o2+AsUZ0/MOZBDArjIqcQ+ALtUcEaCeydkZiu/cnJG57JB6uGh46Kec9N+JSqXiOuzvyEIZCEIqGCbSLYILAd1po/eRH8fbsXeeaE5Wt35Pskz9EiuBW4D5563ISKoOSgpNjXGyMnQDEPwJGAS7GZShM+4ufx0davYM9PZa7AxfaF6Xdn4rnTjVtLJj/xJh96PaB1EBl+H6nuEGMun1HTdUeuJwjlST/Leb+aO658AmbAJs5U8d4laqdleVXjU2LxiRlr4ouL/bvn5X0+kE7/8Q+Kxdcgw/t9U8U/xciFwaZDVmfiby1P7s2RVcPtGb161imaND9Q6GEiI1uJvnPc0Q/BTb2x6Bd/nWerz3cH437X/jrn2LIdXsnUSMRMNz6OgqAGolWAuIli7nfNvb/J2xjEyDo842f1APKZYOrW77Qd/XAi41cb3gXkrLsOi5WVTZ4SE32tQI5JZdkBZqcyiwhiAkSGVySPHhDQZ+GbS1tO61oaxvOQyxhRXhG5QYD3cXwZ0j2mCBToh8gOgf2jQFbAmt9bBH+rKI0+Pa3Anmf4xRzSfcFixoaAe8EV6Q8+opB3Q/WgwYf3of6O3LsvN5umX4CNAeSHKqU/YBqn4rhn3rniTa9JaOnlavRcAzlQ3Yw1VYN/Pwi4WVZQURWIuxd8AFtV8JyxWBmJyPfvOLXrUTcCKA6RcFuhqnLeb2aWVYzzjlKVEwR6HCDu5dkUQMZDtQICt7+Qe0Hzr79F9zZ+0Ds1uhKB7yavuL9DAH0AegWyw0KfN6pPWZGnAPwTwLMCeUYk+dfm2g3Ph9uazJfW0ADPX1i1T3Qfb6YHW21VZ4rI6wBMVmCiG6ArNJLpiQiht9QlHxf4UPRBsBmKZ+AmOhgT9wLtTryw7bG2+Q+77TCK7+9IIQ3LKw/xvMi7VHGGERzoJkhAUAqBUVUjbgLPK14+qAXEeTyvgi4b6LeOXnN2vKkpN9ughH4/sEAKZEDgorUn7p/oD94qA+lnX6dAiQz8rrgxnMuI1C+iSVjErMBz36cC9Ftgk4j8L5L2N82ndW8QKcLvoSG83Sp+P5n4CIDzIKmVhyWAertdZTHwfR7IwG/xU6pYipLIN1tOWut+h8f0x415rrz78Nimsv0OlMAcqEbLjbHWJs1LsQnB470bp7+Ujy/iXTr/P3dUn28Fl4vF0ZDUanTP5WB2f0Duh1kAqxgYC+vA1kUJSe0NmxqfBVANRMRt1WVdQqDd3QgKMQN/k2oGi3ar9d3/iUK91NhGkRqDq1rjXsoP/g0PvGoTPAPFC+7/7Y7VVDmpAl2ljKucqJjB0eRA4E9gBifJDmYGcPd16h/SCAzqwGoKlWBwSwf3/3f/554FktDUfn89ImYrxNXLPh8E9mlPzT+jEbvR9nqPD06Udy7FN74Zxl+7ywpY21njTQt6Jia86GtVg2kCHKyqB4ro/juDKJPUyj4ug+Dgy2g3+cSNjwyMGLVqZKAvU/cLZKDvBz7udtJd/TyMWoV0aGoM9/L6pB5nBu7t1P3s6pa6L1P37OAY3r1wH7hXNfW/uxt61/DePXCmCnF/LIP33BYBHkLU3NB80rp7w3jZvLfWNzxUM06e65ln1JzjJqYp7GQRGT/w2+AmBrn6uke1gfRfxkhCFZsheCAAbjq6Jn4/g+e7EVbIJWvnjuvtT85SY06E6iwglYVsEgTjoCgd7HYZeBQeLCP17TH4SsLdKanv49TvcAKChFpNpr6TxX0vwT0/+O47WVO/1eK+r9xnC9R8uaV+3bqx9H3ktk97xPZdLKKfATAtjeBmEtC/WCNXLKmJd4T0LVF8xQxmHpSkLFRPaqA4CIrYwPcc3Co2dy+mfjsHv8+81Pe5ex/g3qVB/+RZ+cW4ffw1hZxtzq1Of2jdvSV2uz04GrHTrJWDPSMHWcVUBSYLdB8RcSvVywYWcYnn3jWKiLHqxj2DvxVuzD3wG7Drt21wrJT6JXDfBEO9s37Ft0XIN9zA7+7g4Olfv2MD/em+mQbHduKpwv1W/+vYgVP0Vd9nqdGlW0ThMlb9FaLffkbKlxTE4gn3XqetwWDKpjL4215rxJsOIwd5wNRAsK+o+520Zer2T1eNuX3UJTV5R2JQG3X9rzIwtkm9cXZebgTjzCy8Abld49RU1796bPOy+yLkXt5rca+6B1P36r8zbu0aw6faMjAmT43pB1/opu7pgYVOcOPmTVCsNGJ/MGPV+kfCnNR10b3HViRLyi+A1Xe6DJCq7nlGU66D75Y1Ne3b/Va67yg3pk9tizP431c8w8juV1S7lrt4Qar5u/rqFc81u8aAr/ibGRzTuT+HXc8n2+AsBmzcg8+/x7W7+n2ghIHjB/7eBp+TUv+LSf1tDWS1edX3Q6o+bqzwsmcPCdxz3GAmbPccF+z8XXTPcO472T3T9yiwDaovGiPPW6tPQcw/xZO/B73YiDMO3tImbQX9PDPUl2g2/6J4LQoUhMClD1RGX9wWm+5pUJUK6Im8FrCTASlT91XqvtjF9kJNrxi7xUI2yUDqqz+ZqP9w8NRhz+bjy6+CwM/TSro93yYlXtg3Fi11AfQDPMW+qigXz4hat2rI9sN4vX5gd8Qi9qWE1a0ojW6a9KfENmYhGEanKuTSDZWRFxOxiL9Nxsci/eNtJFYeU780YVPeFSaQiHvcl6gaG6jvecalOu1TX/tsNNJnTNBvEe3Dtp5elE3srQD82PhtwYt/e51tm1/YP+ivlmxYAq9iSk10h7dlfCQonay+HgATHC7iHQbo6xU43AWJBtOHDaMjsnOoAtuh+KsxeMSq/QsEf5GE9xhs37PliYrNkdf09d1SucG9fB8TL5VTaU2nbCqL2i2Tk7Z0gudpubHBeB8yzougTK2UqQvoiXthL1usBk+rZ57Cs94zbfPX9Wan13gVChS4gHux8fBR0b7esoi3LYiVR8ZJqai/6cW+YPK48e6hDzs29QsOHmznk8D2cZvsuO3H+2N9bJcaH2+J7S/wX+8ZOVIDmQ6D/aAYJ6LupZgPY7a6zDvW2sc9ax/xSvDXJ4PxmwripVeB39oZr75CFt1dPd6W2kMUcqhaeY0YqXChcs+zCWvNdhHd5ltsjXnYprB9AdSHiQQ+1HejtpjvDuzXWCSS+lt7+SdIWAlMicSMFbVJLxUm6vU83wtMxETFDwITcdFvzzMSWGONigk8d/S/3ncEJd7TwEAAfdLmhLw4rjf1sml8dJJs6+0bePEUxFIvmiIlCeN7VrAtMvDiySY8lJfDi/jG1cWLRIdKtY/AT1ovZhT9sSBIJmyk3Pp+f8zGrPW3Br7vvlPGH1ymzyQ26qQ1ZfripOIbi2b0vht8ET3pdX8zTzy3xUwtO1h2lPQL+ku8WFmv2dHbb6L9ZeblfelFYybwXP/5/+q/dPszU21xGQ+NH/yrPgP3bsTYUpv6L6zvuf8tIhHR/sCLuDvdCzx3d3lqS6xnSoz7byClntFYYFEGl2XQTUUR6REjT1ujGxGUPd5W07kjm+Nm97vw3LagwgTRfT0PEyIw46wLCAWmQqBRa9SIj20SwzO+m+DCMWvat5l7LqiYsilqg56KfonsY01yvFhM8OBNhARlsCYmnqRWNgaBe9Hvuxf9vVCv1wh6xENvv0USnp/QiEnANwmvx/rwIgn3HWW83qBvfIXFk/+u0tFTSpJNtZ27Aupp17XQD3RBzkfalx4HeJeJSAOAiXto0xOq9rvW4Pa2leufQ473Yi4EdzcB7x+dNbHEDr9MS5Oxft83KiVBWblJuvH3tuSL2h+dJCW9fSZaWmYkmgwS1iSP7i/ee9GZoLPGPLTpOXPA8dNl25O9snn7NlPqxix+meccIv0Jsy2IeBE/adzvGkoSnvst8fyBscnLf1f8SMREkv8eC+32vigBbNKKGzyFfd9Y9xsX2IHfuFgMLx+vWV9F3C+c+6/xjPGssQlTAuPF1GgMSY3BmBjUj4l4UWMAd4QHed4G9p+m3Dxx+D3x7WEGUcNu/5DlpcYyMJum1Mgbxm+TF8t6ZftGN8cUcOMa9183tpkUS8iO5yKRHWUDYxs/4n70A4nESkzfSwP3wcvHN7sb27x6vDFk3UI6wP0cmeDf4xxXrHF3rO/uyxhSY3k3hnfjdrPrnoi4aSLGExX3ftdaJFXMjgSweco+wdZMTZzZlQGpxJQeKGr3gzWToNYF0RPWSo9osjdqJNEfQdIg6sPYwPq+dc8zbty/6xkm8CMWPf8J6PooVmFlV/+4/5pIYNxzjedHTJBUeeXfjPv19lJ/P7uM3JSK/oTd7nk7Utma+7dOkpLywecYl8aitMwkd7g5coPPM71WoAPPM17UN0F/qXgTBsfB1nvFhOBd94iNeNb9ae5qgWtbEIlYlwnb/ReeH5Qg8HdgHx8l/UFFf4k+2/ukHrL/RPti5etsG9rchPnQv09CuiVHVAwD6CNi40kUGBQYDOjhaUR3vYQpTUS0b0uFdS9Ue8sODvhSkHcLBSiQbwLuhdYTz0XKxpXZ8qia1wSCwxHgDWLwGhWZpFb3Me7lgKT2AHL7TrrVhDGBRG1qdri6sWxkcOboHscSAyvOUqut3AguqW5Vg1vpoJowIr06sKfnVlW8JIIXVPVp8eTP6nuP2v7kE9vF9BzyhN/LiSb5dgexPhSgAAWGFti1atSt6Z+CKVqMD9NDK/AIClCAAhSgAAUKXcAtmjhQd8wQlXMCkaOhOj61Cs9lNhHtFAnum7FywwsFHcwr9E5i/SlAAQpQgAIZEGAAPQOoLJICFKAABShQiAJuxmW86rDogROmxl7sS8YinkaRkDIDWyExU4rApca0pb4xMbGIiiBqjJS4FWYuzVQqzZIxbq9WX9VYG9h+VUlGopIIAtsf9dCbWnUWSI8Vb0fEJPp9H0lUxBJ48uDEWF/BWYj3DOtMAQpQgAIUoAAFKEABClBgrAi41O4PlfRH3aq7Q1d2Jhg0Hys9z3ZSgAIUoMBYFGAAfSz2OttMAQpQgAIUoAAFKEABClCAAhSgAAUoQAEKUIACFKAABShAAQpQgAL/IcAAOm8KClCAAhSgAAUoQAEKUIACFKAABShAAQpQgAIUoAAFKEABClCAAhSgAAAG0HkbUIACFKAABShAAQpQgAIUoAAFKEABClCAAhSgAAUoQAEKUIACFKAABXIdQG/smH0oAr2WPVGkAgZBRMzXflK77rEibSGbRQEKUIACFKAABShAAQpQgAIUoAAFKEABClCAAhSgAAUoQAEKyxjOpQAADCNJREFUFJFAzlegNy6vvhwG1wMYV0SuY74pCviieuOEicG1t8zckBzzIASgAAUoQAEKUIACFKAABShAAQpQgAIUoAAFKEABClCAAhSgAAXyXiDnAfRLH6iMbt0SvRiit+S9FiuYrkCPin5oa+8Ld9x99mP96Z7E4yhAAQpQgAIUoAAFKEABClCAAhSgAAUoQAEKUIACFKAABShAAQrkUiDnAfRdjW/oqH6Hp/gegMm5BOG1RykgsgmQa45Yue62pibYUZbG0ylAAQpQgAIUoAAFKEABClCAAhSgAAUoQAEKUIACFKAABShAAQpkTSBvAuhN2mQe7bznFKj+HMCkrAnwQqEJqOARa83FbXXr1kOgoRXMgihAAQpQgAIUoAAFKEABClCAAhSgAAUoQAEKUIACFKAABShAAQpkQSBvAui72nph+9wZviabIXJ8FtrPS4Qj4AOIG/UWLa6/f2M4RbIUClCAAhSgAAUoQAEKUIACFKAABShAAQpQgAIUoAAFKEABClCAAtkVyLsAumt+48pZhyAwXwWwMLscvNpIBERxvanAV+6cHd86kvN5DgUoQAEKUIACFKAABShAAQpQgAIUoAAFKEABClCAAhSgAAUoQIF8EMjLALqDubijprRXe74ElfeLoDwfsFiHVwqo6uMCXNVS3/0L2lCAAhSgAAUoQAEKUIACFKAABShAAQpQgAIUoAAFKEABClCAAhQodIG8DaA72EsfqIzu2OadEUC+C8UhhY5dTPVX1aXGeB9vrln3Z+53Xkw9y7ZQgAIUoAAFKEABClCAAhSgAAUoQAEKUIACFKAABShAAQpQYOwK5HUAfVe3LFg2+0jx9DYAJwCIjt3uyouWPy+w3x0/wX7xlpkbknlRI1aCAhSgAAUoQAEKUIACFKAABShAAQpQgAIUoAAFKEABClCAAhSgQAgCBRFAd+1sWFY50TPRKyB6XQjtZhEjERCsCRRX4flpD7bNbwtGUgTPoQAFKEABClCAAhSgAAUoQAEKUIACFKAABShAAQpQgAIUoAAFKJCvAgUTQN8FOH/FiacYsd8B9I35ilp09RLZpIG9feI+QdMtMzf0FF372CAKUIACFKAABShAAQpQgAIUoAAFKEABClCAAhSgAAUoQAEKUIACAAougO7q3LBizkHG2A+K4qNM6Z7Z+1iA5Sp6zREru3/X1ASb2auxdApQgAIUoAAFKEABClCAAhSgAAUoQAEKUIACFKAABShAAQpQgAK5EyjEAHpKq6kJ5pFTZr1VxHwRFjMgMLljLLIrCxSKxxX6nWel/JudtZ1+kbWQzaEABShAAQpQgAIUoAAFKEABClCAAhSgAAUoQAEKUIACFKAABSjwHwIFG0Df1ZK33VN5YCwWuQLANezfcAQE+L5V74bWuvv/BhdM54cCFKAABShAAQpQgAIUoAAFKEABClCAAhSgAAUoQAEKUIACFKDAGBAo+AD6rj5asKx6tnhyI6CzAa5GH/a9q7Aq6Fa1n1hSv37VsM/nCRSgAAUoQAEKUIACFKAABShAAQpQgAIUoAAFKEABClCAAhSgAAUKXKBoAuiuHxrumbOvV6LnQfUrAA4o8L7JZvX/IaqfkljsnsUnr3kxmxfmtShAAQpQgAIUoAAFKEABClCAAhSgAAUoQAEKUIACFKAABShAAQrki0BRBdB3oTasrpzmJbwbVWSeAPvkC3b+1UOeUtifGQm+0Fy74fn8qx9rRAEKUIACFKAABShAAQpQgAIUoAAFKEABClCAAhSgAAUoQAEKUCB7AkUZQHd8lQ9URg/fEpslYj+twNnZIy2AKwl6VeRmo3Jbc826P3Of8wLoM1aRAhSgAAUoQAEKUIACFKAABShAAQpQgAIUoAAFKEABClCAAhTIuEDRBtB3yTVogxfp3Hihqrka0BkAohlXzd8LvAjFA4GxH2+rXf9g/laTNaMABShAAQpQgAIUoAAFKEABClCAAhSgAAUoQAEKUIACFKAABSiQfYGiD6DvIl3YUbmfauQ8KL4KwX7Zp87hFRWBiN4pEe87yWe2PNg2/+FEDmvDS1OAAhSgAAUoQAEKUIACFKAABShAAQpQgAIUoAAFKEABClCAAhTIS4ExE0DfpX/Jmrnje5LJT0NlPoDpAExe9kw4lXoR0N9bmM8uqe26n6naw0FlKRSgAAUoQAEKUIACFKAABShAAQpQgAIUoAAFKEABClCAAhSgQHEKjLkAuuvGpiaYR0+dPU1t0Cgw10JQXlzdK/0Cvd0Y70eJ5176A1ecF1fvsjUUoAAFKEABClCAAhSgAAUoQAEKUIACFKAABShAAQpQgAIUoEBmBMZkAP3llA33z9k30m+vVeAt0IJfkf5PCNaJ2qbmuvUPZeaWYakUoAAFKEABClCAAhSgAAUoQAEKUIACFKAABShAAQpQgAIUoAAFilNgzAfQXbc2KcyjnbOnQXGeqH5WBZMLqrsV/4TojWrx2yPrux9rEtiCqj8rSwEKUIACFKAABShAAQpQgAIUoAAFKEABClCAAhSgAAUoQAEKUCAPBBhAf1UnXLr03PKtFc9eBWAhgMMBKcmDftpNFbQfkIcs5CflUnrb7bWdL+VnPVkrClCAAhSgAAUoQAEKUIACFKAABShAAQpQgAIUoAAFKEABClCAAoUhwAD6HvppYUflflYjc0XggumnQpE3VgK9Uy2+b/pi/7v4nDUvFsatxlpSgAIUoAAFKEABClCAAhSgAAUoQAEKUIACFKAABShAAQpQgAIUyG+BvAkK5ytTUxPMwydVnyoGnxDFcRBMzXpdFQkY/BWKZabE+8biufdvzHodeEEKUIACFKAABShAAQpQgAIUoAAFKEABClCAAhSgAAUoQAEKUIACRS7AAHqaHVzTUROZ6vcdLlF7JqxcCeDQNE8dzWEJVf0VFD82kPWL6+PPiUBHUyDPpQAFKEABClCAAhSgAAUoQAEKUIACFKAABShAAQpQgAIUoAAFKECB3QswgD6CO+PSpZXlW8sjbxfoe1TkKAD7jaCYPZ3SB+hjgFkWiHy3rXbdYyGWzaIoQAEKUIACFKAABShAAQpQgAIUoAAFKEABClCAAhSgAAUoQAEKUGAPAgygj+LWuPSByvIdO2Kv9337Zoh+SCAHjKK4XlV836ouKRuPv/y4unvzKMriqRSgAAUoQAEKUIACFKAABShAAQpQgAIUoAAFKEABClCAAhSgAAUoMEwBBtCHCbanwxu0wfM6Hz9fVd4v0BkADk6j6CcB/auIua0Ez7TeXvuPvjTO4SEUoAAFKEABClCAAhSgAAUoQAEKUIACFKAABShAAQpQgAIUoAAFKJABAQbQQ0Z1e6VP6e8/NBINZqng3YDUv/wSAlir+LUY3KnW+/3Ex/ofv+V9G5IhV4PFUYACFKAABShAAQpQgAIUoAAFKEABClCAAhSgAAUoQAEKUIACFKDAMAUYQB8m2HAPX7DixNcbE7xHFSeL4qd+f+LOtrN/v2m45fB4ClCAAhSgAAUoQAEKUIACFKAABShAAQpQgAIUoAAFKEABClCAAhTIrAAD6Jn1/VfpDUuOirXNfziRpcvxMhSgAAUoQAEKUIACFKAABShAAQpQgAIUoAAFKEABClCAAhSgAAUoMEwBBtCHCcbDKUABClCAAhSgAAUoQAEKUIACFKAABShAAQpQgAIUoAAFKEABClCgOAUYQC/OfmWrKEABClCAAhSgAAUoQAEKUIACFKAABShAAQpQgAIUoAAFKEABClBgmAIMoA8TjIdTgAIUoAAFKEABClCAAhSgAAUoQAEKUIACFKAABShAAQpQgAIUoEBxCjCAXpz9ylZRgAIUoAAFKEABClCAAhSgAAUoQAEKUIACFKAABShAAQpQgAIUoMAwBRhAHyYYD6cABShAAQpQgAIUoAAFKEABClCAAhSgAAUoQAEKUIACFKAABShAgeIUYAC9OPuVraIABShAAQpQgAIUoAAFKEABClCAAhSgAAUoQAEKUIACFKAABShAgWEKMIA+TDAeTgEKUIACFKAABShAAQpQgAIUoAAFKEABClCAAhSgAAUoQAEKUIACxSnAAHpx9itbRQEKUIACFKAABShAAQpQgAIUoAAFKEABClCAAhSgAAUoQAEKUIACwxT4/x/OqRHTijkQAAAAAElFTkSuQmCC' alt='Powered by'>
</div>
</body>
</html>
"@
# Combine all content
$htmlContent = "<html><head><style>table { width: 100%; border-collapse: collapse; } th, td { border: 1px solid black; padding: 8px; text-align: left; } th { background-color: #4CAF50; color: white; } img { max-width: 100px; }</style></head><body>" + $summary + $databaseChangesContent + $logEntriesContent + "</body></html>"
# Send email
$smtpClient = New-Object System.Net.Mail.SmtpClient($SmtpServer)
$smtpClient.Credentials = New-Object System.Net.NetworkCredential($SmtpUsername, $SmtpPassword)
$smtpClient.EnableSsl = $true
$smtpClient.Port = 2525
$mailMessage = New-Object System.Net.Mail.MailMessage
$mailMessage.From = $From
$mailMessage.To.Add($To)
$mailMessage.Subject = $Subject
$mailMessage.Body = $htmlContent
$mailMessage.IsBodyHtml = $true
try {
$smtpClient.Send($mailMessage)
Log-Action -Action "Report Sent" -Details "Report successfully sent to $To." -Object "MAIL"
} catch {
Log-Action -Action "Report Sending Failed" -Details "Failed to send report: $_" -Object "MAIL"
}
}
function Is-PrivateIP($ipAddress) {
$privateRanges = @(
"10.0.0.0/8",
"172.16.0.0/12",
"192.168.0.0/16",
"169.254.0.0/16", # Link-local
"127.0.0.0/8" # Loopback
)
foreach ($range in $privateRanges) {
if (Test-Connection -ComputerName $ipAddress -Count 1 -Quiet) {
if ([IPAddress]::Parse($ipAddress).IsInSubnet($range)) {
return $true
}
}
}
return $false
}
function Add-FirewallRuleForAzureWorker {
# Get the public IP address of the current machine
$publicIp = (Invoke-RestMethod -Uri "http://ipinfo.io/ip").Trim()
# Check if the IP address is already present in the firewall rules
$existingRule = Get-AzSqlServerFirewallRule -ResourceGroupName $resourceGroupName -ServerName $serverName | Where-Object { $_.StartIpAddress -eq $publicIp -and $_.EndIpAddress -eq $publicIp }
if (-not $existingRule) {
# Add a firewall rule to allow the current public IP address to access the SQL server
$firewallRuleName = "TempFirewallRule-$($publicIp.Replace('.', '-'))"
New-AzSqlServerFirewallRule -ResourceGroupName $resourceGroupName -ServerName $serverName -FirewallRuleName $firewallRuleName -StartIpAddress $publicIp -EndIpAddress $publicIp
Write-Output "Firewall rule added for IP: $publicIp"
} else {
$firewallRuleName = $existingRule.FirewallRuleName
Write-Output "Firewall rule already exists for IP: $publicIp"
}
}
function Remove-FirewallRuleForAzureWorker {
$publicIp = (Invoke-RestMethod -Uri "http://ipinfo.io/ip").Trim()
$firewallRuleName = "TempFirewallRule-$($publicIp.Replace('.', '-'))"
Remove-AzSqlServerFirewallRule -ResourceGroupName $resourceGroupName -ServerName $serverName -FirewallRuleName $firewallRuleName
Write-Output "Firewall rule removed for IP: $publicIp"
}
# Function to execute a SQL query
function Invoke-SqlQuery {
param (
[string]$ConnectionString,
[string]$SqlQuery,
[string]$Object,
[int]$CommandTimeout = 30000 # Set a default timeout of 30000 seconds (500 minutes)
)
$connection = New-Object System.Data.SqlClient.SqlConnection
$connection.ConnectionString = $ConnectionString
try {
$connection.Open()
$command = $connection.CreateCommand()
$command.CommandText = $SqlQuery
$command.CommandTimeout = $CommandTimeout
$adapter = New-Object System.Data.SqlClient.SqlDataAdapter $command
$dataTable = New-Object System.Data.DataTable
$adapter.Fill($dataTable) | Out-Null
Log-Action -Object $Object -Action "SQL Query Execution" -Details "Successfully executed SQL query: $SqlQuery"
return $dataTable
}
catch {
Log-Action -Object $Object -Action "SQL Query Execution" -Details "Error executing SQL query: $_"
}
finally {
$connection.Close()
}
}
# Function to check and adjust storage in elastic pool
function Adjust-ElasticPoolStorage {
param (
[string]$ResourceGroupName,
[string]$ServerName,
[string]$ElasticPoolName,
[int]$SpaceRequired
)
Log-Action -Object "ElasticPool" -Action "Elastic Pool Adjustment" -Details "Adjusting storage for elastic pool: $ElasticPoolName"
# Get the elastic pool resource
try {
$elasticPool = Get-AzSqlElasticPool -ResourceGroupName $ResourceGroupName -ServerName $ServerName -ElasticPoolName $ElasticPoolName -ErrorAction Stop
} catch {
Log-Action -Object "ElasticPool" -Action "Elastic Pool Retrieval" -Details "Failed to retrieve elastic pool $ElasticPoolName $_"
return
}
# Calculate used space and available space in the elastic pool
$metric = Get-AzMetric -ResourceId $elasticPool.ResourceId -MetricName 'allocated_data_storage' -ErrorAction SilentlyContinue
if ($metric.Data.Count -gt 0) {
$usedSpaceObject = $metric.Data | Select-Object -Last 1
$usedSpaceBytes = $usedSpaceObject.Average
$usedSpaceGB = [math]::Floor($usedSpaceBytes / 1GB)
}
if ($usedSpaceGB -le 10) {
Log-Action -Object "ElasticPool" -Action "Metric Retrieval" -Details "Failed to retrieve allocated data storage metric for elastic pool $ElasticPoolName."
return
}
$availableSpaceGB = [math]::Floor($elasticPool.MaxSizeBytes / 1GB) - $usedSpaceGB
$currentgoal = $usedSpaceGB + $elasticpoolbuffer
$newgoal = $usedSpaceGB + $elasticpoolbuffer + $SpaceRequired
$global:elasticPoolTotalSize = $newgoal
$spacedifference = $newgoal - $currentgoal
Log-Action -Object "ElasticPool" -Action "Elastic Pool Usage" -Details "Current Usage in Elastic Pool: $usedSpaceGB GB (Available: $availableSpaceGB GB)"
if ($spacedifference -lt 10 -or $spacedifference -gt 10) {
$newSizeGB = $newgoal
Log-Action -Object "ElasticPool" -Action "Elastic Pool Adjustment" -Details "Adjusting storage for elastic pool $ElasticPoolName to $newSizeGB GB."
try {
Set-AzSqlElasticPool -ResourceGroupName $ResourceGroupName -ServerName $ServerName -ElasticPoolName $ElasticPoolName -StorageMB ($newSizeGB * 1024) -ErrorAction Stop
Log-Action -Object "ElasticPool" -Action "Elastic Pool Adjustment" -Details "Elastic pool $ElasticPoolName storage adjusted successfully to $newSizeGB GB."
Log-DatabaseChange -DatabaseName "ElasticPool" -ChangeType "Elastic Pool Adjustment" -ChangeAmountGB $spacedifference
$global:elasticPoolResizeCount++
$global:elasticPoolTotalChangeGB = $global:elasticPoolTotalChangeGB + $spacedifference
} catch {
Log-Action -Object "ElasticPool" -Action "Elastic Pool Adjustment" -Details "Failed to resize elastic pool $ElasticPoolName $_"
}
} else {
Log-Action -Object "ElasticPool" -Action "Elastic Pool Adjustment" -Details "Elastic pool $ElasticPoolName already in target range of $newgoal GB."
}
}
# Authenticate with Azure
try {
$AzureContext = Connect-AzAccount -Identity -ErrorAction Stop
Log-Action -Action "Authentication" -Details "Successfully authenticated to Azure." -Object "Authentication"
} catch {
Log-Action -Action "Authentication" -Details "Failed to authenticate to Azure: $_" -Object "Authentication"
exit
}
# Load System.Data.SqlClient
Add-Type -AssemblyName "System.Data"
# Get all SQL Databases
$databases = Get-AzSqlDatabase -ResourceGroupName $resourceGroupName -ServerName $serverName
$privateip = $false
# Resolve DNS to IPs
try {
$ipAddresses = [System.Net.Dns]::GetHostAddresses($serverFqdn)
} catch {
Write-Host "Failed to resolve $serverFqdn. Error: $_"
return
}
# Check if the resolved IPs are private or public
foreach ($ip in $ipAddresses) {
if (Is-PrivateIP $ip.IPAddressToString) {
$privateip = $true
}
}
if ($privateip -eq $false) {
#Allow traffic for public ip
Add-FirewallRuleForAzureWorker
start-sleep 60
}
Log-Action -Action "Process Start" -Details "Starting Database Maintenance for $($databases.Count) databases." -Object ""
foreach ($db in $databases) {
# Skip system databases like 'master'
if ($db.DatabaseName -in @("master", "model", "msdb", "tempdb")) {
continue
}
continue
$databaseName = $db.DatabaseName
Log-Action -Object $databaseName -Action "Database Check" -Details "Checking DBCC storage"
# SQL command to get space allocated in MB and space allocated unused in MB
$sqlCommand = @"
SELECT DB_NAME() as DatabaseName,
SUM(size/128.0) AS DatabaseDataSpaceAllocatedInMB,
SUM(size/128.0 - CAST(FILEPROPERTY(name, 'SpaceUsed') AS int)/128.0) AS DatabaseDataSpaceAllocatedUnusedInMB
FROM sys.database_files
GROUP BY type_desc
HAVING type_desc = 'ROWS';
"@
$connectionStringDb = "Server=$serverFqdn; Database=$databaseName; User ID=$userName; Password=$password; Encrypt=True; TrustServerCertificate=False;"
$databaseMetrics = Invoke-SqlQuery -ConnectionString $connectionStringDb -SqlQuery $sqlCommand -Object $databaseName
$allocated = [double]($databaseMetrics.DatabaseDataSpaceAllocatedInMB | Select-Object -First 1)
$unused = [double]($databaseMetrics.DatabaseDataSpaceAllocatedUnusedInMB | Select-Object -First 1)
$databaseMetrics.DatabaseDataSpaceAllocatedInMB
$databaseMetrics.DatabaseDataSpaceAllocatedUnusedInMB
write-output "DBCC allocated: $allocated"
write-output "DBCC unused: $unused"
$unusedPercentage = ($unused / $allocated) * 100
Log-Action -Action "Database DBCC Usage" -Details "unused percentage: $unusedPercentage%" -Object $databaseName
# If the unused space is more than 20% of the total space, and more than 1000MB, run the shrink command
if ($unusedPercentage -gt 20 -and $unused -ge 1000) {
Log-Action -Action "Database DBCC Usage" -Details "Shrinking DBCC database" -Object $databaseName
$shrinkCommand = "DBCC SHRINKDATABASE ([$databaseName], 20) WITH WAIT_AT_LOW_PRIORITY (ABORT_AFTER_WAIT = SELF);"
Invoke-SqlQuery -ConnectionString $connectionStringDb -SqlQuery $shrinkCommand -Object $databaseName
$shrinkCount++
Log-DatabaseChange -DatabaseName $databaseName -ChangeType "Shrink DBCC" -ChangeAmountGB $unused / 1024
} else {
Log-Action -Action "Database DBCC Usage" -Details "Shrink not needed" -Object $databaseName
}
}
#Remove Firewall Rule For Azure Worker
Remove-FirewallRuleForAzureWorker
#short sleep so metric can catch up with shrink actions
if ($shrinkCount -gt 0) {
Start-Sleep -Seconds 500
}
foreach ($db in $databases) {
# Skip system databases like 'master'
if ($db.DatabaseName -in @("master", "model", "msdb", "tempdb")) {
continue
}
$databaseName = $db.DatabaseName
Log-Action -Object $databaseName -Action "Database Check" -Details "Checking storage"
# Get the resource for the database
$db_resource = Get-AzResource -ResourceId $db.ResourceId
# Retrieve the latest 'storage' metric from Azure Monitor
try {
$db_metric_storage = $db_resource | Get-AzMetric -MetricName 'storage' -WarningAction SilentlyContinue
$db_UsedSpace = $db_metric_storage.Data.Maximum | Select-Object -Last 1
$usedSpaceGB = [math]::Floor($db_UsedSpace / 1GB) # Use Floor to ensure we get the integer value without rounding up
} catch {
Log-Action -Action "Metric Retrieval" -Details "Failed to retrieve metric information for database" -Object $databaseName
continue
}
$maxSizeGB = [math]::Floor($db.MaxSizeBytes / 1GB) # Use Floor to get an integer value
$availableSpaceGB = $maxSizeGB - $usedSpaceGB
$GoalSpaceGB = $usedSpaceGB + $targetFreeSpaceGB
$differenceGB = $GoalSpaceGB - $maxSizeGB
Log-Action -Action "Database Usage" -Details "Current Usage: $usedSpaceGB GB of $maxSizeGB GB (Available: $availableSpaceGB GB, Goal: $GoalSpaceGB, Difference: $differenceGB)" -Object $databaseName
# Ensure available space is between $minFreeSpaceGB and $targetFreeSpaceGB
if ($availableSpaceGB -ge $maxFreeSpaceGB -or $availableSpaceGB -le $minFreeSpaceGB) {
$newSizeGB = [int]$GoalSpaceGB
try {
if ($differenceGB -gt 2) {
Log-Action -Action "Database Resize" -Details "Requesting elastic space for $($differenceGB) increase" -Object $databaseName
Adjust-ElasticPoolStorage -ResourceGroupName $resourceGroupName -ServerName $serverName -ElasticPoolName $elasticPoolName -SpaceRequired $differenceGB
}
Log-Action -Action "Database Resize" -Details "Resizing database to $newSizeGB GB" -Object $databaseName
Set-AzSqlDatabase -ResourceGroupName $resourceGroupName -ServerName $serverName -DatabaseName $db.DatabaseName -MaxSizeBytes ($newSizeGB * 1GB) -ErrorAction Stop
Log-Action -Action "Database Resize" -Details "Database resized successfully to $newSizeGB GB." -Object $databaseName
$resizeCount++
Log-DatabaseChange -DatabaseName $databaseName -ChangeType "Increase" -ChangeAmountGB ($newSizeGB - $maxSizeGB)
} catch {
Log-Action -Action "Database Resize" -Details "Failed to resize database" -Object $databaseName
}
} else {
Log-Action -Action "Database Check" -Details "Database has sufficient space within the desired range." -Object $databaseName
}
}
#short sleep so metric can catch up with shrink actions
if ($resizeCount -gt 0 -or $shrinkCount -gt 0) {
Start-Sleep -Seconds 500
}
# Always adjust elastic pool storage at the end to ensure target free space is met
Adjust-ElasticPoolStorage -ResourceGroupName $resourceGroupName -ServerName $serverName -ElasticPoolName $elasticPoolName -SpaceRequired 0
Log-Action -Action "Process Completion" -Details "Database size check and adjustment completed." -Object ""
# Send report via SMTP
Send-Report -SmtpServer "" -From "" -To "" -Subject "SQL Server Storage Adjustment Report" -SmtpUsername "" -SmtpPassword ""
No responses yet